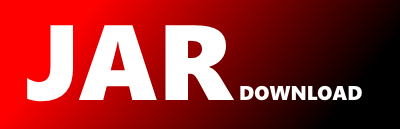
com.aliyun.ros20190910.models.GetStackResourceResponseBody Maven / Gradle / Ivy
Show all versions of ros20190910 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ros20190910.models;
import com.aliyun.tea.*;
public class GetStackResourceResponseBody extends TeaModel {
/**
* The resource type.
*
* example:
* 2019-08-01T06:01:23
*/
@NameInMap("CreateTime")
public String createTime;
/**
* The reason why the resource is in its current state.
*
* example:
* no description
*/
@NameInMap("Description")
public String description;
/**
* The ID of the stack.
*
* example:
* 2020-02-27T07:47:47
*/
@NameInMap("DriftDetectionTime")
public String driftDetectionTime;
/**
* The time when the resource was updated.
* The time follows the ISO 8601 standard in the YYYY-MM-DDThh:mm:ss format. The time is displayed in UTC.
*
* example:
* WebServer
*/
@NameInMap("LogicalResourceId")
public String logicalResourceId;
/**
* The list of the resource properties.
*
* example:
* {"key": "value"}
*/
@NameInMap("Metadata")
public java.util.Map metadata;
/**
* The information about the modules from which the resource is created. This parameter is returned only if the resource is created from modules.
*/
@NameInMap("ModuleInfo")
public GetStackResourceResponseBodyModuleInfo moduleInfo;
/**
* The metadata.
*
* example:
* d04af923-e6b7-4272-aeaa-47ec9777****
*/
@NameInMap("PhysicalResourceId")
public String physicalResourceId;
/**
* The physical ID of the resource.
*
* example:
* B288A0BE-D927-4888-B0F7-B35EF84B6E6
*/
@NameInMap("RequestId")
public String requestId;
/**
* The status of the resource in the last successful drift detection. Valid values:
*
* - DELETED: The actual configuration of the resource differs from its expected template configuration because the resource is deleted.
* - MODIFIED: The actual configuration of the resource differs from its expected template configuration.
* - NOT_CHECKED: ROS has not checked whether the actual configuration of the resource differs from its expected template configuration.
* - IN_SYNC: The actual configuration of the resource matches its expected template configuration.
*
*/
@NameInMap("ResourceAttributes")
public java.util.List> resourceAttributes;
/**
* The time when the last successful drift detection was performed on the stack.
*
* example:
* IN_SYNC
*/
@NameInMap("ResourceDriftStatus")
public String resourceDriftStatus;
/**
* The logical ID of the resource defined in the template.
*
* example:
* ALIYUN::ROS::WaitConditionHandle
*/
@NameInMap("ResourceType")
public String resourceType;
/**
* The ID of the stack.
*
* example:
* efdf5c10-96a5-4fd7-ab89-68e7baa2****
*/
@NameInMap("StackId")
public String stackId;
/**
* The name of the stack.
*
* example:
* test-describe-resource
*/
@NameInMap("StackName")
public String stackName;
/**
* The ID of the request.
*
* example:
* CREATE_COMPLETE
*/
@NameInMap("Status")
public String status;
/**
* The time when the resource was created.
* The time follows the ISO 8601 standard in the YYYY-MM-DDThh:mm:ss format. The time is displayed in UTC.
*
* example:
* state changed
*/
@NameInMap("StatusReason")
public String statusReason;
/**
* The name of the stack.
* The name can be up to 255 characters in length, and can contain digits, letters, hyphens (-), and underscores (_). The name must start with a digit or letter.
*
* example:
* 2019-08-01T06:01:29
*/
@NameInMap("UpdateTime")
public String updateTime;
public static GetStackResourceResponseBody build(java.util.Map map) throws Exception {
GetStackResourceResponseBody self = new GetStackResourceResponseBody();
return TeaModel.build(map, self);
}
public GetStackResourceResponseBody setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
public String getCreateTime() {
return this.createTime;
}
public GetStackResourceResponseBody setDescription(String description) {
this.description = description;
return this;
}
public String getDescription() {
return this.description;
}
public GetStackResourceResponseBody setDriftDetectionTime(String driftDetectionTime) {
this.driftDetectionTime = driftDetectionTime;
return this;
}
public String getDriftDetectionTime() {
return this.driftDetectionTime;
}
public GetStackResourceResponseBody setLogicalResourceId(String logicalResourceId) {
this.logicalResourceId = logicalResourceId;
return this;
}
public String getLogicalResourceId() {
return this.logicalResourceId;
}
public GetStackResourceResponseBody setMetadata(java.util.Map metadata) {
this.metadata = metadata;
return this;
}
public java.util.Map getMetadata() {
return this.metadata;
}
public GetStackResourceResponseBody setModuleInfo(GetStackResourceResponseBodyModuleInfo moduleInfo) {
this.moduleInfo = moduleInfo;
return this;
}
public GetStackResourceResponseBodyModuleInfo getModuleInfo() {
return this.moduleInfo;
}
public GetStackResourceResponseBody setPhysicalResourceId(String physicalResourceId) {
this.physicalResourceId = physicalResourceId;
return this;
}
public String getPhysicalResourceId() {
return this.physicalResourceId;
}
public GetStackResourceResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public GetStackResourceResponseBody setResourceAttributes(java.util.List> resourceAttributes) {
this.resourceAttributes = resourceAttributes;
return this;
}
public java.util.List> getResourceAttributes() {
return this.resourceAttributes;
}
public GetStackResourceResponseBody setResourceDriftStatus(String resourceDriftStatus) {
this.resourceDriftStatus = resourceDriftStatus;
return this;
}
public String getResourceDriftStatus() {
return this.resourceDriftStatus;
}
public GetStackResourceResponseBody setResourceType(String resourceType) {
this.resourceType = resourceType;
return this;
}
public String getResourceType() {
return this.resourceType;
}
public GetStackResourceResponseBody setStackId(String stackId) {
this.stackId = stackId;
return this;
}
public String getStackId() {
return this.stackId;
}
public GetStackResourceResponseBody setStackName(String stackName) {
this.stackName = stackName;
return this;
}
public String getStackName() {
return this.stackName;
}
public GetStackResourceResponseBody setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
public GetStackResourceResponseBody setStatusReason(String statusReason) {
this.statusReason = statusReason;
return this;
}
public String getStatusReason() {
return this.statusReason;
}
public GetStackResourceResponseBody setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
public String getUpdateTime() {
return this.updateTime;
}
public static class GetStackResourceResponseBodyModuleInfo extends TeaModel {
/**
* The concatenated logical IDs of one or more modules that contain the resource. The modules are listed from the outermost layer and separated by forward slashes (/
).
* In the following example, the resource is created from Module B nested within Parent Module A:
* moduleA/moduleB
*
* example:
* moduleA/moduleB
*/
@NameInMap("LogicalIdHierarchy")
public String logicalIdHierarchy;
/**
* The concatenated types of one or more modules that contain the resource. The module types are listed from the outermost layer and separated by forward slashes (/
).
* In the following example, the resource is created from a module of the MODULE::ROS::Child::Example
type that is nested within a parent module of the MODULE::ROS::Parent::Example
type:
* MODULE::ROS::Parent::Example/MODULE::ROS::Child::Example
*
* example:
* MODULE::ROS::Parent::Example/MODULE::ROS::Child::Example
*/
@NameInMap("TypeHierarchy")
public String typeHierarchy;
public static GetStackResourceResponseBodyModuleInfo build(java.util.Map map) throws Exception {
GetStackResourceResponseBodyModuleInfo self = new GetStackResourceResponseBodyModuleInfo();
return TeaModel.build(map, self);
}
public GetStackResourceResponseBodyModuleInfo setLogicalIdHierarchy(String logicalIdHierarchy) {
this.logicalIdHierarchy = logicalIdHierarchy;
return this;
}
public String getLogicalIdHierarchy() {
return this.logicalIdHierarchy;
}
public GetStackResourceResponseBodyModuleInfo setTypeHierarchy(String typeHierarchy) {
this.typeHierarchy = typeHierarchy;
return this;
}
public String getTypeHierarchy() {
return this.typeHierarchy;
}
}
}