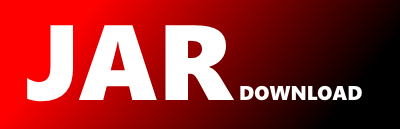
com.aliyun.ros.cdk.apig.RosRoute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ros-cdk-apig Show documentation
Show all versions of ros-cdk-apig Show documentation
Aliyun SDK Copyright (C) Alibaba Cloud Computing All rights reserved. http://www.aliyun.com
The newest version!
package com.aliyun.ros.cdk.apig;
/**
* This class is a base encapsulation around the ROS resource type ALIYUN::APIG::Route
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.85.0 (build 08ee592)", date = "2024-12-13T06:45:04.267Z")
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute")
public class RosRoute extends com.aliyun.ros.cdk.core.RosResource {
protected RosRoute(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected RosRoute(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
ROS_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(com.aliyun.ros.cdk.apig.RosRoute.class, "ROS_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
* @param enableResourcePropertyConstraint This parameter is required.
*/
public RosRoute(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.apig.RosRouteProps props, final @org.jetbrains.annotations.NotNull java.lang.Boolean enableResourcePropertyConstraint) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required"), java.util.Objects.requireNonNull(enableResourcePropertyConstraint, "enableResourcePropertyConstraint is required") });
}
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The resource type name for this resource class.
*/
public final static java.lang.String ROS_RESOURCE_TYPE_NAME;
/**
*/
public @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable getAttrBackend() {
return software.amazon.jsii.Kernel.get(this, "attrBackend", software.amazon.jsii.NativeType.forClass(com.aliyun.ros.cdk.core.IResolvable.class));
}
/**
*/
public @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable getAttrDescription() {
return software.amazon.jsii.Kernel.get(this, "attrDescription", software.amazon.jsii.NativeType.forClass(com.aliyun.ros.cdk.core.IResolvable.class));
}
/**
*/
public @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable getAttrDomainInfos() {
return software.amazon.jsii.Kernel.get(this, "attrDomainInfos", software.amazon.jsii.NativeType.forClass(com.aliyun.ros.cdk.core.IResolvable.class));
}
/**
*/
public @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable getAttrEnvironmentInfo() {
return software.amazon.jsii.Kernel.get(this, "attrEnvironmentInfo", software.amazon.jsii.NativeType.forClass(com.aliyun.ros.cdk.core.IResolvable.class));
}
/**
*/
public @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable getAttrMatch() {
return software.amazon.jsii.Kernel.get(this, "attrMatch", software.amazon.jsii.NativeType.forClass(com.aliyun.ros.cdk.core.IResolvable.class));
}
/**
*/
public @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable getAttrRouteId() {
return software.amazon.jsii.Kernel.get(this, "attrRouteId", software.amazon.jsii.NativeType.forClass(com.aliyun.ros.cdk.core.IResolvable.class));
}
/**
*/
public @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable getAttrRouteName() {
return software.amazon.jsii.Kernel.get(this, "attrRouteName", software.amazon.jsii.NativeType.forClass(com.aliyun.ros.cdk.core.IResolvable.class));
}
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map getRosProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "rosProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
*/
public @org.jetbrains.annotations.NotNull java.lang.Object getBackend() {
return software.amazon.jsii.Kernel.get(this, "backend", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setBackend(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "backend", java.util.Objects.requireNonNull(value, "backend is required"));
}
/**
*/
public void setBackend(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.apig.RosRoute.BackendProperty value) {
software.amazon.jsii.Kernel.set(this, "backend", java.util.Objects.requireNonNull(value, "backend is required"));
}
public @org.jetbrains.annotations.NotNull java.lang.Boolean getEnableResourcePropertyConstraint() {
return software.amazon.jsii.Kernel.get(this, "enableResourcePropertyConstraint", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
public void setEnableResourcePropertyConstraint(final @org.jetbrains.annotations.NotNull java.lang.Boolean value) {
software.amazon.jsii.Kernel.set(this, "enableResourcePropertyConstraint", java.util.Objects.requireNonNull(value, "enableResourcePropertyConstraint is required"));
}
/**
*/
public @org.jetbrains.annotations.NotNull java.lang.Object getEnvironmentInfo() {
return software.amazon.jsii.Kernel.get(this, "environmentInfo", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setEnvironmentInfo(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "environmentInfo", java.util.Objects.requireNonNull(value, "environmentInfo is required"));
}
/**
*/
public void setEnvironmentInfo(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.apig.RosRoute.EnvironmentInfoProperty value) {
software.amazon.jsii.Kernel.set(this, "environmentInfo", java.util.Objects.requireNonNull(value, "environmentInfo is required"));
}
/**
*/
public @org.jetbrains.annotations.NotNull java.lang.Object getHttpApiId() {
return software.amazon.jsii.Kernel.get(this, "httpApiId", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setHttpApiId(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "httpApiId", java.util.Objects.requireNonNull(value, "httpApiId is required"));
}
/**
*/
public void setHttpApiId(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "httpApiId", java.util.Objects.requireNonNull(value, "httpApiId is required"));
}
/**
*/
public @org.jetbrains.annotations.NotNull java.lang.Object getMatch() {
return software.amazon.jsii.Kernel.get(this, "match", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setMatch(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "match", java.util.Objects.requireNonNull(value, "match is required"));
}
/**
*/
public void setMatch(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.apig.RosRoute.MatchProperty value) {
software.amazon.jsii.Kernel.set(this, "match", java.util.Objects.requireNonNull(value, "match is required"));
}
/**
*/
public @org.jetbrains.annotations.NotNull java.lang.Object getRouteName() {
return software.amazon.jsii.Kernel.get(this, "routeName", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setRouteName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "routeName", java.util.Objects.requireNonNull(value, "routeName is required"));
}
/**
*/
public void setRouteName(final @org.jetbrains.annotations.NotNull com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "routeName", java.util.Objects.requireNonNull(value, "routeName is required"));
}
/**
*/
public @org.jetbrains.annotations.Nullable java.lang.Object getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
*/
public void setDescription(final @org.jetbrains.annotations.Nullable com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
*/
public @org.jetbrains.annotations.Nullable java.lang.Object getDomainIds() {
return software.amazon.jsii.Kernel.get(this, "domainIds", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setDomainIds(final @org.jetbrains.annotations.Nullable com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "domainIds", value);
}
/**
*/
public void setDomainIds(final @org.jetbrains.annotations.Nullable java.util.List value) {
if (software.amazon.jsii.Configuration.getRuntimeTypeChecking()) {
for (int __idx_ac66f0 = 0; __idx_ac66f0 < value.size(); __idx_ac66f0++) {
final java.lang.Object __val_ac66f0 = value.get(__idx_ac66f0);
if (
!(__val_ac66f0 instanceof java.lang.String)
&& !(__val_ac66f0 instanceof com.aliyun.ros.cdk.core.IResolvable)
&& !(__val_ac66f0.getClass().equals(software.amazon.jsii.JsiiObject.class))
) {
throw new IllegalArgumentException(
new java.lang.StringBuilder("Expected ")
.append("value").append(".get(").append(__idx_ac66f0).append(")")
.append(" to be one of: java.lang.String, com.aliyun.ros.cdk.core.IResolvable; received ")
.append(__val_ac66f0.getClass()).toString());
}
}
}
software.amazon.jsii.Kernel.set(this, "domainIds", value);
}
/**
*/
public @org.jetbrains.annotations.Nullable java.lang.Object getDomainInfos() {
return software.amazon.jsii.Kernel.get(this, "domainInfos", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
*/
public void setDomainInfos(final @org.jetbrains.annotations.Nullable com.aliyun.ros.cdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "domainInfos", value);
}
/**
*/
public void setDomainInfos(final @org.jetbrains.annotations.Nullable java.util.List value) {
if (software.amazon.jsii.Configuration.getRuntimeTypeChecking()) {
for (int __idx_ac66f0 = 0; __idx_ac66f0 < value.size(); __idx_ac66f0++) {
final java.lang.Object __val_ac66f0 = value.get(__idx_ac66f0);
if (
!(__val_ac66f0 instanceof com.aliyun.ros.cdk.core.IResolvable)
&& !(__val_ac66f0 instanceof com.aliyun.ros.cdk.apig.RosRoute.DomainInfosProperty)
&& !(__val_ac66f0.getClass().equals(software.amazon.jsii.JsiiObject.class))
) {
throw new IllegalArgumentException(
new java.lang.StringBuilder("Expected ")
.append("value").append(".get(").append(__idx_ac66f0).append(")")
.append(" to be one of: com.aliyun.ros.cdk.core.IResolvable, com.aliyun.ros.cdk.apig.RosRoute.DomainInfosProperty; received ")
.append(__val_ac66f0.getClass()).toString());
}
}
}
software.amazon.jsii.Kernel.set(this, "domainInfos", value);
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.BackendProperty")
@software.amazon.jsii.Jsii.Proxy(BackendProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface BackendProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getScene();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getServices();
/**
* @return a {@link Builder} of {@link BackendProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link BackendProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object scene;
java.lang.Object services;
/**
* Sets the value of {@link BackendProperty#getScene}
* @param scene the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scene(java.lang.String scene) {
this.scene = scene;
return this;
}
/**
* Sets the value of {@link BackendProperty#getScene}
* @param scene the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scene(com.aliyun.ros.cdk.core.IResolvable scene) {
this.scene = scene;
return this;
}
/**
* Sets the value of {@link BackendProperty#getServices}
* @param services the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder services(com.aliyun.ros.cdk.core.IResolvable services) {
this.services = services;
return this;
}
/**
* Sets the value of {@link BackendProperty#getServices}
* @param services the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder services(java.util.List extends java.lang.Object> services) {
this.services = services;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link BackendProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public BackendProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link BackendProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements BackendProperty {
private final java.lang.Object scene;
private final java.lang.Object services;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.scene = software.amazon.jsii.Kernel.get(this, "scene", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.services = software.amazon.jsii.Kernel.get(this, "services", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.scene = java.util.Objects.requireNonNull(builder.scene, "scene is required");
this.services = java.util.Objects.requireNonNull(builder.services, "services is required");
}
@Override
public final java.lang.Object getScene() {
return this.scene;
}
@Override
public final java.lang.Object getServices() {
return this.services;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("scene", om.valueToTree(this.getScene()));
data.set("services", om.valueToTree(this.getServices()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.BackendProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
BackendProperty.Jsii$Proxy that = (BackendProperty.Jsii$Proxy) o;
if (!scene.equals(that.scene)) return false;
return this.services.equals(that.services);
}
@Override
public final int hashCode() {
int result = this.scene.hashCode();
result = 31 * result + (this.services.hashCode());
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.DomainInfosProperty")
@software.amazon.jsii.Jsii.Proxy(DomainInfosProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface DomainInfosProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDomainId() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getName() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getProtocol() {
return null;
}
/**
* @return a {@link Builder} of {@link DomainInfosProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DomainInfosProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object domainId;
java.lang.Object name;
java.lang.Object protocol;
/**
* Sets the value of {@link DomainInfosProperty#getDomainId}
* @param domainId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainId(java.lang.String domainId) {
this.domainId = domainId;
return this;
}
/**
* Sets the value of {@link DomainInfosProperty#getDomainId}
* @param domainId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainId(com.aliyun.ros.cdk.core.IResolvable domainId) {
this.domainId = domainId;
return this;
}
/**
* Sets the value of {@link DomainInfosProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link DomainInfosProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(com.aliyun.ros.cdk.core.IResolvable name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link DomainInfosProperty#getProtocol}
* @param protocol the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder protocol(java.lang.String protocol) {
this.protocol = protocol;
return this;
}
/**
* Sets the value of {@link DomainInfosProperty#getProtocol}
* @param protocol the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder protocol(com.aliyun.ros.cdk.core.IResolvable protocol) {
this.protocol = protocol;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DomainInfosProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DomainInfosProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link DomainInfosProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DomainInfosProperty {
private final java.lang.Object domainId;
private final java.lang.Object name;
private final java.lang.Object protocol;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.domainId = software.amazon.jsii.Kernel.get(this, "domainId", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.protocol = software.amazon.jsii.Kernel.get(this, "protocol", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.domainId = builder.domainId;
this.name = builder.name;
this.protocol = builder.protocol;
}
@Override
public final java.lang.Object getDomainId() {
return this.domainId;
}
@Override
public final java.lang.Object getName() {
return this.name;
}
@Override
public final java.lang.Object getProtocol() {
return this.protocol;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDomainId() != null) {
data.set("domainId", om.valueToTree(this.getDomainId()));
}
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getProtocol() != null) {
data.set("protocol", om.valueToTree(this.getProtocol()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.DomainInfosProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DomainInfosProperty.Jsii$Proxy that = (DomainInfosProperty.Jsii$Proxy) o;
if (this.domainId != null ? !this.domainId.equals(that.domainId) : that.domainId != null) return false;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
return this.protocol != null ? this.protocol.equals(that.protocol) : that.protocol == null;
}
@Override
public final int hashCode() {
int result = this.domainId != null ? this.domainId.hashCode() : 0;
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
result = 31 * result + (this.protocol != null ? this.protocol.hashCode() : 0);
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.EnvironmentInfoProperty")
@software.amazon.jsii.Jsii.Proxy(EnvironmentInfoProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface EnvironmentInfoProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getEnvironmentId();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getAlias() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getGatewayInfo() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getName() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getSubDomains() {
return null;
}
/**
* @return a {@link Builder} of {@link EnvironmentInfoProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link EnvironmentInfoProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object environmentId;
java.lang.Object alias;
java.lang.Object gatewayInfo;
java.lang.Object name;
java.lang.Object subDomains;
/**
* Sets the value of {@link EnvironmentInfoProperty#getEnvironmentId}
* @param environmentId the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environmentId(java.lang.String environmentId) {
this.environmentId = environmentId;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getEnvironmentId}
* @param environmentId the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environmentId(com.aliyun.ros.cdk.core.IResolvable environmentId) {
this.environmentId = environmentId;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getAlias}
* @param alias the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder alias(java.lang.String alias) {
this.alias = alias;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getAlias}
* @param alias the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder alias(com.aliyun.ros.cdk.core.IResolvable alias) {
this.alias = alias;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getGatewayInfo}
* @param gatewayInfo the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gatewayInfo(com.aliyun.ros.cdk.core.IResolvable gatewayInfo) {
this.gatewayInfo = gatewayInfo;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getGatewayInfo}
* @param gatewayInfo the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gatewayInfo(com.aliyun.ros.cdk.apig.RosRoute.GatewayInfoProperty gatewayInfo) {
this.gatewayInfo = gatewayInfo;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(com.aliyun.ros.cdk.core.IResolvable name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getSubDomains}
* @param subDomains the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subDomains(com.aliyun.ros.cdk.core.IResolvable subDomains) {
this.subDomains = subDomains;
return this;
}
/**
* Sets the value of {@link EnvironmentInfoProperty#getSubDomains}
* @param subDomains the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subDomains(java.util.List extends java.lang.Object> subDomains) {
this.subDomains = subDomains;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link EnvironmentInfoProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public EnvironmentInfoProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link EnvironmentInfoProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements EnvironmentInfoProperty {
private final java.lang.Object environmentId;
private final java.lang.Object alias;
private final java.lang.Object gatewayInfo;
private final java.lang.Object name;
private final java.lang.Object subDomains;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.environmentId = software.amazon.jsii.Kernel.get(this, "environmentId", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.alias = software.amazon.jsii.Kernel.get(this, "alias", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.gatewayInfo = software.amazon.jsii.Kernel.get(this, "gatewayInfo", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.subDomains = software.amazon.jsii.Kernel.get(this, "subDomains", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.environmentId = java.util.Objects.requireNonNull(builder.environmentId, "environmentId is required");
this.alias = builder.alias;
this.gatewayInfo = builder.gatewayInfo;
this.name = builder.name;
this.subDomains = builder.subDomains;
}
@Override
public final java.lang.Object getEnvironmentId() {
return this.environmentId;
}
@Override
public final java.lang.Object getAlias() {
return this.alias;
}
@Override
public final java.lang.Object getGatewayInfo() {
return this.gatewayInfo;
}
@Override
public final java.lang.Object getName() {
return this.name;
}
@Override
public final java.lang.Object getSubDomains() {
return this.subDomains;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("environmentId", om.valueToTree(this.getEnvironmentId()));
if (this.getAlias() != null) {
data.set("alias", om.valueToTree(this.getAlias()));
}
if (this.getGatewayInfo() != null) {
data.set("gatewayInfo", om.valueToTree(this.getGatewayInfo()));
}
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getSubDomains() != null) {
data.set("subDomains", om.valueToTree(this.getSubDomains()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.EnvironmentInfoProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EnvironmentInfoProperty.Jsii$Proxy that = (EnvironmentInfoProperty.Jsii$Proxy) o;
if (!environmentId.equals(that.environmentId)) return false;
if (this.alias != null ? !this.alias.equals(that.alias) : that.alias != null) return false;
if (this.gatewayInfo != null ? !this.gatewayInfo.equals(that.gatewayInfo) : that.gatewayInfo != null) return false;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
return this.subDomains != null ? this.subDomains.equals(that.subDomains) : that.subDomains == null;
}
@Override
public final int hashCode() {
int result = this.environmentId.hashCode();
result = 31 * result + (this.alias != null ? this.alias.hashCode() : 0);
result = 31 * result + (this.gatewayInfo != null ? this.gatewayInfo.hashCode() : 0);
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
result = 31 * result + (this.subDomains != null ? this.subDomains.hashCode() : 0);
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.GatewayInfoProperty")
@software.amazon.jsii.Jsii.Proxy(GatewayInfoProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface GatewayInfoProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getGatewayId() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getName() {
return null;
}
/**
* @return a {@link Builder} of {@link GatewayInfoProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link GatewayInfoProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object gatewayId;
java.lang.Object name;
/**
* Sets the value of {@link GatewayInfoProperty#getGatewayId}
* @param gatewayId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gatewayId(java.lang.String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Sets the value of {@link GatewayInfoProperty#getGatewayId}
* @param gatewayId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gatewayId(com.aliyun.ros.cdk.core.IResolvable gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* Sets the value of {@link GatewayInfoProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link GatewayInfoProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(com.aliyun.ros.cdk.core.IResolvable name) {
this.name = name;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link GatewayInfoProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public GatewayInfoProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link GatewayInfoProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements GatewayInfoProperty {
private final java.lang.Object gatewayId;
private final java.lang.Object name;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.gatewayId = software.amazon.jsii.Kernel.get(this, "gatewayId", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.gatewayId = builder.gatewayId;
this.name = builder.name;
}
@Override
public final java.lang.Object getGatewayId() {
return this.gatewayId;
}
@Override
public final java.lang.Object getName() {
return this.name;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getGatewayId() != null) {
data.set("gatewayId", om.valueToTree(this.getGatewayId()));
}
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.GatewayInfoProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GatewayInfoProperty.Jsii$Proxy that = (GatewayInfoProperty.Jsii$Proxy) o;
if (this.gatewayId != null ? !this.gatewayId.equals(that.gatewayId) : that.gatewayId != null) return false;
return this.name != null ? this.name.equals(that.name) : that.name == null;
}
@Override
public final int hashCode() {
int result = this.gatewayId != null ? this.gatewayId.hashCode() : 0;
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.HeadersProperty")
@software.amazon.jsii.Jsii.Proxy(HeadersProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface HeadersProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getName() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getType() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getValue() {
return null;
}
/**
* @return a {@link Builder} of {@link HeadersProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link HeadersProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object name;
java.lang.Object type;
java.lang.Object value;
/**
* Sets the value of {@link HeadersProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link HeadersProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(com.aliyun.ros.cdk.core.IResolvable name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link HeadersProperty#getType}
* @param type the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link HeadersProperty#getType}
* @param type the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(com.aliyun.ros.cdk.core.IResolvable type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link HeadersProperty#getValue}
* @param value the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.String value) {
this.value = value;
return this;
}
/**
* Sets the value of {@link HeadersProperty#getValue}
* @param value the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(com.aliyun.ros.cdk.core.IResolvable value) {
this.value = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link HeadersProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public HeadersProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link HeadersProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements HeadersProperty {
private final java.lang.Object name;
private final java.lang.Object type;
private final java.lang.Object value;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = builder.name;
this.type = builder.type;
this.value = builder.value;
}
@Override
public final java.lang.Object getName() {
return this.name;
}
@Override
public final java.lang.Object getType() {
return this.type;
}
@Override
public final java.lang.Object getValue() {
return this.value;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getType() != null) {
data.set("type", om.valueToTree(this.getType()));
}
if (this.getValue() != null) {
data.set("value", om.valueToTree(this.getValue()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.HeadersProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HeadersProperty.Jsii$Proxy that = (HeadersProperty.Jsii$Proxy) o;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
if (this.type != null ? !this.type.equals(that.type) : that.type != null) return false;
return this.value != null ? this.value.equals(that.value) : that.value == null;
}
@Override
public final int hashCode() {
int result = this.name != null ? this.name.hashCode() : 0;
result = 31 * result + (this.type != null ? this.type.hashCode() : 0);
result = 31 * result + (this.value != null ? this.value.hashCode() : 0);
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.MatchProperty")
@software.amazon.jsii.Jsii.Proxy(MatchProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface MatchProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getPath();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getHeaders() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getIgnoreUriCase() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getMethods() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getQueryParams() {
return null;
}
/**
* @return a {@link Builder} of {@link MatchProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link MatchProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object path;
java.lang.Object headers;
java.lang.Object ignoreUriCase;
java.lang.Object methods;
java.lang.Object queryParams;
/**
* Sets the value of {@link MatchProperty#getPath}
* @param path the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder path(com.aliyun.ros.cdk.core.IResolvable path) {
this.path = path;
return this;
}
/**
* Sets the value of {@link MatchProperty#getPath}
* @param path the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder path(com.aliyun.ros.cdk.apig.RosRoute.PathProperty path) {
this.path = path;
return this;
}
/**
* Sets the value of {@link MatchProperty#getHeaders}
* @param headers the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder headers(com.aliyun.ros.cdk.core.IResolvable headers) {
this.headers = headers;
return this;
}
/**
* Sets the value of {@link MatchProperty#getHeaders}
* @param headers the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder headers(java.util.List extends java.lang.Object> headers) {
this.headers = headers;
return this;
}
/**
* Sets the value of {@link MatchProperty#getIgnoreUriCase}
* @param ignoreUriCase the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ignoreUriCase(java.lang.Boolean ignoreUriCase) {
this.ignoreUriCase = ignoreUriCase;
return this;
}
/**
* Sets the value of {@link MatchProperty#getIgnoreUriCase}
* @param ignoreUriCase the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ignoreUriCase(com.aliyun.ros.cdk.core.IResolvable ignoreUriCase) {
this.ignoreUriCase = ignoreUriCase;
return this;
}
/**
* Sets the value of {@link MatchProperty#getMethods}
* @param methods the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder methods(com.aliyun.ros.cdk.core.IResolvable methods) {
this.methods = methods;
return this;
}
/**
* Sets the value of {@link MatchProperty#getMethods}
* @param methods the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder methods(java.util.List extends java.lang.Object> methods) {
this.methods = methods;
return this;
}
/**
* Sets the value of {@link MatchProperty#getQueryParams}
* @param queryParams the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder queryParams(com.aliyun.ros.cdk.core.IResolvable queryParams) {
this.queryParams = queryParams;
return this;
}
/**
* Sets the value of {@link MatchProperty#getQueryParams}
* @param queryParams the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder queryParams(java.util.List extends java.lang.Object> queryParams) {
this.queryParams = queryParams;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link MatchProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public MatchProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link MatchProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements MatchProperty {
private final java.lang.Object path;
private final java.lang.Object headers;
private final java.lang.Object ignoreUriCase;
private final java.lang.Object methods;
private final java.lang.Object queryParams;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.path = software.amazon.jsii.Kernel.get(this, "path", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.headers = software.amazon.jsii.Kernel.get(this, "headers", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.ignoreUriCase = software.amazon.jsii.Kernel.get(this, "ignoreUriCase", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.methods = software.amazon.jsii.Kernel.get(this, "methods", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.queryParams = software.amazon.jsii.Kernel.get(this, "queryParams", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.path = java.util.Objects.requireNonNull(builder.path, "path is required");
this.headers = builder.headers;
this.ignoreUriCase = builder.ignoreUriCase;
this.methods = builder.methods;
this.queryParams = builder.queryParams;
}
@Override
public final java.lang.Object getPath() {
return this.path;
}
@Override
public final java.lang.Object getHeaders() {
return this.headers;
}
@Override
public final java.lang.Object getIgnoreUriCase() {
return this.ignoreUriCase;
}
@Override
public final java.lang.Object getMethods() {
return this.methods;
}
@Override
public final java.lang.Object getQueryParams() {
return this.queryParams;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("path", om.valueToTree(this.getPath()));
if (this.getHeaders() != null) {
data.set("headers", om.valueToTree(this.getHeaders()));
}
if (this.getIgnoreUriCase() != null) {
data.set("ignoreUriCase", om.valueToTree(this.getIgnoreUriCase()));
}
if (this.getMethods() != null) {
data.set("methods", om.valueToTree(this.getMethods()));
}
if (this.getQueryParams() != null) {
data.set("queryParams", om.valueToTree(this.getQueryParams()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.MatchProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MatchProperty.Jsii$Proxy that = (MatchProperty.Jsii$Proxy) o;
if (!path.equals(that.path)) return false;
if (this.headers != null ? !this.headers.equals(that.headers) : that.headers != null) return false;
if (this.ignoreUriCase != null ? !this.ignoreUriCase.equals(that.ignoreUriCase) : that.ignoreUriCase != null) return false;
if (this.methods != null ? !this.methods.equals(that.methods) : that.methods != null) return false;
return this.queryParams != null ? this.queryParams.equals(that.queryParams) : that.queryParams == null;
}
@Override
public final int hashCode() {
int result = this.path.hashCode();
result = 31 * result + (this.headers != null ? this.headers.hashCode() : 0);
result = 31 * result + (this.ignoreUriCase != null ? this.ignoreUriCase.hashCode() : 0);
result = 31 * result + (this.methods != null ? this.methods.hashCode() : 0);
result = 31 * result + (this.queryParams != null ? this.queryParams.hashCode() : 0);
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.PathProperty")
@software.amazon.jsii.Jsii.Proxy(PathProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface PathProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getType();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getValue();
/**
* @return a {@link Builder} of {@link PathProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PathProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object type;
java.lang.Object value;
/**
* Sets the value of {@link PathProperty#getType}
* @param type the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link PathProperty#getType}
* @param type the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(com.aliyun.ros.cdk.core.IResolvable type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link PathProperty#getValue}
* @param value the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.String value) {
this.value = value;
return this;
}
/**
* Sets the value of {@link PathProperty#getValue}
* @param value the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(com.aliyun.ros.cdk.core.IResolvable value) {
this.value = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PathProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public PathProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link PathProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PathProperty {
private final java.lang.Object type;
private final java.lang.Object value;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.type = java.util.Objects.requireNonNull(builder.type, "type is required");
this.value = java.util.Objects.requireNonNull(builder.value, "value is required");
}
@Override
public final java.lang.Object getType() {
return this.type;
}
@Override
public final java.lang.Object getValue() {
return this.value;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("type", om.valueToTree(this.getType()));
data.set("value", om.valueToTree(this.getValue()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.PathProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PathProperty.Jsii$Proxy that = (PathProperty.Jsii$Proxy) o;
if (!type.equals(that.type)) return false;
return this.value.equals(that.value);
}
@Override
public final int hashCode() {
int result = this.type.hashCode();
result = 31 * result + (this.value.hashCode());
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.QueryParamsProperty")
@software.amazon.jsii.Jsii.Proxy(QueryParamsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface QueryParamsProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getName() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getType() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getValue() {
return null;
}
/**
* @return a {@link Builder} of {@link QueryParamsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link QueryParamsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object name;
java.lang.Object type;
java.lang.Object value;
/**
* Sets the value of {@link QueryParamsProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link QueryParamsProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(com.aliyun.ros.cdk.core.IResolvable name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link QueryParamsProperty#getType}
* @param type the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link QueryParamsProperty#getType}
* @param type the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(com.aliyun.ros.cdk.core.IResolvable type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link QueryParamsProperty#getValue}
* @param value the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.String value) {
this.value = value;
return this;
}
/**
* Sets the value of {@link QueryParamsProperty#getValue}
* @param value the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(com.aliyun.ros.cdk.core.IResolvable value) {
this.value = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link QueryParamsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public QueryParamsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link QueryParamsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements QueryParamsProperty {
private final java.lang.Object name;
private final java.lang.Object type;
private final java.lang.Object value;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = builder.name;
this.type = builder.type;
this.value = builder.value;
}
@Override
public final java.lang.Object getName() {
return this.name;
}
@Override
public final java.lang.Object getType() {
return this.type;
}
@Override
public final java.lang.Object getValue() {
return this.value;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getType() != null) {
data.set("type", om.valueToTree(this.getType()));
}
if (this.getValue() != null) {
data.set("value", om.valueToTree(this.getValue()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.QueryParamsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
QueryParamsProperty.Jsii$Proxy that = (QueryParamsProperty.Jsii$Proxy) o;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
if (this.type != null ? !this.type.equals(that.type) : that.type != null) return false;
return this.value != null ? this.value.equals(that.value) : that.value == null;
}
@Override
public final int hashCode() {
int result = this.name != null ? this.name.hashCode() : 0;
result = 31 * result + (this.type != null ? this.type.hashCode() : 0);
result = 31 * result + (this.value != null ? this.value.hashCode() : 0);
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.ServicesProperty")
@software.amazon.jsii.Jsii.Proxy(ServicesProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ServicesProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getName() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getPort() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getProtocol() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getServiceId() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getVersion() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getWeight() {
return null;
}
/**
* @return a {@link Builder} of {@link ServicesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ServicesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object name;
java.lang.Object port;
java.lang.Object protocol;
java.lang.Object serviceId;
java.lang.Object version;
java.lang.Object weight;
/**
* Sets the value of {@link ServicesProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(com.aliyun.ros.cdk.core.IResolvable name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getPort}
* @param port the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(java.lang.Number port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getPort}
* @param port the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(com.aliyun.ros.cdk.core.IResolvable port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getProtocol}
* @param protocol the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder protocol(java.lang.String protocol) {
this.protocol = protocol;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getProtocol}
* @param protocol the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder protocol(com.aliyun.ros.cdk.core.IResolvable protocol) {
this.protocol = protocol;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getServiceId}
* @param serviceId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceId(java.lang.String serviceId) {
this.serviceId = serviceId;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getServiceId}
* @param serviceId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceId(com.aliyun.ros.cdk.core.IResolvable serviceId) {
this.serviceId = serviceId;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getVersion}
* @param version the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder version(java.lang.String version) {
this.version = version;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getVersion}
* @param version the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder version(com.aliyun.ros.cdk.core.IResolvable version) {
this.version = version;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getWeight}
* @param weight the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder weight(java.lang.Number weight) {
this.weight = weight;
return this;
}
/**
* Sets the value of {@link ServicesProperty#getWeight}
* @param weight the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder weight(com.aliyun.ros.cdk.core.IResolvable weight) {
this.weight = weight;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ServicesProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ServicesProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ServicesProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ServicesProperty {
private final java.lang.Object name;
private final java.lang.Object port;
private final java.lang.Object protocol;
private final java.lang.Object serviceId;
private final java.lang.Object version;
private final java.lang.Object weight;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.port = software.amazon.jsii.Kernel.get(this, "port", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.protocol = software.amazon.jsii.Kernel.get(this, "protocol", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.serviceId = software.amazon.jsii.Kernel.get(this, "serviceId", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.version = software.amazon.jsii.Kernel.get(this, "version", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.weight = software.amazon.jsii.Kernel.get(this, "weight", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = builder.name;
this.port = builder.port;
this.protocol = builder.protocol;
this.serviceId = builder.serviceId;
this.version = builder.version;
this.weight = builder.weight;
}
@Override
public final java.lang.Object getName() {
return this.name;
}
@Override
public final java.lang.Object getPort() {
return this.port;
}
@Override
public final java.lang.Object getProtocol() {
return this.protocol;
}
@Override
public final java.lang.Object getServiceId() {
return this.serviceId;
}
@Override
public final java.lang.Object getVersion() {
return this.version;
}
@Override
public final java.lang.Object getWeight() {
return this.weight;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getPort() != null) {
data.set("port", om.valueToTree(this.getPort()));
}
if (this.getProtocol() != null) {
data.set("protocol", om.valueToTree(this.getProtocol()));
}
if (this.getServiceId() != null) {
data.set("serviceId", om.valueToTree(this.getServiceId()));
}
if (this.getVersion() != null) {
data.set("version", om.valueToTree(this.getVersion()));
}
if (this.getWeight() != null) {
data.set("weight", om.valueToTree(this.getWeight()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.ServicesProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ServicesProperty.Jsii$Proxy that = (ServicesProperty.Jsii$Proxy) o;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
if (this.port != null ? !this.port.equals(that.port) : that.port != null) return false;
if (this.protocol != null ? !this.protocol.equals(that.protocol) : that.protocol != null) return false;
if (this.serviceId != null ? !this.serviceId.equals(that.serviceId) : that.serviceId != null) return false;
if (this.version != null ? !this.version.equals(that.version) : that.version != null) return false;
return this.weight != null ? this.weight.equals(that.weight) : that.weight == null;
}
@Override
public final int hashCode() {
int result = this.name != null ? this.name.hashCode() : 0;
result = 31 * result + (this.port != null ? this.port.hashCode() : 0);
result = 31 * result + (this.protocol != null ? this.protocol.hashCode() : 0);
result = 31 * result + (this.serviceId != null ? this.serviceId.hashCode() : 0);
result = 31 * result + (this.version != null ? this.version.hashCode() : 0);
result = 31 * result + (this.weight != null ? this.weight.hashCode() : 0);
return result;
}
}
}
/**
*/
@software.amazon.jsii.Jsii(module = com.aliyun.ros.cdk.apig.$Module.class, fqn = "@alicloud/ros-cdk-apig.RosRoute.SubDomainsProperty")
@software.amazon.jsii.Jsii.Proxy(SubDomainsProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface SubDomainsProperty extends software.amazon.jsii.JsiiSerializable {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDomainId() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getName() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getNetworkType() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getProtocol() {
return null;
}
/**
* @return a {@link Builder} of {@link SubDomainsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link SubDomainsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object domainId;
java.lang.Object name;
java.lang.Object networkType;
java.lang.Object protocol;
/**
* Sets the value of {@link SubDomainsProperty#getDomainId}
* @param domainId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainId(java.lang.String domainId) {
this.domainId = domainId;
return this;
}
/**
* Sets the value of {@link SubDomainsProperty#getDomainId}
* @param domainId the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainId(com.aliyun.ros.cdk.core.IResolvable domainId) {
this.domainId = domainId;
return this;
}
/**
* Sets the value of {@link SubDomainsProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link SubDomainsProperty#getName}
* @param name the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(com.aliyun.ros.cdk.core.IResolvable name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link SubDomainsProperty#getNetworkType}
* @param networkType the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder networkType(java.lang.String networkType) {
this.networkType = networkType;
return this;
}
/**
* Sets the value of {@link SubDomainsProperty#getNetworkType}
* @param networkType the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder networkType(com.aliyun.ros.cdk.core.IResolvable networkType) {
this.networkType = networkType;
return this;
}
/**
* Sets the value of {@link SubDomainsProperty#getProtocol}
* @param protocol the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder protocol(java.lang.String protocol) {
this.protocol = protocol;
return this;
}
/**
* Sets the value of {@link SubDomainsProperty#getProtocol}
* @param protocol the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder protocol(com.aliyun.ros.cdk.core.IResolvable protocol) {
this.protocol = protocol;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link SubDomainsProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public SubDomainsProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link SubDomainsProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements SubDomainsProperty {
private final java.lang.Object domainId;
private final java.lang.Object name;
private final java.lang.Object networkType;
private final java.lang.Object protocol;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.domainId = software.amazon.jsii.Kernel.get(this, "domainId", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.networkType = software.amazon.jsii.Kernel.get(this, "networkType", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.protocol = software.amazon.jsii.Kernel.get(this, "protocol", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.domainId = builder.domainId;
this.name = builder.name;
this.networkType = builder.networkType;
this.protocol = builder.protocol;
}
@Override
public final java.lang.Object getDomainId() {
return this.domainId;
}
@Override
public final java.lang.Object getName() {
return this.name;
}
@Override
public final java.lang.Object getNetworkType() {
return this.networkType;
}
@Override
public final java.lang.Object getProtocol() {
return this.protocol;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDomainId() != null) {
data.set("domainId", om.valueToTree(this.getDomainId()));
}
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getNetworkType() != null) {
data.set("networkType", om.valueToTree(this.getNetworkType()));
}
if (this.getProtocol() != null) {
data.set("protocol", om.valueToTree(this.getProtocol()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@alicloud/ros-cdk-apig.RosRoute.SubDomainsProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SubDomainsProperty.Jsii$Proxy that = (SubDomainsProperty.Jsii$Proxy) o;
if (this.domainId != null ? !this.domainId.equals(that.domainId) : that.domainId != null) return false;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
if (this.networkType != null ? !this.networkType.equals(that.networkType) : that.networkType != null) return false;
return this.protocol != null ? this.protocol.equals(that.protocol) : that.protocol == null;
}
@Override
public final int hashCode() {
int result = this.domainId != null ? this.domainId.hashCode() : 0;
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
result = 31 * result + (this.networkType != null ? this.networkType.hashCode() : 0);
result = 31 * result + (this.protocol != null ? this.protocol.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link com.aliyun.ros.cdk.apig.RosRoute}.
*/
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param enableResourcePropertyConstraint This parameter is required.
*/
public static Builder create(final com.aliyun.ros.cdk.core.Construct scope, final java.lang.String id, final java.lang.Boolean enableResourcePropertyConstraint) {
return new Builder(scope, id, enableResourcePropertyConstraint);
}
private final com.aliyun.ros.cdk.core.Construct scope;
private final java.lang.String id;
private final java.lang.Boolean enableResourcePropertyConstraint;
private final com.aliyun.ros.cdk.apig.RosRouteProps.Builder props;
private Builder(final com.aliyun.ros.cdk.core.Construct scope, final java.lang.String id, final java.lang.Boolean enableResourcePropertyConstraint) {
this.scope = scope;
this.id = id;
this.enableResourcePropertyConstraint = enableResourcePropertyConstraint;
this.props = new com.aliyun.ros.cdk.apig.RosRouteProps.Builder();
}
/**
* @return {@code this}
* @param backend This parameter is required.
*/
public Builder backend(final com.aliyun.ros.cdk.core.IResolvable backend) {
this.props.backend(backend);
return this;
}
/**
* @return {@code this}
* @param backend This parameter is required.
*/
public Builder backend(final com.aliyun.ros.cdk.apig.RosRoute.BackendProperty backend) {
this.props.backend(backend);
return this;
}
/**
* @return {@code this}
* @param environmentInfo This parameter is required.
*/
public Builder environmentInfo(final com.aliyun.ros.cdk.core.IResolvable environmentInfo) {
this.props.environmentInfo(environmentInfo);
return this;
}
/**
* @return {@code this}
* @param environmentInfo This parameter is required.
*/
public Builder environmentInfo(final com.aliyun.ros.cdk.apig.RosRoute.EnvironmentInfoProperty environmentInfo) {
this.props.environmentInfo(environmentInfo);
return this;
}
/**
* @return {@code this}
* @param httpApiId This parameter is required.
*/
public Builder httpApiId(final java.lang.String httpApiId) {
this.props.httpApiId(httpApiId);
return this;
}
/**
* @return {@code this}
* @param httpApiId This parameter is required.
*/
public Builder httpApiId(final com.aliyun.ros.cdk.core.IResolvable httpApiId) {
this.props.httpApiId(httpApiId);
return this;
}
/**
* @return {@code this}
* @param match This parameter is required.
*/
public Builder match(final com.aliyun.ros.cdk.core.IResolvable match) {
this.props.match(match);
return this;
}
/**
* @return {@code this}
* @param match This parameter is required.
*/
public Builder match(final com.aliyun.ros.cdk.apig.RosRoute.MatchProperty match) {
this.props.match(match);
return this;
}
/**
* @return {@code this}
* @param routeName This parameter is required.
*/
public Builder routeName(final java.lang.String routeName) {
this.props.routeName(routeName);
return this;
}
/**
* @return {@code this}
* @param routeName This parameter is required.
*/
public Builder routeName(final com.aliyun.ros.cdk.core.IResolvable routeName) {
this.props.routeName(routeName);
return this;
}
/**
* @return {@code this}
* @param description This parameter is required.
*/
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* @return {@code this}
* @param description This parameter is required.
*/
public Builder description(final com.aliyun.ros.cdk.core.IResolvable description) {
this.props.description(description);
return this;
}
/**
* @return {@code this}
* @param domainIds This parameter is required.
*/
public Builder domainIds(final com.aliyun.ros.cdk.core.IResolvable domainIds) {
this.props.domainIds(domainIds);
return this;
}
/**
* @return {@code this}
* @param domainIds This parameter is required.
*/
public Builder domainIds(final java.util.List extends java.lang.Object> domainIds) {
this.props.domainIds(domainIds);
return this;
}
/**
* @return {@code this}
* @param domainInfos This parameter is required.
*/
public Builder domainInfos(final com.aliyun.ros.cdk.core.IResolvable domainInfos) {
this.props.domainInfos(domainInfos);
return this;
}
/**
* @return {@code this}
* @param domainInfos This parameter is required.
*/
public Builder domainInfos(final java.util.List extends java.lang.Object> domainInfos) {
this.props.domainInfos(domainInfos);
return this;
}
/**
* @return a newly built instance of {@link com.aliyun.ros.cdk.apig.RosRoute}.
*/
@Override
public com.aliyun.ros.cdk.apig.RosRoute build() {
return new com.aliyun.ros.cdk.apig.RosRoute(
this.scope,
this.id,
this.props.build(),
this.enableResourcePropertyConstraint
);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy