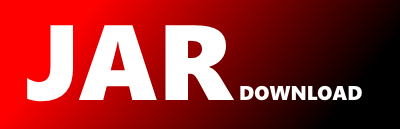
com.aliyun.rds20140815.models.DescribeDedicatedHostsResponseBody Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.rds20140815.models;
import com.aliyun.tea.*;
public class DescribeDedicatedHostsResponseBody extends TeaModel {
/**
* The host group ID.
*
* example:
* dhg-7a9xxxxxxxx
*/
@NameInMap("DedicatedHostGroupId")
public String dedicatedHostGroupId;
/**
* The host information.
*/
@NameInMap("DedicatedHosts")
public DescribeDedicatedHostsResponseBodyDedicatedHosts dedicatedHosts;
/**
* The request ID.
*
* example:
* C860658E-68A6-46C1-AF6E-3AE7C4D3CACF
*/
@NameInMap("RequestId")
public String requestId;
public static DescribeDedicatedHostsResponseBody build(java.util.Map map) throws Exception {
DescribeDedicatedHostsResponseBody self = new DescribeDedicatedHostsResponseBody();
return TeaModel.build(map, self);
}
public DescribeDedicatedHostsResponseBody setDedicatedHostGroupId(String dedicatedHostGroupId) {
this.dedicatedHostGroupId = dedicatedHostGroupId;
return this;
}
public String getDedicatedHostGroupId() {
return this.dedicatedHostGroupId;
}
public DescribeDedicatedHostsResponseBody setDedicatedHosts(DescribeDedicatedHostsResponseBodyDedicatedHosts dedicatedHosts) {
this.dedicatedHosts = dedicatedHosts;
return this;
}
public DescribeDedicatedHostsResponseBodyDedicatedHosts getDedicatedHosts() {
return this.dedicatedHosts;
}
public DescribeDedicatedHostsResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public static class DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts extends TeaModel {
/**
* The host account. You can call the CreateDedicatedHostAccount operation to create a host account.
*
* example:
* test123
*/
@NameInMap("AccountName")
public String accountName;
/**
* Specifies whether instances can be deployed on the host. Valid values:
*
* - 0: Instances cannot be deployed on the host.
* - 1: Instances can be deployed on the host.
*
*
* example:
* 1
*/
@NameInMap("AllocationStatus")
public String allocationStatus;
/**
* The bastion host ID.
*
* example:
* bastionhost-cn-m7xxxxxxxx
*/
@NameInMap("BastionInstanceId")
public String bastionInstanceId;
/**
* The core overcommitment ratio of the dedicated cluster. Unit: percentage. For more information about the core overcommitment ratio, see Manage a dedicated cluster.
*
* example:
* 200
*/
@NameInMap("CPUAllocationRatio")
public String CPUAllocationRatio;
/**
* The number of used CPU cores on the host. Unit: cores.
*
* example:
* 4
*/
@NameInMap("CpuUsed")
public String cpuUsed;
/**
* The time when the host was created.
*
* example:
* 2021-03-25 17:29:06.0
*/
@NameInMap("CreatedTime")
public String createdTime;
/**
* The dedicated cluster ID.
*
* example:
* dhg-7a9xxxxxxxx
*/
@NameInMap("DedicatedHostGroupId")
public String dedicatedHostGroupId;
/**
* The host ID.
*
* example:
* i-bpxxxxxxx
*/
@NameInMap("DedicatedHostId")
public String dedicatedHostId;
/**
* The disk overcommitment ratio of the dedicated cluster. Unit: percentage. For more information about the core overcommitment ratio, see Manage a dedicated cluster.
*
* example:
* 200
*/
@NameInMap("DiskAllocationRatio")
public String diskAllocationRatio;
/**
* The time when the host expires.
*
* example:
* 2021-04-25T16:00:00Z
*/
@NameInMap("EndTime")
public String endTime;
/**
* The database engine of instances that are created on the host.
*
* example:
* mysql
*/
@NameInMap("Engine")
public String engine;
/**
* The total number of CPU cores that are configured for the host. Unit: cores.
*
* example:
* 8
*/
@NameInMap("HostCPU")
public String hostCPU;
/**
* The instance type of the host.
*
* example:
* ecs.i2.16xlarge
*/
@NameInMap("HostClass")
public String hostClass;
/**
* The total memory space of the host. Unit: MB.
*
* example:
* 32238
*/
@NameInMap("HostMem")
public String hostMem;
/**
* The host name.
*
* example:
* testHost1
*/
@NameInMap("HostName")
public String hostName;
/**
* The status of the host. Valid values:
*
* - 0: creating
* - 1: running
* - 2: faulty
* - 3: being replaced
* - 4: deprecated
* - 5: deleting
* - 6: restarting
*
*
* example:
* 1
*/
@NameInMap("HostStatus")
public String hostStatus;
/**
* The storage capacity of the host. Unit: MB.
*
* example:
* 2097152
*/
@NameInMap("HostStorage")
public String hostStorage;
/**
* The storage type of the host. Valid values:
*
* - dhg_cloud_ssd: ESSD
* - dhg_local_ssd: local SSD
*
*
* example:
* dhg_cloud_ssd
*/
@NameInMap("HostType")
public String hostType;
/**
* The internal IP address of the host.
*
* example:
* 192.xx.xx.xx
*/
@NameInMap("IPAddress")
public String IPAddress;
/**
* The host image. This parameter is returned only when the Engine parameter is set to mssql. Valid values:
*
* - WindowsWithMssqlStdLicense: a Windows image that contains the licenses of SQL Server Standard Edition
* - WindowsWithMssqlEntLisence: a Windows image that contains the licenses of SQL Server Enterprise Edition
* - WindowsWithMssqlWebLisence: a Windows image that contains the licenses of SQL Server Web Edition
*
*
* example:
* WindowsWithMssqlStdLicense
*/
@NameInMap("ImageCategory")
public String imageCategory;
/**
* The total number of instances that are created on the host.
*
* example:
* 4
*/
@NameInMap("InstanceNumber")
public String instanceNumber;
/**
* The maximum memory usage per host in the dedicated cluster.
*
* example:
* 90
*/
@NameInMap("MemAllocationRatio")
public String memAllocationRatio;
/**
* The size of the used memory. Unit: MB.
*
* example:
* 16384
*/
@NameInMap("MemoryUsed")
public String memoryUsed;
/**
* Indicates whether the feature that allows you to have the OS permissions on the host is enabled. Valid values:
*
* - 0 or null: The permissions cannot be granted.
* - 1: The permissions can be granted.
* - 3: The permissions have been granted.
*
*
* example:
* 3
*/
@NameInMap("OpenPermission")
public String openPermission;
/**
* The amount of used storage space on the host.
*
* example:
* 0
*/
@NameInMap("StorageUsed")
public String storageUsed;
/**
* The ID of the virtual private cloud (VPC) to which the host belongs.
*
* example:
* vpc-bpxxxxxxx
*/
@NameInMap("VPCId")
public String VPCId;
/**
* The ID of the vSwitch associated with the specified VPC.
*
* example:
* vsw-bpxxxxxxx
*/
@NameInMap("VSwitchId")
public String vSwitchId;
/**
* The zone ID of the host.
*
* example:
* cn-hangzhou-i
*/
@NameInMap("ZoneId")
public String zoneId;
public static DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts build(java.util.Map map) throws Exception {
DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts self = new DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts();
return TeaModel.build(map, self);
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setAccountName(String accountName) {
this.accountName = accountName;
return this;
}
public String getAccountName() {
return this.accountName;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setAllocationStatus(String allocationStatus) {
this.allocationStatus = allocationStatus;
return this;
}
public String getAllocationStatus() {
return this.allocationStatus;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setBastionInstanceId(String bastionInstanceId) {
this.bastionInstanceId = bastionInstanceId;
return this;
}
public String getBastionInstanceId() {
return this.bastionInstanceId;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setCPUAllocationRatio(String CPUAllocationRatio) {
this.CPUAllocationRatio = CPUAllocationRatio;
return this;
}
public String getCPUAllocationRatio() {
return this.CPUAllocationRatio;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setCpuUsed(String cpuUsed) {
this.cpuUsed = cpuUsed;
return this;
}
public String getCpuUsed() {
return this.cpuUsed;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setCreatedTime(String createdTime) {
this.createdTime = createdTime;
return this;
}
public String getCreatedTime() {
return this.createdTime;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setDedicatedHostGroupId(String dedicatedHostGroupId) {
this.dedicatedHostGroupId = dedicatedHostGroupId;
return this;
}
public String getDedicatedHostGroupId() {
return this.dedicatedHostGroupId;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setDedicatedHostId(String dedicatedHostId) {
this.dedicatedHostId = dedicatedHostId;
return this;
}
public String getDedicatedHostId() {
return this.dedicatedHostId;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setDiskAllocationRatio(String diskAllocationRatio) {
this.diskAllocationRatio = diskAllocationRatio;
return this;
}
public String getDiskAllocationRatio() {
return this.diskAllocationRatio;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
public String getEndTime() {
return this.endTime;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setEngine(String engine) {
this.engine = engine;
return this;
}
public String getEngine() {
return this.engine;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setHostCPU(String hostCPU) {
this.hostCPU = hostCPU;
return this;
}
public String getHostCPU() {
return this.hostCPU;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setHostClass(String hostClass) {
this.hostClass = hostClass;
return this;
}
public String getHostClass() {
return this.hostClass;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setHostMem(String hostMem) {
this.hostMem = hostMem;
return this;
}
public String getHostMem() {
return this.hostMem;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setHostName(String hostName) {
this.hostName = hostName;
return this;
}
public String getHostName() {
return this.hostName;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setHostStatus(String hostStatus) {
this.hostStatus = hostStatus;
return this;
}
public String getHostStatus() {
return this.hostStatus;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setHostStorage(String hostStorage) {
this.hostStorage = hostStorage;
return this;
}
public String getHostStorage() {
return this.hostStorage;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setHostType(String hostType) {
this.hostType = hostType;
return this;
}
public String getHostType() {
return this.hostType;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setIPAddress(String IPAddress) {
this.IPAddress = IPAddress;
return this;
}
public String getIPAddress() {
return this.IPAddress;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setImageCategory(String imageCategory) {
this.imageCategory = imageCategory;
return this;
}
public String getImageCategory() {
return this.imageCategory;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setInstanceNumber(String instanceNumber) {
this.instanceNumber = instanceNumber;
return this;
}
public String getInstanceNumber() {
return this.instanceNumber;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setMemAllocationRatio(String memAllocationRatio) {
this.memAllocationRatio = memAllocationRatio;
return this;
}
public String getMemAllocationRatio() {
return this.memAllocationRatio;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setMemoryUsed(String memoryUsed) {
this.memoryUsed = memoryUsed;
return this;
}
public String getMemoryUsed() {
return this.memoryUsed;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setOpenPermission(String openPermission) {
this.openPermission = openPermission;
return this;
}
public String getOpenPermission() {
return this.openPermission;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setStorageUsed(String storageUsed) {
this.storageUsed = storageUsed;
return this;
}
public String getStorageUsed() {
return this.storageUsed;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setVPCId(String VPCId) {
this.VPCId = VPCId;
return this;
}
public String getVPCId() {
return this.VPCId;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setVSwitchId(String vSwitchId) {
this.vSwitchId = vSwitchId;
return this;
}
public String getVSwitchId() {
return this.vSwitchId;
}
public DescribeDedicatedHostsResponseBodyDedicatedHostsDedicatedHosts setZoneId(String zoneId) {
this.zoneId = zoneId;
return this;
}
public String getZoneId() {
return this.zoneId;
}
}
public static class DescribeDedicatedHostsResponseBodyDedicatedHosts extends TeaModel {
@NameInMap("DedicatedHosts")
public java.util.List dedicatedHosts;
public static DescribeDedicatedHostsResponseBodyDedicatedHosts build(java.util.Map map) throws Exception {
DescribeDedicatedHostsResponseBodyDedicatedHosts self = new DescribeDedicatedHostsResponseBodyDedicatedHosts();
return TeaModel.build(map, self);
}
public DescribeDedicatedHostsResponseBodyDedicatedHosts setDedicatedHosts(java.util.List dedicatedHosts) {
this.dedicatedHosts = dedicatedHosts;
return this;
}
public java.util.List getDedicatedHosts() {
return this.dedicatedHosts;
}
}
}