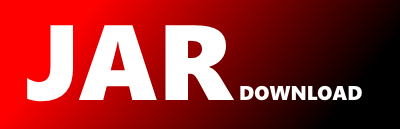
com.aliyun.rds20140815.models.DescribeCrossRegionBackupsResponseBody Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.rds20140815.models;
import com.aliyun.tea.*;
public class DescribeCrossRegionBackupsResponseBody extends TeaModel {
/**
* The end of the time range to query.
*
* example:
* 2019-06-15T12:10:00Z
*/
@NameInMap("EndTime")
public String endTime;
/**
* The cross-region data backup files.
*/
@NameInMap("Items")
public DescribeCrossRegionBackupsResponseBodyItems items;
/**
* The page number. Pages start from page 1.
* Default value: 1.
*
* example:
* 1
*/
@NameInMap("PageNumber")
public Integer pageNumber;
/**
* The number of cross-region data backup files on the current page.
*
* example:
* 30
*/
@NameInMap("PageRecordCount")
public Integer pageRecordCount;
/**
* The region ID of the instance.
*
* example:
* cn-hangzhou
*/
@NameInMap("RegionId")
public String regionId;
/**
* The request ID.
*
* example:
* 60912B41-7579-4B5D-B289-8856030F0A6A
*/
@NameInMap("RequestId")
public String requestId;
/**
* The beginning of the time range to query.
*
* example:
* 2019-05-30T12:10:00Z
*/
@NameInMap("StartTime")
public String startTime;
/**
* The total number of entries that are returned.
*
* example:
* 100
*/
@NameInMap("TotalRecordCount")
public Integer totalRecordCount;
public static DescribeCrossRegionBackupsResponseBody build(java.util.Map map) throws Exception {
DescribeCrossRegionBackupsResponseBody self = new DescribeCrossRegionBackupsResponseBody();
return TeaModel.build(map, self);
}
public DescribeCrossRegionBackupsResponseBody setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
public String getEndTime() {
return this.endTime;
}
public DescribeCrossRegionBackupsResponseBody setItems(DescribeCrossRegionBackupsResponseBodyItems items) {
this.items = items;
return this;
}
public DescribeCrossRegionBackupsResponseBodyItems getItems() {
return this.items;
}
public DescribeCrossRegionBackupsResponseBody setPageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
public Integer getPageNumber() {
return this.pageNumber;
}
public DescribeCrossRegionBackupsResponseBody setPageRecordCount(Integer pageRecordCount) {
this.pageRecordCount = pageRecordCount;
return this;
}
public Integer getPageRecordCount() {
return this.pageRecordCount;
}
public DescribeCrossRegionBackupsResponseBody setRegionId(String regionId) {
this.regionId = regionId;
return this;
}
public String getRegionId() {
return this.regionId;
}
public DescribeCrossRegionBackupsResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeCrossRegionBackupsResponseBody setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
public String getStartTime() {
return this.startTime;
}
public DescribeCrossRegionBackupsResponseBody setTotalRecordCount(Integer totalRecordCount) {
this.totalRecordCount = totalRecordCount;
return this;
}
public Integer getTotalRecordCount() {
return this.totalRecordCount;
}
public static class DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions extends TeaModel {
@NameInMap("RestoreRegion")
public java.util.List restoreRegion;
public static DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions build(java.util.Map map) throws Exception {
DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions self = new DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions();
return TeaModel.build(map, self);
}
public DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions setRestoreRegion(java.util.List restoreRegion) {
this.restoreRegion = restoreRegion;
return this;
}
public java.util.List getRestoreRegion() {
return this.restoreRegion;
}
}
public static class DescribeCrossRegionBackupsResponseBodyItemsItem extends TeaModel {
/**
* The time when the cross-region data backup file was generated.
*
* example:
* 2019-06-15T12:10:00Z
*/
@NameInMap("BackupEndTime")
public String backupEndTime;
/**
* The method that is used to generate the cross-region data backup file. Valid values:
*
* - L: logical backup
* - P: physical backup
*
*
* example:
* P
*/
@NameInMap("BackupMethod")
public String backupMethod;
/**
* The level at which the cross-region data backup file is generated.
*
* - 0: instance-level backup
* - 1: database-level backup
*
*
* example:
* 0
*/
@NameInMap("BackupSetScale")
public Integer backupSetScale;
/**
* The status of the cross-region data backup. Valid values:
*
* - 0: The cross-region data backup is successful.
* - 1: The cross-region data backup failed.
*
*
* example:
* 0
*/
@NameInMap("BackupSetStatus")
public Integer backupSetStatus;
/**
* The time when the cross-region data backup started.
*
* example:
* 2019-05-30T12:10:00Z
*/
@NameInMap("BackupStartTime")
public String backupStartTime;
/**
* The type of the cross-region data backup. Valid values:
*
* - F: full data backup
* - I: incremental data backup
*
*
* example:
* F
*/
@NameInMap("BackupType")
public String backupType;
/**
* The RDS edition of the instance. Valid values:
*
* - Basic: RDS Basic Edition.
* - HighAvailability: RDS High-availability Edition.
* - Finance: RDS Enterprise Edition. This edition is available only for the China site (aliyun.com).
*
*
* example:
* HighAvailability
*/
@NameInMap("Category")
public String category;
/**
* The point in time that is indicated by the data in the cross-region data backup file.
*
* example:
* 2019-06-12T05:44:46Z
*/
@NameInMap("ConsistentTime")
public String consistentTime;
/**
* The external URL from which you can download the cross-region data backup file.
*
* example:
* http://rdsddrbak-shanghai.oss-cn-shanghai.aliyuncs.com/xxxxx
*/
@NameInMap("CrossBackupDownloadLink")
public String crossBackupDownloadLink;
/**
* The ID of the cross-region data backup file.
*
* example:
* 14377
*/
@NameInMap("CrossBackupId")
public Integer crossBackupId;
/**
* The ID of the region in which the cross-region backup files of the instance are stored.
*
* example:
* cn-shanghai
*/
@NameInMap("CrossBackupRegion")
public String crossBackupRegion;
/**
* The name of the compressed package that contains the cross-region data backup file.
*
* example:
* cn-hangzhou_rm-xxxxx_hins81xxx_data_20190612134426_qp.xb
*/
@NameInMap("CrossBackupSetFile")
public String crossBackupSetFile;
/**
* The location where the cross-region data backup file is stored.
*
* example:
* oss
*/
@NameInMap("CrossBackupSetLocation")
public String crossBackupSetLocation;
/**
* The size of the cross-region data backup file. Unit: bytes.
*
* example:
* 5312836
*/
@NameInMap("CrossBackupSetSize")
public Long crossBackupSetSize;
/**
* The storage type. Valid values:
*
* - local_ssd: local SSDs. This is the recommended storage type.
* - cloud_ssd: standard SSD.
* - cloud_essd: enhanced SSD (ESSD).
*
*
* example:
* ssd
*/
@NameInMap("DBInstanceStorageType")
public String DBInstanceStorageType;
/**
* The database engine of the instance.
*
* example:
* mysql
*/
@NameInMap("Engine")
public String engine;
/**
* The database engine version.
*
* example:
* 5.6
*/
@NameInMap("EngineVersion")
public String engineVersion;
/**
* The instance ID. This parameter is used to determine whether the instance that generates the cross-region data backup file is a primary or secondary instance.
*
* example:
* 8161055
*/
@NameInMap("InstanceId")
public Integer instanceId;
/**
* The regions to which the cross-region data backup file can be restored.
*/
@NameInMap("RestoreRegions")
public DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions restoreRegions;
public static DescribeCrossRegionBackupsResponseBodyItemsItem build(java.util.Map map) throws Exception {
DescribeCrossRegionBackupsResponseBodyItemsItem self = new DescribeCrossRegionBackupsResponseBodyItemsItem();
return TeaModel.build(map, self);
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setBackupEndTime(String backupEndTime) {
this.backupEndTime = backupEndTime;
return this;
}
public String getBackupEndTime() {
return this.backupEndTime;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setBackupMethod(String backupMethod) {
this.backupMethod = backupMethod;
return this;
}
public String getBackupMethod() {
return this.backupMethod;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setBackupSetScale(Integer backupSetScale) {
this.backupSetScale = backupSetScale;
return this;
}
public Integer getBackupSetScale() {
return this.backupSetScale;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setBackupSetStatus(Integer backupSetStatus) {
this.backupSetStatus = backupSetStatus;
return this;
}
public Integer getBackupSetStatus() {
return this.backupSetStatus;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setBackupStartTime(String backupStartTime) {
this.backupStartTime = backupStartTime;
return this;
}
public String getBackupStartTime() {
return this.backupStartTime;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setBackupType(String backupType) {
this.backupType = backupType;
return this;
}
public String getBackupType() {
return this.backupType;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setCategory(String category) {
this.category = category;
return this;
}
public String getCategory() {
return this.category;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setConsistentTime(String consistentTime) {
this.consistentTime = consistentTime;
return this;
}
public String getConsistentTime() {
return this.consistentTime;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setCrossBackupDownloadLink(String crossBackupDownloadLink) {
this.crossBackupDownloadLink = crossBackupDownloadLink;
return this;
}
public String getCrossBackupDownloadLink() {
return this.crossBackupDownloadLink;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setCrossBackupId(Integer crossBackupId) {
this.crossBackupId = crossBackupId;
return this;
}
public Integer getCrossBackupId() {
return this.crossBackupId;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setCrossBackupRegion(String crossBackupRegion) {
this.crossBackupRegion = crossBackupRegion;
return this;
}
public String getCrossBackupRegion() {
return this.crossBackupRegion;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setCrossBackupSetFile(String crossBackupSetFile) {
this.crossBackupSetFile = crossBackupSetFile;
return this;
}
public String getCrossBackupSetFile() {
return this.crossBackupSetFile;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setCrossBackupSetLocation(String crossBackupSetLocation) {
this.crossBackupSetLocation = crossBackupSetLocation;
return this;
}
public String getCrossBackupSetLocation() {
return this.crossBackupSetLocation;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setCrossBackupSetSize(Long crossBackupSetSize) {
this.crossBackupSetSize = crossBackupSetSize;
return this;
}
public Long getCrossBackupSetSize() {
return this.crossBackupSetSize;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setDBInstanceStorageType(String DBInstanceStorageType) {
this.DBInstanceStorageType = DBInstanceStorageType;
return this;
}
public String getDBInstanceStorageType() {
return this.DBInstanceStorageType;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setEngine(String engine) {
this.engine = engine;
return this;
}
public String getEngine() {
return this.engine;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
public String getEngineVersion() {
return this.engineVersion;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setInstanceId(Integer instanceId) {
this.instanceId = instanceId;
return this;
}
public Integer getInstanceId() {
return this.instanceId;
}
public DescribeCrossRegionBackupsResponseBodyItemsItem setRestoreRegions(DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions restoreRegions) {
this.restoreRegions = restoreRegions;
return this;
}
public DescribeCrossRegionBackupsResponseBodyItemsItemRestoreRegions getRestoreRegions() {
return this.restoreRegions;
}
}
public static class DescribeCrossRegionBackupsResponseBodyItems extends TeaModel {
@NameInMap("Item")
public java.util.List item;
public static DescribeCrossRegionBackupsResponseBodyItems build(java.util.Map map) throws Exception {
DescribeCrossRegionBackupsResponseBodyItems self = new DescribeCrossRegionBackupsResponseBodyItems();
return TeaModel.build(map, self);
}
public DescribeCrossRegionBackupsResponseBodyItems setItem(java.util.List item) {
this.item = item;
return this;
}
public java.util.List getItem() {
return this.item;
}
}
}