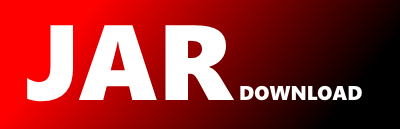
com.aliyun.sas20181203.models.DescribeBruteForceRecordsResponseBody Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class DescribeBruteForceRecordsResponseBody extends TeaModel {
/**
* The IP addresses.
*/
@NameInMap("MachineList")
public java.util.List machineList;
/**
* The pagination information.
*/
@NameInMap("PageInfo")
public DescribeBruteForceRecordsResponseBodyPageInfo pageInfo;
/**
* The ID of the request, which is used to locate and troubleshoot issues.
*
* example:
* 7E0618A9-D5EF-4220-9471-C42B5E92719F
*/
@NameInMap("RequestId")
public String requestId;
public static DescribeBruteForceRecordsResponseBody build(java.util.Map map) throws Exception {
DescribeBruteForceRecordsResponseBody self = new DescribeBruteForceRecordsResponseBody();
return TeaModel.build(map, self);
}
public DescribeBruteForceRecordsResponseBody setMachineList(java.util.List machineList) {
this.machineList = machineList;
return this;
}
public java.util.List getMachineList() {
return this.machineList;
}
public DescribeBruteForceRecordsResponseBody setPageInfo(DescribeBruteForceRecordsResponseBodyPageInfo pageInfo) {
this.pageInfo = pageInfo;
return this;
}
public DescribeBruteForceRecordsResponseBodyPageInfo getPageInfo() {
return this.pageInfo;
}
public DescribeBruteForceRecordsResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public static class DescribeBruteForceRecordsResponseBodyMachineList extends TeaModel {
/**
* The status of the host network extension. Valid values:
*
* - true: online
* - false: offline
*
*
* example:
* false
*/
@NameInMap("AliNetOnline")
public Boolean aliNetOnline;
/**
* The timestamp when the block action on the IP address becomes invalid.
*
* example:
* 1671506882063
*/
@NameInMap("BlockExpireDate")
public Long blockExpireDate;
/**
* The IP address that is blocked.
*
* example:
* 10.12.XX.XX
*/
@NameInMap("BlockIp")
public String blockIp;
/**
* The blocking type. Valid values:
*
* - group: security group
* - alinet: host network extension
*
*
* example:
* alinet
*/
@NameInMap("BlockType")
public String blockType;
/**
* The error code returned when the defense rule fails to block the IP address.
*
* example:
* InstanceSecurityGroupLimitExceeded
*/
@NameInMap("ErrorCode")
public String errorCode;
/**
* The ID of the primary key that is recorded in the defense rule.
*
* example:
* 112XX
*/
@NameInMap("Id")
public Long id;
/**
* The instance name of the server.
*
* example:
* record-test-***
*/
@NameInMap("InstanceName")
public String instanceName;
/**
* The public IP address.
*
* example:
* 120.79.XX.XX
*/
@NameInMap("InternetIp")
public String internetIp;
/**
* The private IP address.
*
* example:
* 192.168.XX.XX
*/
@NameInMap("IntranetIp")
public String intranetIp;
/**
* The port that is attacked.
*
* example:
* 22/22
*/
@NameInMap("Port")
public String port;
/**
* The name of the defense rule.
*
* example:
* AntiRuleName
*/
@NameInMap("RuleName")
public String ruleName;
/**
* The type of the defense rule. Valid values:
*
* - userRule: custom rule
* - blinkRule: system rule
*
*
* example:
* userRule
*/
@NameInMap("Source")
public String source;
/**
* The status of the defense rule. Valid values:
*
* - 0: invalid
* - 1: enabled
* - 2: failed
*
*
* example:
* 2
*/
@NameInMap("Status")
public Integer status;
/**
* The UUID of the server on which the defense rule takes effect.
*
* example:
* 6d5b361f-958d-48a8-a9d2-d6e82c1****
*/
@NameInMap("Uuid")
public String uuid;
public static DescribeBruteForceRecordsResponseBodyMachineList build(java.util.Map map) throws Exception {
DescribeBruteForceRecordsResponseBodyMachineList self = new DescribeBruteForceRecordsResponseBodyMachineList();
return TeaModel.build(map, self);
}
public DescribeBruteForceRecordsResponseBodyMachineList setAliNetOnline(Boolean aliNetOnline) {
this.aliNetOnline = aliNetOnline;
return this;
}
public Boolean getAliNetOnline() {
return this.aliNetOnline;
}
public DescribeBruteForceRecordsResponseBodyMachineList setBlockExpireDate(Long blockExpireDate) {
this.blockExpireDate = blockExpireDate;
return this;
}
public Long getBlockExpireDate() {
return this.blockExpireDate;
}
public DescribeBruteForceRecordsResponseBodyMachineList setBlockIp(String blockIp) {
this.blockIp = blockIp;
return this;
}
public String getBlockIp() {
return this.blockIp;
}
public DescribeBruteForceRecordsResponseBodyMachineList setBlockType(String blockType) {
this.blockType = blockType;
return this;
}
public String getBlockType() {
return this.blockType;
}
public DescribeBruteForceRecordsResponseBodyMachineList setErrorCode(String errorCode) {
this.errorCode = errorCode;
return this;
}
public String getErrorCode() {
return this.errorCode;
}
public DescribeBruteForceRecordsResponseBodyMachineList setId(Long id) {
this.id = id;
return this;
}
public Long getId() {
return this.id;
}
public DescribeBruteForceRecordsResponseBodyMachineList setInstanceName(String instanceName) {
this.instanceName = instanceName;
return this;
}
public String getInstanceName() {
return this.instanceName;
}
public DescribeBruteForceRecordsResponseBodyMachineList setInternetIp(String internetIp) {
this.internetIp = internetIp;
return this;
}
public String getInternetIp() {
return this.internetIp;
}
public DescribeBruteForceRecordsResponseBodyMachineList setIntranetIp(String intranetIp) {
this.intranetIp = intranetIp;
return this;
}
public String getIntranetIp() {
return this.intranetIp;
}
public DescribeBruteForceRecordsResponseBodyMachineList setPort(String port) {
this.port = port;
return this;
}
public String getPort() {
return this.port;
}
public DescribeBruteForceRecordsResponseBodyMachineList setRuleName(String ruleName) {
this.ruleName = ruleName;
return this;
}
public String getRuleName() {
return this.ruleName;
}
public DescribeBruteForceRecordsResponseBodyMachineList setSource(String source) {
this.source = source;
return this;
}
public String getSource() {
return this.source;
}
public DescribeBruteForceRecordsResponseBodyMachineList setStatus(Integer status) {
this.status = status;
return this;
}
public Integer getStatus() {
return this.status;
}
public DescribeBruteForceRecordsResponseBodyMachineList setUuid(String uuid) {
this.uuid = uuid;
return this;
}
public String getUuid() {
return this.uuid;
}
}
public static class DescribeBruteForceRecordsResponseBodyPageInfo extends TeaModel {
/**
* The number of entries returned on the current page.
*
* example:
* 10
*/
@NameInMap("Count")
public Integer count;
/**
* The page number of the returned page.
*
* example:
* 1
*/
@NameInMap("CurrentPage")
public Integer currentPage;
/**
* The number of entries returned per page. Default value: 20.
*
* example:
* 20
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The total number of entries returned.
*
* example:
* 263
*/
@NameInMap("TotalCount")
public Integer totalCount;
public static DescribeBruteForceRecordsResponseBodyPageInfo build(java.util.Map map) throws Exception {
DescribeBruteForceRecordsResponseBodyPageInfo self = new DescribeBruteForceRecordsResponseBodyPageInfo();
return TeaModel.build(map, self);
}
public DescribeBruteForceRecordsResponseBodyPageInfo setCount(Integer count) {
this.count = count;
return this;
}
public Integer getCount() {
return this.count;
}
public DescribeBruteForceRecordsResponseBodyPageInfo setCurrentPage(Integer currentPage) {
this.currentPage = currentPage;
return this;
}
public Integer getCurrentPage() {
return this.currentPage;
}
public DescribeBruteForceRecordsResponseBodyPageInfo setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public DescribeBruteForceRecordsResponseBodyPageInfo setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
}
}