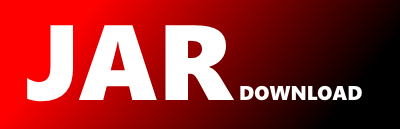
com.aliyun.ims20190815.models.SetSecurityPreferenceShrinkRequest Maven / Gradle / Ivy
Show all versions of ims20190815 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ims20190815.models;
import com.aliyun.tea.*;
public class SetSecurityPreferenceShrinkRequest extends TeaModel {
/**
* Specifies whether RAM users can change their passwords. Valid values:
*
* - true (default)
* - false
*
*
* example:
* true
*/
@NameInMap("AllowUserToChangePassword")
public Boolean allowUserToChangePassword;
@NameInMap("AllowUserToLoginWithPasskey")
public Boolean allowUserToLoginWithPasskey;
/**
* Specifies whether RAM users can manage their AccessKey pairs. Valid values:
*
* - true
* - false (default)
*
*
* example:
* false
*/
@NameInMap("AllowUserToManageAccessKeys")
public Boolean allowUserToManageAccessKeys;
/**
* Specifies whether RAM users can manage their MFA devices. Valid values:
*
* - true (default)
* - false
*
*
* example:
* true
*/
@NameInMap("AllowUserToManageMFADevices")
public Boolean allowUserToManageMFADevices;
/**
* Specifies whether RAM users can manage their personal DingTalk accounts, such as binding and unbinding of the accounts. Valid values:
*
* - true (default)
* - false
*
*
* example:
* true
*/
@NameInMap("AllowUserToManagePersonalDingTalk")
public Boolean allowUserToManagePersonalDingTalk;
/**
* Specifies whether RAM users can remember the MFA devices for seven days. Valid values:
*
* - true
* - false (default)
*
*
* example:
* false
*/
@NameInMap("EnableSaveMFATicket")
public Boolean enableSaveMFATicket;
/**
* The subnet mask that specifies the IP addresses from which you can log on to the Alibaba Cloud Management Console. This parameter takes effect on password-based logon and single sign-on (SSO). This parameter does not take effect on API calls that are authenticated by using AccessKey pairs.
*
* - If you specify a subnet mask, RAM users can use only the IP addresses in the subnet mask to log on to the Alibaba Cloud Management Console.
* - If you do not specify a subnet mask, RAM users can use all IP addresses to log on to the Alibaba Cloud Management Console.
*
* If you need to specify multiple subnet masks, separate the subnet masks with semicolons (;). Example: 192.168.0.0/16;10.0.0.0/8.
* You can specify up to 40 subnet masks. The total length of the subnet masks can be a maximum of 512 characters.
*
* example:
* 10.0.0.0/8
*/
@NameInMap("LoginNetworkMasks")
public String loginNetworkMasks;
/**
* The validity period of the logon session of RAM users.
* Valid values: 1 to 24. Unit: hours.
* Default value: 6.
*
* example:
* 6
*/
@NameInMap("LoginSessionDuration")
public Integer loginSessionDuration;
/**
* Specifies whether MFA is required for all RAM users when they log on to the Alibaba Cloud Management Console. This parameter is used to replace EnforceMFAForLogin. EnforceMFAForLogin is still valid. However, we recommend that you use MFAOperationForLogin. Valid values:
*
* - mandatory: MFA is required for all RAM users. If you use EnforceMFAForLogin, set the value to true.
* - independent (default): User-specific settings are applied. If you use EnforceMFAForLogin, set the value to false.
* - adaptive: MFA is required only for RAM users who initiated unusual logons.
*
*
* example:
* adaptive
*/
@NameInMap("MFAOperationForLogin")
public String MFAOperationForLogin;
/**
* Specifies whether to enable MFA for RAM users who initiated unusual logons. Valid values:
*
* - autonomous (default): yes. MFA is prompted for RAM users who initiated unusual logons. However, the RAM users are allowed to skip MFA.
* - enforceVerify: MFA is prompted for RAM users who initiated unusual logons and the RAM users cannot skip MFA.
*
*
* example:
* autonomous
*/
@NameInMap("OperationForRiskLogin")
public String operationForRiskLogin;
/**
* The MFA methods.
*/
@NameInMap("VerificationTypes")
public String verificationTypesShrink;
public static SetSecurityPreferenceShrinkRequest build(java.util.Map map) throws Exception {
SetSecurityPreferenceShrinkRequest self = new SetSecurityPreferenceShrinkRequest();
return TeaModel.build(map, self);
}
public SetSecurityPreferenceShrinkRequest setAllowUserToChangePassword(Boolean allowUserToChangePassword) {
this.allowUserToChangePassword = allowUserToChangePassword;
return this;
}
public Boolean getAllowUserToChangePassword() {
return this.allowUserToChangePassword;
}
public SetSecurityPreferenceShrinkRequest setAllowUserToLoginWithPasskey(Boolean allowUserToLoginWithPasskey) {
this.allowUserToLoginWithPasskey = allowUserToLoginWithPasskey;
return this;
}
public Boolean getAllowUserToLoginWithPasskey() {
return this.allowUserToLoginWithPasskey;
}
public SetSecurityPreferenceShrinkRequest setAllowUserToManageAccessKeys(Boolean allowUserToManageAccessKeys) {
this.allowUserToManageAccessKeys = allowUserToManageAccessKeys;
return this;
}
public Boolean getAllowUserToManageAccessKeys() {
return this.allowUserToManageAccessKeys;
}
public SetSecurityPreferenceShrinkRequest setAllowUserToManageMFADevices(Boolean allowUserToManageMFADevices) {
this.allowUserToManageMFADevices = allowUserToManageMFADevices;
return this;
}
public Boolean getAllowUserToManageMFADevices() {
return this.allowUserToManageMFADevices;
}
public SetSecurityPreferenceShrinkRequest setAllowUserToManagePersonalDingTalk(Boolean allowUserToManagePersonalDingTalk) {
this.allowUserToManagePersonalDingTalk = allowUserToManagePersonalDingTalk;
return this;
}
public Boolean getAllowUserToManagePersonalDingTalk() {
return this.allowUserToManagePersonalDingTalk;
}
public SetSecurityPreferenceShrinkRequest setEnableSaveMFATicket(Boolean enableSaveMFATicket) {
this.enableSaveMFATicket = enableSaveMFATicket;
return this;
}
public Boolean getEnableSaveMFATicket() {
return this.enableSaveMFATicket;
}
public SetSecurityPreferenceShrinkRequest setLoginNetworkMasks(String loginNetworkMasks) {
this.loginNetworkMasks = loginNetworkMasks;
return this;
}
public String getLoginNetworkMasks() {
return this.loginNetworkMasks;
}
public SetSecurityPreferenceShrinkRequest setLoginSessionDuration(Integer loginSessionDuration) {
this.loginSessionDuration = loginSessionDuration;
return this;
}
public Integer getLoginSessionDuration() {
return this.loginSessionDuration;
}
public SetSecurityPreferenceShrinkRequest setMFAOperationForLogin(String MFAOperationForLogin) {
this.MFAOperationForLogin = MFAOperationForLogin;
return this;
}
public String getMFAOperationForLogin() {
return this.MFAOperationForLogin;
}
public SetSecurityPreferenceShrinkRequest setOperationForRiskLogin(String operationForRiskLogin) {
this.operationForRiskLogin = operationForRiskLogin;
return this;
}
public String getOperationForRiskLogin() {
return this.operationForRiskLogin;
}
public SetSecurityPreferenceShrinkRequest setVerificationTypesShrink(String verificationTypesShrink) {
this.verificationTypesShrink = verificationTypesShrink;
return this;
}
public String getVerificationTypesShrink() {
return this.verificationTypesShrink;
}
}