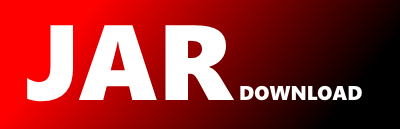
com.aliyun.iot20180120.models.ListRuleResponseBody Maven / Gradle / Ivy
Show all versions of iot20180120 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.iot20180120.models;
import com.aliyun.tea.*;
public class ListRuleResponseBody extends TeaModel {
/**
* The error code that is returned if the call fails. For more information, see Error codes.
*
* example:
* iot.system.SystemException
*/
@NameInMap("Code")
public String code;
/**
* The rules returned if the call is successful. For more information, see the "RuleInfo" section of this topic.
*
* The returned rules are sorted in reverse-chronological order based on the time when the rules were created.
*
*/
@NameInMap("Data")
public ListRuleResponseBodyData data;
/**
* The error message that is returned if the call fails.
*/
@NameInMap("ErrorMessage")
public String errorMessage;
/**
* The page number of the returned page.
*
* example:
* 1
*/
@NameInMap("Page")
public Integer page;
/**
* The number of entries returned per page.
*
* example:
* 2
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The ID of the request.
*
* example:
* 1564B626-DE97-452D-9E9B-305888AC6105
*/
@NameInMap("RequestId")
public String requestId;
/**
* Indicates whether the call was successful. Valid values:
*
* - true: The call was successful.
* - false: The call failed.
*
*
* example:
* true
*/
@NameInMap("Success")
public Boolean success;
/**
* The total number of pages returned.
*
* example:
* 25
*/
@NameInMap("Total")
public Integer total;
public static ListRuleResponseBody build(java.util.Map map) throws Exception {
ListRuleResponseBody self = new ListRuleResponseBody();
return TeaModel.build(map, self);
}
public ListRuleResponseBody setCode(String code) {
this.code = code;
return this;
}
public String getCode() {
return this.code;
}
public ListRuleResponseBody setData(ListRuleResponseBodyData data) {
this.data = data;
return this;
}
public ListRuleResponseBodyData getData() {
return this.data;
}
public ListRuleResponseBody setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
public String getErrorMessage() {
return this.errorMessage;
}
public ListRuleResponseBody setPage(Integer page) {
this.page = page;
return this;
}
public Integer getPage() {
return this.page;
}
public ListRuleResponseBody setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public ListRuleResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public ListRuleResponseBody setSuccess(Boolean success) {
this.success = success;
return this;
}
public Boolean getSuccess() {
return this.success;
}
public ListRuleResponseBody setTotal(Integer total) {
this.total = total;
return this;
}
public Integer getTotal() {
return this.total;
}
public static class ListRuleResponseBodyDataRuleInfo extends TeaModel {
/**
* The ID of the user who created the rule.
*
* example:
* 1231579085000000
*/
@NameInMap("CreateUserId")
public Long createUserId;
/**
* The time when the rule was created. The time is displayed in UTC-6.
*
* example:
* Wed Feb 27 20:45:43 CST 2019
*/
@NameInMap("Created")
public String created;
/**
* The data type of the rule. Valid values: JSON and BINARY.
*
* example:
* JSON
*/
@NameInMap("DataType")
public String dataType;
/**
* The ID of the rule.
*
* example:
* 151454
*/
@NameInMap("Id")
public Long id;
/**
* The time when the rule was last modified. The time is displayed in UTC-6.
*
* example:
* Wed Feb 27 20:45:43 CST 2019
*/
@NameInMap("Modified")
public String modified;
/**
* The name of the rule.
*
* example:
* test123
*/
@NameInMap("Name")
public String name;
/**
* The ProductKey of the product to which the rule applies.
*
* example:
* a1KiV******
*/
@NameInMap("ProductKey")
public String productKey;
/**
* The description of the rule.
*
* example:
* rule1Desc
*/
@NameInMap("RuleDesc")
public String ruleDesc;
/**
* The content that follows the Select keyword in the SQL statement of the rule.
*
* example:
* deviceName() as deviceName
*/
@NameInMap("Select")
public String select;
/**
* The topic to which the rule applies. The topic does not include the ProductKey level. Format: ${deviceName}/topicShortName
. ${deviceName} indicates the name of the device, and topicShortName indicates the custom name of the topic.
*
* For information about how to use a plus sign (+
) or a number sign (#
) as a wildcard character in a topic, see Topic wildcards.
*
*
* example:
* +/thing/event/property/post
*/
@NameInMap("ShortTopic")
public String shortTopic;
/**
* The status of the rule. Valid values:
*
* - RUNNING: The rule is running.
* - STOP: The rule is disabled.
*
*
* example:
* STOP
*/
@NameInMap("Status")
public String status;
/**
* The topic to which the rule applies. Format: ${productKey}/${deviceName}/topicShortName
.
*
* For information about how to use a plus sign (+
) or a number sign (#
) as a wildcard character in a topic, see Topic wildcards.
*
*/
@NameInMap("Topic")
public String topic;
/**
* The time when the device was created. The time is displayed in UTC.
*
* example:
* 2019-02-27T12:40:43.000Z
*/
@NameInMap("UtcCreated")
public String utcCreated;
/**
* The time when the rule was last modified.
*
* example:
* 2019-02-27T12:45:43.000Z
*/
@NameInMap("UtcModified")
public String utcModified;
/**
* The Where query condition in the SQL statement of the rule.
*
* example:
* Temperature>35
*/
@NameInMap("Where")
public String where;
public static ListRuleResponseBodyDataRuleInfo build(java.util.Map map) throws Exception {
ListRuleResponseBodyDataRuleInfo self = new ListRuleResponseBodyDataRuleInfo();
return TeaModel.build(map, self);
}
public ListRuleResponseBodyDataRuleInfo setCreateUserId(Long createUserId) {
this.createUserId = createUserId;
return this;
}
public Long getCreateUserId() {
return this.createUserId;
}
public ListRuleResponseBodyDataRuleInfo setCreated(String created) {
this.created = created;
return this;
}
public String getCreated() {
return this.created;
}
public ListRuleResponseBodyDataRuleInfo setDataType(String dataType) {
this.dataType = dataType;
return this;
}
public String getDataType() {
return this.dataType;
}
public ListRuleResponseBodyDataRuleInfo setId(Long id) {
this.id = id;
return this;
}
public Long getId() {
return this.id;
}
public ListRuleResponseBodyDataRuleInfo setModified(String modified) {
this.modified = modified;
return this;
}
public String getModified() {
return this.modified;
}
public ListRuleResponseBodyDataRuleInfo setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public ListRuleResponseBodyDataRuleInfo setProductKey(String productKey) {
this.productKey = productKey;
return this;
}
public String getProductKey() {
return this.productKey;
}
public ListRuleResponseBodyDataRuleInfo setRuleDesc(String ruleDesc) {
this.ruleDesc = ruleDesc;
return this;
}
public String getRuleDesc() {
return this.ruleDesc;
}
public ListRuleResponseBodyDataRuleInfo setSelect(String select) {
this.select = select;
return this;
}
public String getSelect() {
return this.select;
}
public ListRuleResponseBodyDataRuleInfo setShortTopic(String shortTopic) {
this.shortTopic = shortTopic;
return this;
}
public String getShortTopic() {
return this.shortTopic;
}
public ListRuleResponseBodyDataRuleInfo setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
public ListRuleResponseBodyDataRuleInfo setTopic(String topic) {
this.topic = topic;
return this;
}
public String getTopic() {
return this.topic;
}
public ListRuleResponseBodyDataRuleInfo setUtcCreated(String utcCreated) {
this.utcCreated = utcCreated;
return this;
}
public String getUtcCreated() {
return this.utcCreated;
}
public ListRuleResponseBodyDataRuleInfo setUtcModified(String utcModified) {
this.utcModified = utcModified;
return this;
}
public String getUtcModified() {
return this.utcModified;
}
public ListRuleResponseBodyDataRuleInfo setWhere(String where) {
this.where = where;
return this;
}
public String getWhere() {
return this.where;
}
}
public static class ListRuleResponseBodyData extends TeaModel {
@NameInMap("RuleInfo")
public java.util.List ruleInfo;
public static ListRuleResponseBodyData build(java.util.Map map) throws Exception {
ListRuleResponseBodyData self = new ListRuleResponseBodyData();
return TeaModel.build(map, self);
}
public ListRuleResponseBodyData setRuleInfo(java.util.List ruleInfo) {
this.ruleInfo = ruleInfo;
return this;
}
public java.util.List getRuleInfo() {
return this.ruleInfo;
}
}
}