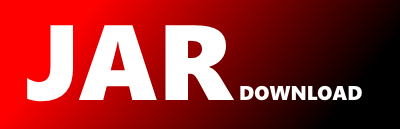
com.aliyun.sas20181203.models.CreateUniBackupPolicyRequest Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class CreateUniBackupPolicyRequest extends TeaModel {
/**
* The name of the database account.
*
* example:
* admin
*/
@NameInMap("AccountName")
public String accountName;
/**
* The password of the database account.
*
* example:
* Pass****
*/
@NameInMap("AccountPassword")
public String accountPassword;
/**
* Specifies whether the database is manually added. Valid values:
*
* - true: yes
* - false: no
*
*
* example:
* true
*/
@NameInMap("DatabaseAddByUser")
public String databaseAddByUser;
/**
* The type of the database. Valid values:
*
* - MYSQL
* - ORACLE
* - MSSQL
*
* This parameter is required.
*
* example:
* MYSQL
*/
@NameInMap("DatabaseType")
public String databaseType;
/**
* The policy for full data backup. The value of this parameter is a JSON string. The JSON string contains the following fields:
*
* - start: the start time of a backup task.
* - interval: the interval of backup tasks.
* - type: the unit of the interval.
* - days: the days of a week on which a backup task is performed.
*
* This parameter is required.
*
* example:
* {"days":[4],"interval":1,"planType":"weekly","startTime":"22:00:00"}
*/
@NameInMap("FullPlan")
public java.util.Map fullPlan;
/**
* The policy for incremental data backup. The value of this parameter is a JSON string. The JSON string contains the following fields:
*
* - start: the start time of a backup task.
* - interval: the interval of backup tasks.
* - type: the unit of the interval.
* - days: the days of a week on which a backup task is performed.
*
* This parameter is required.
*
* example:
* {"interval":1,"planType":"daily","startTime":"23:30:00"}
*/
@NameInMap("IncPlan")
public java.util.Map incPlan;
/**
* The ID of the Elastic Compute Service (ECS) instance.
*
* You can call the DescribeUniBackupDatabase operation to query the IDs of ECS instances.
*
* This parameter is required.
*
* example:
* i-bp1fu4aqltf1huhc****
*/
@NameInMap("InstanceId")
public String instanceId;
/**
* The name of the anti-ransomware policy.
* This parameter is required.
*
* example:
* mysql-policy
*/
@NameInMap("PolicyName")
public String policyName;
/**
* The retention period of backup data.
* This parameter is required.
*
* example:
* 7
*/
@NameInMap("Retention")
public Integer retention;
/**
* The maximum network bandwidth that is allowed during data backup. Unit: bytes.
* This parameter is required.
*
* example:
* 5242880
*/
@NameInMap("SpeedLimiter")
public Long speedLimiter;
/**
* The region in which the server resides.
* This parameter is required.
*
* example:
* cn-hangzhou
*/
@NameInMap("UniRegionId")
public String uniRegionId;
/**
* The UUID of the server whose data is backed up based on the anti-ransomware policy.
*
* You can call the DescribeCloudCenterInstances operation to query the UUIDs of servers.
*
*
* example:
* 045cad48-eb08-4047-a70c-713aec7b****
*/
@NameInMap("Uuid")
public String uuid;
public static CreateUniBackupPolicyRequest build(java.util.Map map) throws Exception {
CreateUniBackupPolicyRequest self = new CreateUniBackupPolicyRequest();
return TeaModel.build(map, self);
}
public CreateUniBackupPolicyRequest setAccountName(String accountName) {
this.accountName = accountName;
return this;
}
public String getAccountName() {
return this.accountName;
}
public CreateUniBackupPolicyRequest setAccountPassword(String accountPassword) {
this.accountPassword = accountPassword;
return this;
}
public String getAccountPassword() {
return this.accountPassword;
}
public CreateUniBackupPolicyRequest setDatabaseAddByUser(String databaseAddByUser) {
this.databaseAddByUser = databaseAddByUser;
return this;
}
public String getDatabaseAddByUser() {
return this.databaseAddByUser;
}
public CreateUniBackupPolicyRequest setDatabaseType(String databaseType) {
this.databaseType = databaseType;
return this;
}
public String getDatabaseType() {
return this.databaseType;
}
public CreateUniBackupPolicyRequest setFullPlan(java.util.Map fullPlan) {
this.fullPlan = fullPlan;
return this;
}
public java.util.Map getFullPlan() {
return this.fullPlan;
}
public CreateUniBackupPolicyRequest setIncPlan(java.util.Map incPlan) {
this.incPlan = incPlan;
return this;
}
public java.util.Map getIncPlan() {
return this.incPlan;
}
public CreateUniBackupPolicyRequest setInstanceId(String instanceId) {
this.instanceId = instanceId;
return this;
}
public String getInstanceId() {
return this.instanceId;
}
public CreateUniBackupPolicyRequest setPolicyName(String policyName) {
this.policyName = policyName;
return this;
}
public String getPolicyName() {
return this.policyName;
}
public CreateUniBackupPolicyRequest setRetention(Integer retention) {
this.retention = retention;
return this;
}
public Integer getRetention() {
return this.retention;
}
public CreateUniBackupPolicyRequest setSpeedLimiter(Long speedLimiter) {
this.speedLimiter = speedLimiter;
return this;
}
public Long getSpeedLimiter() {
return this.speedLimiter;
}
public CreateUniBackupPolicyRequest setUniRegionId(String uniRegionId) {
this.uniRegionId = uniRegionId;
return this;
}
public String getUniRegionId() {
return this.uniRegionId;
}
public CreateUniBackupPolicyRequest setUuid(String uuid) {
this.uuid = uuid;
return this;
}
public String getUuid() {
return this.uuid;
}
}