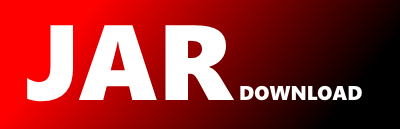
com.aliyun.iot20180120.models.QueryShareTaskDeviceListResponseBody Maven / Gradle / Ivy
Show all versions of iot20180120 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.iot20180120.models;
import com.aliyun.tea.*;
public class QueryShareTaskDeviceListResponseBody extends TeaModel {
/**
* The error code returned if the request fails. For more information, see Error codes.
*
* example:
* iot.system.SystemException
*/
@NameInMap("Code")
public String code;
/**
* The result of the request.
*/
@NameInMap("Data")
public QueryShareTaskDeviceListResponseBodyData data;
/**
* The error message returned if the request fails.
*
* example:
* request parameter error
*/
@NameInMap("ErrorMessage")
public String errorMessage;
/**
* The request ID.
*
* example:
* E55E50B7-40EE-4B6B-8BBE-D3ED55CCF565
*/
@NameInMap("RequestId")
public String requestId;
/**
* Indicates whether the request was successful. Valid values:
*
* - true
* - false
*
*
* example:
* true
*/
@NameInMap("Success")
public Boolean success;
public static QueryShareTaskDeviceListResponseBody build(java.util.Map map) throws Exception {
QueryShareTaskDeviceListResponseBody self = new QueryShareTaskDeviceListResponseBody();
return TeaModel.build(map, self);
}
public QueryShareTaskDeviceListResponseBody setCode(String code) {
this.code = code;
return this;
}
public String getCode() {
return this.code;
}
public QueryShareTaskDeviceListResponseBody setData(QueryShareTaskDeviceListResponseBodyData data) {
this.data = data;
return this;
}
public QueryShareTaskDeviceListResponseBodyData getData() {
return this.data;
}
public QueryShareTaskDeviceListResponseBody setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
public String getErrorMessage() {
return this.errorMessage;
}
public QueryShareTaskDeviceListResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public QueryShareTaskDeviceListResponseBody setSuccess(Boolean success) {
this.success = success;
return this;
}
public Boolean getSuccess() {
return this.success;
}
public static class QueryShareTaskDeviceListResponseBodyDataDeviceListItems extends TeaModel {
/**
* The DeviceName of the device.
*
* example:
* test
*/
@NameInMap("DeviceName")
public String deviceName;
/**
* The time when the device was added to the sharing task.
*
* example:
* 1620624606000
*/
@NameInMap("GmtAdded")
public Long gmtAdded;
/**
* The ID of the device. The ID is the unique identifier that is issued by IoT Platform to the device.
*
* example:
* Q7uOhVRdZRRlDnTLv****00100
*/
@NameInMap("IotId")
public String iotId;
/**
* The ProductKey of the product to which the device belongs.
*
* example:
* a1BwAGV****
*/
@NameInMap("ProductKey")
public String productKey;
public static QueryShareTaskDeviceListResponseBodyDataDeviceListItems build(java.util.Map map) throws Exception {
QueryShareTaskDeviceListResponseBodyDataDeviceListItems self = new QueryShareTaskDeviceListResponseBodyDataDeviceListItems();
return TeaModel.build(map, self);
}
public QueryShareTaskDeviceListResponseBodyDataDeviceListItems setDeviceName(String deviceName) {
this.deviceName = deviceName;
return this;
}
public String getDeviceName() {
return this.deviceName;
}
public QueryShareTaskDeviceListResponseBodyDataDeviceListItems setGmtAdded(Long gmtAdded) {
this.gmtAdded = gmtAdded;
return this;
}
public Long getGmtAdded() {
return this.gmtAdded;
}
public QueryShareTaskDeviceListResponseBodyDataDeviceListItems setIotId(String iotId) {
this.iotId = iotId;
return this;
}
public String getIotId() {
return this.iotId;
}
public QueryShareTaskDeviceListResponseBodyDataDeviceListItems setProductKey(String productKey) {
this.productKey = productKey;
return this;
}
public String getProductKey() {
return this.productKey;
}
}
public static class QueryShareTaskDeviceListResponseBodyDataDeviceList extends TeaModel {
@NameInMap("items")
public java.util.List items;
public static QueryShareTaskDeviceListResponseBodyDataDeviceList build(java.util.Map map) throws Exception {
QueryShareTaskDeviceListResponseBodyDataDeviceList self = new QueryShareTaskDeviceListResponseBodyDataDeviceList();
return TeaModel.build(map, self);
}
public QueryShareTaskDeviceListResponseBodyDataDeviceList setItems(java.util.List items) {
this.items = items;
return this;
}
public java.util.List getItems() {
return this.items;
}
}
public static class QueryShareTaskDeviceListResponseBodyData extends TeaModel {
/**
* The devices in the sharing task.
*/
@NameInMap("DeviceList")
public QueryShareTaskDeviceListResponseBodyDataDeviceList deviceList;
/**
* The page number.
*
* example:
* 1
*/
@NameInMap("PageId")
public Integer pageId;
/**
* The number of entries per page.
*
* example:
* 20
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The total number of entries returned.
*
* example:
* 200
*/
@NameInMap("Total")
public Integer total;
public static QueryShareTaskDeviceListResponseBodyData build(java.util.Map map) throws Exception {
QueryShareTaskDeviceListResponseBodyData self = new QueryShareTaskDeviceListResponseBodyData();
return TeaModel.build(map, self);
}
public QueryShareTaskDeviceListResponseBodyData setDeviceList(QueryShareTaskDeviceListResponseBodyDataDeviceList deviceList) {
this.deviceList = deviceList;
return this;
}
public QueryShareTaskDeviceListResponseBodyDataDeviceList getDeviceList() {
return this.deviceList;
}
public QueryShareTaskDeviceListResponseBodyData setPageId(Integer pageId) {
this.pageId = pageId;
return this;
}
public Integer getPageId() {
return this.pageId;
}
public QueryShareTaskDeviceListResponseBodyData setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public QueryShareTaskDeviceListResponseBodyData setTotal(Integer total) {
this.total = total;
return this;
}
public Integer getTotal() {
return this.total;
}
}
}