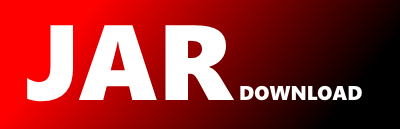
com.aliyun.ros20190910.models.ListChangeSetsRequest Maven / Gradle / Ivy
Show all versions of ros20190910 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ros20190910.models;
import com.aliyun.tea.*;
public class ListChangeSetsRequest extends TeaModel {
/**
* The ID of the change set. If detailed information about the change set is not required, you can use this parameter to replace the GetChangeSet operation.
*
* example:
* 1f6521a4-05af-4975-afe9-bc4b45ad****
*/
@NameInMap("ChangeSetId")
public String changeSetId;
/**
* The name of change set N. Maximum value of N: 5. You can use an asterisk (\*) as a wildcard for fuzzy search.
*
* example:
* MyChangeSet
*/
@NameInMap("ChangeSetName")
public java.util.List changeSetName;
/**
* The execution status of change set N. Maximum value of N: 5. Valid values:
*
* - UNAVAILABLE
* - AVAILABLE
* - EXECUTE_IN_PROGRESS
* - EXECUTE_COMPLETE
* - EXECUTE_FAILED
* - OBSOLETE
*
*
* example:
* AVAILABLE
*/
@NameInMap("ExecutionStatus")
public java.util.List executionStatus;
/**
* The page number.\
* Pages start from page 1.\
* Default value: 1.
*
* example:
* 1
*/
@NameInMap("PageNumber")
public Long pageNumber;
/**
* The number of entries per page.\
* Valid values: 1 to 50.\
* Default value: 10.
*
* example:
* 10
*/
@NameInMap("PageSize")
public Long pageSize;
/**
* The region ID of the change set. You can call the DescribeRegions operation to query the most recent region list.
* This parameter is required.
*
* example:
* cn-hangzhou
*/
@NameInMap("RegionId")
public String regionId;
/**
* The ID of the stack.
* This parameter is required.
*
* example:
* 4a6c9851-3b0f-4f5f-b4ca-a14bf691****
*/
@NameInMap("StackId")
public String stackId;
/**
* The status of change set N. Maximum value of N: 5. Valid values:
*
* - CREATE_PENDING
* - CREATE_IN_PROGRESS
* - CREATE_COMPLETE
* - CREATE_FAILED
* - DELETE_FAILED
* - DELETE_COMPLETE
*
*
* example:
* CREATE_COMPLETE
*/
@NameInMap("Status")
public java.util.List status;
public static ListChangeSetsRequest build(java.util.Map map) throws Exception {
ListChangeSetsRequest self = new ListChangeSetsRequest();
return TeaModel.build(map, self);
}
public ListChangeSetsRequest setChangeSetId(String changeSetId) {
this.changeSetId = changeSetId;
return this;
}
public String getChangeSetId() {
return this.changeSetId;
}
public ListChangeSetsRequest setChangeSetName(java.util.List changeSetName) {
this.changeSetName = changeSetName;
return this;
}
public java.util.List getChangeSetName() {
return this.changeSetName;
}
public ListChangeSetsRequest setExecutionStatus(java.util.List executionStatus) {
this.executionStatus = executionStatus;
return this;
}
public java.util.List getExecutionStatus() {
return this.executionStatus;
}
public ListChangeSetsRequest setPageNumber(Long pageNumber) {
this.pageNumber = pageNumber;
return this;
}
public Long getPageNumber() {
return this.pageNumber;
}
public ListChangeSetsRequest setPageSize(Long pageSize) {
this.pageSize = pageSize;
return this;
}
public Long getPageSize() {
return this.pageSize;
}
public ListChangeSetsRequest setRegionId(String regionId) {
this.regionId = regionId;
return this;
}
public String getRegionId() {
return this.regionId;
}
public ListChangeSetsRequest setStackId(String stackId) {
this.stackId = stackId;
return this;
}
public String getStackId() {
return this.stackId;
}
public ListChangeSetsRequest setStatus(java.util.List status) {
this.status = status;
return this;
}
public java.util.List getStatus() {
return this.status;
}
}