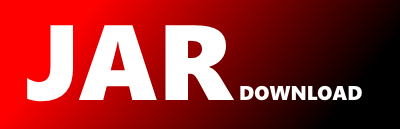
com.aliyun.r_kvstore20150101.models.DescribeInstancesOverviewResponseBody Maven / Gradle / Ivy
Show all versions of r_kvstore20150101 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.r_kvstore20150101.models;
import com.aliyun.tea.*;
public class DescribeInstancesOverviewResponseBody extends TeaModel {
/**
* An array of instances.
*/
@NameInMap("Instances")
public java.util.List instances;
/**
* The ID of the request.
*
* example:
* 1E83311F-0EE4-4922-A3BF-730B312B****
*/
@NameInMap("RequestId")
public String requestId;
/**
* The total number of instances.
*
* example:
* 1
*/
@NameInMap("TotalCount")
public Integer totalCount;
public static DescribeInstancesOverviewResponseBody build(java.util.Map map) throws Exception {
DescribeInstancesOverviewResponseBody self = new DescribeInstancesOverviewResponseBody();
return TeaModel.build(map, self);
}
public DescribeInstancesOverviewResponseBody setInstances(java.util.List instances) {
this.instances = instances;
return this;
}
public java.util.List getInstances() {
return this.instances;
}
public DescribeInstancesOverviewResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeInstancesOverviewResponseBody setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
public static class DescribeInstancesOverviewResponseBodyInstances extends TeaModel {
/**
* The architecture of the instance. Valid values:
*
* - cluster: cluster architecture
* - standard: standard architecture
* - rwsplit: read/write splitting architecture
*
*
* example:
* cluster
*/
@NameInMap("ArchitectureType")
public String architectureType;
/**
* The storage capacity of the instance. Unit: MB.
*
* example:
* 4096
*/
@NameInMap("Capacity")
public Long capacity;
/**
* The billing method of the instance. Valid values:
*
* - PrePaid: subscription
* - PostPaid: pay-as-you-go
*
*
* example:
* PostPaid
*/
@NameInMap("ChargeType")
public String chargeType;
/**
* The internal endpoint of the instance.
*
* example:
* r-bp1zxszhcgatnx****.redis.rds.aliyuncs.com
*/
@NameInMap("ConnectionDomain")
public String connectionDomain;
/**
* The time when the instance was created.
*
* example:
* 2018-11-07T08:49:00Z
*/
@NameInMap("CreateTime")
public String createTime;
/**
* The time when the subscription instance expires.
*
* example:
* 2022-06-13T16:00:00Z
*/
@NameInMap("EndTime")
public String endTime;
/**
* The database engine version of the instance. Valid values: 2.8, 4.0, and 5.0.
*
* example:
* 4.0
*/
@NameInMap("EngineVersion")
public String engineVersion;
/**
* The ID of the distributed instance.
*
* This parameter is returned only when the instance is a child instance of a distributed instance.
*
*
* example:
* gr-bp14rkqrhac****
*/
@NameInMap("GlobalInstanceId")
public String globalInstanceId;
/**
* The instance type of the instance.
*
* example:
* redis.logic.sharding.2g.2db.0rodb.4proxy.default
*/
@NameInMap("InstanceClass")
public String instanceClass;
/**
* The ID of the instance.
*
* example:
* r-bp1zxszhcgatnx****
*/
@NameInMap("InstanceId")
public String instanceId;
/**
* The name of the instance.
*
* example:
* apitest
*/
@NameInMap("InstanceName")
public String instanceName;
/**
* The state of the instance. Valid values:
*
* - Normal: The instance is normal.
* - Creating: The instance is being created.
* - Changing: The configurations of the instance are being changed.
* - Inactive: The instance is disabled.
* - Flushing: The instance is being released.
* - Released: The instance is released.
* - Transforming: The billing method of the instance is being changed.
* - Unavailable: The instance is unavailable.
* - Error: The instance failed to be created.
* - Migrating: The instance is being migrated.
* - BackupRecovering: The instance is being restored from a backup.
* - MinorVersionUpgrading: The minor version of the instance is being updated.
* - NetworkModifying: The network type of the instance is being changed.
* - SSLModifying: The SSL certificate of the instance is being changed.
* - MajorVersionUpgrading: The major version of the instance is being upgraded. The instance remains accessible during the upgrade.
*
*
* example:
* Normal
*/
@NameInMap("InstanceStatus")
public String instanceStatus;
/**
* The category of the instance. Valid values:
*
* - Tair
* - Redis
* - Memcache
*
*
* example:
* Redis
*/
@NameInMap("InstanceType")
public String instanceType;
/**
* The network type of the instance. Valid values:
*
* - CLASSIC: classic network
* - VPC: VPC
*
*
* example:
* CLASSIC
*/
@NameInMap("NetworkType")
public String networkType;
/**
* The private IP address of the instance.
*
* This parameter is not returned when the instance is deployed in the classic network.
*
*
* example:
* 172.16.49.***
*/
@NameInMap("PrivateIp")
public String privateIp;
/**
* The region ID of the instance.
*
* example:
* cn-hangzhou
*/
@NameInMap("RegionId")
public String regionId;
/**
* The ID of the resource group to which the instance belongs.
*
* example:
* rg-acfmyiu4ekp****
*/
@NameInMap("ResourceGroupId")
public String resourceGroupId;
/**
* Instance\"s secondary zone id.
*
* This parameter is only returned when the instance has a secondary zone ID.
*
*
* example:
* cn-hangzhou-g
*/
@NameInMap("SecondaryZoneId")
public String secondaryZoneId;
/**
* The ID of the vSwitch to which the instance is connected.
*
* example:
* vsw-bp1e7clcw529l773d****
*/
@NameInMap("VSwitchId")
public String vSwitchId;
/**
* The ID of the VPC.
*
* example:
* vpc-bp1nme44gek34slfc****
*/
@NameInMap("VpcId")
public String vpcId;
/**
* The zone ID of the instance.
*
* example:
* cn-hangzhou-b
*/
@NameInMap("ZoneId")
public String zoneId;
public static DescribeInstancesOverviewResponseBodyInstances build(java.util.Map map) throws Exception {
DescribeInstancesOverviewResponseBodyInstances self = new DescribeInstancesOverviewResponseBodyInstances();
return TeaModel.build(map, self);
}
public DescribeInstancesOverviewResponseBodyInstances setArchitectureType(String architectureType) {
this.architectureType = architectureType;
return this;
}
public String getArchitectureType() {
return this.architectureType;
}
public DescribeInstancesOverviewResponseBodyInstances setCapacity(Long capacity) {
this.capacity = capacity;
return this;
}
public Long getCapacity() {
return this.capacity;
}
public DescribeInstancesOverviewResponseBodyInstances setChargeType(String chargeType) {
this.chargeType = chargeType;
return this;
}
public String getChargeType() {
return this.chargeType;
}
public DescribeInstancesOverviewResponseBodyInstances setConnectionDomain(String connectionDomain) {
this.connectionDomain = connectionDomain;
return this;
}
public String getConnectionDomain() {
return this.connectionDomain;
}
public DescribeInstancesOverviewResponseBodyInstances setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
public String getCreateTime() {
return this.createTime;
}
public DescribeInstancesOverviewResponseBodyInstances setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
public String getEndTime() {
return this.endTime;
}
public DescribeInstancesOverviewResponseBodyInstances setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
public String getEngineVersion() {
return this.engineVersion;
}
public DescribeInstancesOverviewResponseBodyInstances setGlobalInstanceId(String globalInstanceId) {
this.globalInstanceId = globalInstanceId;
return this;
}
public String getGlobalInstanceId() {
return this.globalInstanceId;
}
public DescribeInstancesOverviewResponseBodyInstances setInstanceClass(String instanceClass) {
this.instanceClass = instanceClass;
return this;
}
public String getInstanceClass() {
return this.instanceClass;
}
public DescribeInstancesOverviewResponseBodyInstances setInstanceId(String instanceId) {
this.instanceId = instanceId;
return this;
}
public String getInstanceId() {
return this.instanceId;
}
public DescribeInstancesOverviewResponseBodyInstances setInstanceName(String instanceName) {
this.instanceName = instanceName;
return this;
}
public String getInstanceName() {
return this.instanceName;
}
public DescribeInstancesOverviewResponseBodyInstances setInstanceStatus(String instanceStatus) {
this.instanceStatus = instanceStatus;
return this;
}
public String getInstanceStatus() {
return this.instanceStatus;
}
public DescribeInstancesOverviewResponseBodyInstances setInstanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
public String getInstanceType() {
return this.instanceType;
}
public DescribeInstancesOverviewResponseBodyInstances setNetworkType(String networkType) {
this.networkType = networkType;
return this;
}
public String getNetworkType() {
return this.networkType;
}
public DescribeInstancesOverviewResponseBodyInstances setPrivateIp(String privateIp) {
this.privateIp = privateIp;
return this;
}
public String getPrivateIp() {
return this.privateIp;
}
public DescribeInstancesOverviewResponseBodyInstances setRegionId(String regionId) {
this.regionId = regionId;
return this;
}
public String getRegionId() {
return this.regionId;
}
public DescribeInstancesOverviewResponseBodyInstances setResourceGroupId(String resourceGroupId) {
this.resourceGroupId = resourceGroupId;
return this;
}
public String getResourceGroupId() {
return this.resourceGroupId;
}
public DescribeInstancesOverviewResponseBodyInstances setSecondaryZoneId(String secondaryZoneId) {
this.secondaryZoneId = secondaryZoneId;
return this;
}
public String getSecondaryZoneId() {
return this.secondaryZoneId;
}
public DescribeInstancesOverviewResponseBodyInstances setVSwitchId(String vSwitchId) {
this.vSwitchId = vSwitchId;
return this;
}
public String getVSwitchId() {
return this.vSwitchId;
}
public DescribeInstancesOverviewResponseBodyInstances setVpcId(String vpcId) {
this.vpcId = vpcId;
return this;
}
public String getVpcId() {
return this.vpcId;
}
public DescribeInstancesOverviewResponseBodyInstances setZoneId(String zoneId) {
this.zoneId = zoneId;
return this;
}
public String getZoneId() {
return this.zoneId;
}
}
}