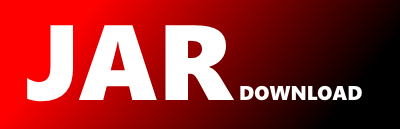
com.aliyun.sas20181203.models.DescribeCustomBlockRecordsResponseBody Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class DescribeCustomBlockRecordsResponseBody extends TeaModel {
/**
* The pagination information.
*/
@NameInMap("PageInfo")
public DescribeCustomBlockRecordsResponseBodyPageInfo pageInfo;
/**
* An array that consists of the defense rules.
*/
@NameInMap("RecordList")
public java.util.List recordList;
/**
* The ID of the request, which is used to locate and troubleshoot issues.
*
* example:
* 028CF634-5268-5660-9575-48C9ED6B7T8Y
*/
@NameInMap("RequestId")
public String requestId;
public static DescribeCustomBlockRecordsResponseBody build(java.util.Map map) throws Exception {
DescribeCustomBlockRecordsResponseBody self = new DescribeCustomBlockRecordsResponseBody();
return TeaModel.build(map, self);
}
public DescribeCustomBlockRecordsResponseBody setPageInfo(DescribeCustomBlockRecordsResponseBodyPageInfo pageInfo) {
this.pageInfo = pageInfo;
return this;
}
public DescribeCustomBlockRecordsResponseBodyPageInfo getPageInfo() {
return this.pageInfo;
}
public DescribeCustomBlockRecordsResponseBody setRecordList(java.util.List recordList) {
this.recordList = recordList;
return this;
}
public java.util.List getRecordList() {
return this.recordList;
}
public DescribeCustomBlockRecordsResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public static class DescribeCustomBlockRecordsResponseBodyPageInfo extends TeaModel {
/**
* The number of entries returned on the current page.
*
* example:
* 3
*/
@NameInMap("Count")
public Integer count;
/**
* The page number of the returned page.
*
* example:
* 1
*/
@NameInMap("CurrentPage")
public Integer currentPage;
/**
* The number of entries returned per page.
*
* example:
* 20
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The total number of entries returned.
*
* example:
* 708
*/
@NameInMap("TotalCount")
public Integer totalCount;
public static DescribeCustomBlockRecordsResponseBodyPageInfo build(java.util.Map map) throws Exception {
DescribeCustomBlockRecordsResponseBodyPageInfo self = new DescribeCustomBlockRecordsResponseBodyPageInfo();
return TeaModel.build(map, self);
}
public DescribeCustomBlockRecordsResponseBodyPageInfo setCount(Integer count) {
this.count = count;
return this;
}
public Integer getCount() {
return this.count;
}
public DescribeCustomBlockRecordsResponseBodyPageInfo setCurrentPage(Integer currentPage) {
this.currentPage = currentPage;
return this;
}
public Integer getCurrentPage() {
return this.currentPage;
}
public DescribeCustomBlockRecordsResponseBodyPageInfo setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public DescribeCustomBlockRecordsResponseBodyPageInfo setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
}
public static class DescribeCustomBlockRecordsResponseBodyRecordListTargetList extends TeaModel {
/**
* The ID of the destination asset.
*
* example:
* 032b618f-b220-4a0d-bd37-fbdc6*******
*/
@NameInMap("Target")
public String target;
/**
* The type of the query. Valid values:
*
* - Set the value to uuid.
*
*
* example:
* uuid
*/
@NameInMap("TargetType")
public String targetType;
public static DescribeCustomBlockRecordsResponseBodyRecordListTargetList build(java.util.Map map) throws Exception {
DescribeCustomBlockRecordsResponseBodyRecordListTargetList self = new DescribeCustomBlockRecordsResponseBodyRecordListTargetList();
return TeaModel.build(map, self);
}
public DescribeCustomBlockRecordsResponseBodyRecordListTargetList setTarget(String target) {
this.target = target;
return this;
}
public String getTarget() {
return this.target;
}
public DescribeCustomBlockRecordsResponseBodyRecordListTargetList setTargetType(String targetType) {
this.targetType = targetType;
return this;
}
public String getTargetType() {
return this.targetType;
}
}
public static class DescribeCustomBlockRecordsResponseBodyRecordList extends TeaModel {
/**
* The timestamp generated when the block action on the IP address becomes invalid.
*
* example:
* 1671506882063
*/
@NameInMap("BlockExpireDate")
public Long blockExpireDate;
/**
* The blocked IP address.
*
* example:
* 45.227.XX.XX
*/
@NameInMap("BlockIp")
public String blockIp;
/**
* The direction of the traffic that is sent by the blocked IP address. Valid values:
*
* - in
* - out
*
*
* example:
* in
*/
@NameInMap("Bound")
public String bound;
/**
* The number of servers for which the defense rule is enabled.
*
* example:
* 4
*/
@NameInMap("EnableCount")
public Integer enableCount;
/**
* The record ID.
*
* example:
* 353376
*/
@NameInMap("Id")
public Long id;
/**
* The total number of servers on which the IP address is blocked.
*
* example:
* 6
*/
@NameInMap("ServerCount")
public Integer serverCount;
/**
* The source of the defense rule.
*
* example:
* UserRule
*/
@NameInMap("Source")
public String source;
/**
* The status of the defense rule against brute-force attacks. Valid values:
*
* - 0: invalid.
* - 1: enabled.
* - 2: failed.
*
*
* example:
* 1
*/
@NameInMap("Status")
public Integer status;
/**
* The servers for which the defense rule is enabled.
*/
@NameInMap("TargetList")
public java.util.List targetList;
public static DescribeCustomBlockRecordsResponseBodyRecordList build(java.util.Map map) throws Exception {
DescribeCustomBlockRecordsResponseBodyRecordList self = new DescribeCustomBlockRecordsResponseBodyRecordList();
return TeaModel.build(map, self);
}
public DescribeCustomBlockRecordsResponseBodyRecordList setBlockExpireDate(Long blockExpireDate) {
this.blockExpireDate = blockExpireDate;
return this;
}
public Long getBlockExpireDate() {
return this.blockExpireDate;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setBlockIp(String blockIp) {
this.blockIp = blockIp;
return this;
}
public String getBlockIp() {
return this.blockIp;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setBound(String bound) {
this.bound = bound;
return this;
}
public String getBound() {
return this.bound;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setEnableCount(Integer enableCount) {
this.enableCount = enableCount;
return this;
}
public Integer getEnableCount() {
return this.enableCount;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setId(Long id) {
this.id = id;
return this;
}
public Long getId() {
return this.id;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setServerCount(Integer serverCount) {
this.serverCount = serverCount;
return this;
}
public Integer getServerCount() {
return this.serverCount;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setSource(String source) {
this.source = source;
return this;
}
public String getSource() {
return this.source;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setStatus(Integer status) {
this.status = status;
return this;
}
public Integer getStatus() {
return this.status;
}
public DescribeCustomBlockRecordsResponseBodyRecordList setTargetList(java.util.List targetList) {
this.targetList = targetList;
return this;
}
public java.util.List getTargetList() {
return this.targetList;
}
}
}