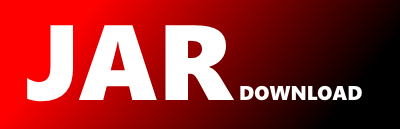
com.aliyun.sas20181203.models.DescribeTraceInfoDetailResponseBody Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class DescribeTraceInfoDetailResponseBody extends TeaModel {
/**
* The ID of the request.
*
* example:
* 24A20733-10A0-4AF6-BE6B-XXXXXXXXX
*/
@NameInMap("RequestId")
public String requestId;
/**
* Indicates whether the request was successful. Valid values:
*
* - true: The request was successful.
* - false: The request failed.
*
*
* example:
* true
*/
@NameInMap("Success")
public Boolean success;
/**
* The details of the tracing diagram.
*/
@NameInMap("TraceInfoDetail")
public DescribeTraceInfoDetailResponseBodyTraceInfoDetail traceInfoDetail;
public static DescribeTraceInfoDetailResponseBody build(java.util.Map map) throws Exception {
DescribeTraceInfoDetailResponseBody self = new DescribeTraceInfoDetailResponseBody();
return TeaModel.build(map, self);
}
public DescribeTraceInfoDetailResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeTraceInfoDetailResponseBody setSuccess(Boolean success) {
this.success = success;
return this;
}
public Boolean getSuccess() {
return this.success;
}
public DescribeTraceInfoDetailResponseBody setTraceInfoDetail(DescribeTraceInfoDetailResponseBodyTraceInfoDetail traceInfoDetail) {
this.traceInfoDetail = traceInfoDetail;
return this;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetail getTraceInfoDetail() {
return this.traceInfoDetail;
}
public static class DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList extends TeaModel {
/**
* The number of times.
*
* example:
* 1
*/
@NameInMap("Count")
public Integer count;
/**
* The ending vertex ID of the edge of the tracing diagram.
*
* example:
* a1d1fa39e5345dcef3f9712172cxxxxx
*/
@NameInMap("EndId")
public String endId;
/**
* The starting vertex ID of the edge of the tracing diagram.
*
* example:
* 02b4bf933c8e3bb8b9465eee502xxxxx
*/
@NameInMap("StartId")
public String startId;
/**
* The point in time.
*
* example:
* 2022-12-21 10:24:42
*/
@NameInMap("Time")
public String time;
/**
* The type of the edge of the tracing diagram.
*
* example:
* trigger_file_alert
*/
@NameInMap("Type")
public String type;
public static DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList build(java.util.Map map) throws Exception {
DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList self = new DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList();
return TeaModel.build(map, self);
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList setCount(Integer count) {
this.count = count;
return this;
}
public Integer getCount() {
return this.count;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList setEndId(String endId) {
this.endId = endId;
return this;
}
public String getEndId() {
return this.endId;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList setStartId(String startId) {
this.startId = startId;
return this;
}
public String getStartId() {
return this.startId;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList setTime(String time) {
this.time = time;
return this;
}
public String getTime() {
return this.time;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEdgeList setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
}
public static class DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList extends TeaModel {
/**
* This parameter is deprecated.
*
* example:
* Deprecated
*/
@NameInMap("DbId")
public Integer dbId;
/**
* The rendering color of the vertex.
*
* example:
* #fff
*/
@NameInMap("DisplayColor")
public String displayColor;
/**
* The icon style of the vertex.
*
* example:
* https://img.alicdn.com/tfs/TB176P5OgDqK1RjSZSyXXaxEVXa-49-48.png
*/
@NameInMap("DisplayIcon")
public String displayIcon;
/**
* This parameter is deprecated.
*
* example:
* [{"name":"${logtime}","value":"$!{time}"}]
*/
@NameInMap("DisplayTemplate")
public String displayTemplate;
/**
* The timestamp when the vertex was created.
*
* example:
* 2022-10-09T11:47Z
*/
@NameInMap("GmtCreate")
public Long gmtCreate;
/**
* The time when the vertex was last modified.
*
* example:
* 2022-10-09T11:47Z
*/
@NameInMap("GmtModified")
public Long gmtModified;
/**
* The ID of the vertex type.
*
* example:
* Alert
*/
@NameInMap("Id")
public String id;
/**
* This parameter is deprecated.
*
* example:
* Deprecated
*/
@NameInMap("Limit")
public Integer limit;
/**
* The name of the vertex type.
*
* example:
* Alert
*/
@NameInMap("Name")
public String name;
/**
* The namespace.
*
* example:
*
*
*
*/
@NameInMap("Namespace")
public String namespace;
/**
* This parameter is deprecated.
*
* example:
* Deprecated
*/
@NameInMap("Offset")
public Integer offset;
public static DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList build(java.util.Map map) throws Exception {
DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList self = new DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList();
return TeaModel.build(map, self);
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setDbId(Integer dbId) {
this.dbId = dbId;
return this;
}
public Integer getDbId() {
return this.dbId;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setDisplayColor(String displayColor) {
this.displayColor = displayColor;
return this;
}
public String getDisplayColor() {
return this.displayColor;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setDisplayIcon(String displayIcon) {
this.displayIcon = displayIcon;
return this;
}
public String getDisplayIcon() {
return this.displayIcon;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setDisplayTemplate(String displayTemplate) {
this.displayTemplate = displayTemplate;
return this;
}
public String getDisplayTemplate() {
return this.displayTemplate;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setGmtCreate(Long gmtCreate) {
this.gmtCreate = gmtCreate;
return this;
}
public Long getGmtCreate() {
return this.gmtCreate;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setGmtModified(Long gmtModified) {
this.gmtModified = gmtModified;
return this;
}
public Long getGmtModified() {
return this.gmtModified;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setId(String id) {
this.id = id;
return this;
}
public String getId() {
return this.id;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setLimit(Integer limit) {
this.limit = limit;
return this;
}
public Integer getLimit() {
return this.limit;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setNamespace(String namespace) {
this.namespace = namespace;
return this;
}
public String getNamespace() {
return this.namespace;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailEntityTypeList setOffset(Integer offset) {
this.offset = offset;
return this;
}
public Integer getOffset() {
return this.offset;
}
}
public static class DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList extends TeaModel {
/**
* Indicates whether the edge is a directional edge. Valid values:
*
* - 1: yes
* - 0: no
*
*
* example:
* 1
*/
@NameInMap("Directed")
public Integer directed;
/**
* The rendering color of the edge.
*
* example:
* #fff
*/
@NameInMap("DisplayColor")
public String displayColor;
/**
* The name of the edge type.
*
* example:
* file
*/
@NameInMap("Name")
public String name;
/**
* The ID of the edge type.
*
* example:
* netflow_to_process
*/
@NameInMap("RelationTypeId")
public String relationTypeId;
/**
* This parameter is deprecated.
*
* example:
* Deprecated
*/
@NameInMap("ShowType")
public String showType;
public static DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList build(java.util.Map map) throws Exception {
DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList self = new DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList();
return TeaModel.build(map, self);
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList setDirected(Integer directed) {
this.directed = directed;
return this;
}
public Integer getDirected() {
return this.directed;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList setDisplayColor(String displayColor) {
this.displayColor = displayColor;
return this;
}
public String getDisplayColor() {
return this.displayColor;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList setRelationTypeId(String relationTypeId) {
this.relationTypeId = relationTypeId;
return this;
}
public String getRelationTypeId() {
return this.relationTypeId;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailRelationTypeList setShowType(String showType) {
this.showType = showType;
return this;
}
public String getShowType() {
return this.showType;
}
}
public static class DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexListNeighborList extends TeaModel {
/**
* The number of neighbor nodes.
*
* example:
* 1
*/
@NameInMap("Count")
public Integer count;
/**
* Indicates whether one more page is returned.
*
* example:
* False
*/
@NameInMap("HasMore")
public Boolean hasMore;
/**
* The type of the neighbor node. The value is fixed as alert.
*
* example:
* alert
*/
@NameInMap("Type")
public String type;
public static DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexListNeighborList build(java.util.Map map) throws Exception {
DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexListNeighborList self = new DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexListNeighborList();
return TeaModel.build(map, self);
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexListNeighborList setCount(Integer count) {
this.count = count;
return this;
}
public Integer getCount() {
return this.count;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexListNeighborList setHasMore(Boolean hasMore) {
this.hasMore = hasMore;
return this;
}
public Boolean getHasMore() {
return this.hasMore;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexListNeighborList setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
}
public static class DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList extends TeaModel {
/**
* The number of times.
*
* example:
* 1
*/
@NameInMap("Count")
public Integer count;
/**
* The ID of the vertex.
*
* example:
* a1d1fa39e5345dcef3f9712172xxxxxx
*/
@NameInMap("Id")
public String id;
/**
* The name of the entity represented by the vertex.
*
* example:
* /usr/local/tomcat
*/
@NameInMap("Name")
public String name;
/**
* An array that consists of the neighbor nodes.
*/
@NameInMap("NeighborList")
public java.util.List neighborList;
/**
* The point in time.
*
* example:
* 2022-12-21 10:24:42
*/
@NameInMap("Time")
public String time;
/**
* The type of the entity represented by the vertex.
*
* example:
* file_path
*/
@NameInMap("Type")
public String type;
public static DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList build(java.util.Map map) throws Exception {
DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList self = new DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList();
return TeaModel.build(map, self);
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList setCount(Integer count) {
this.count = count;
return this;
}
public Integer getCount() {
return this.count;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList setId(String id) {
this.id = id;
return this;
}
public String getId() {
return this.id;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList setNeighborList(java.util.List neighborList) {
this.neighborList = neighborList;
return this;
}
public java.util.List getNeighborList() {
return this.neighborList;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList setTime(String time) {
this.time = time;
return this;
}
public String getTime() {
return this.time;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetailVertexList setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
}
public static class DescribeTraceInfoDetailResponseBodyTraceInfoDetail extends TeaModel {
/**
* An array that consists of the edges of the tracing diagram.
*/
@NameInMap("EdgeList")
public java.util.List edgeList;
/**
* An array that consists of the metadata configurations of the vertex type.
*/
@NameInMap("EntityTypeList")
public java.util.List entityTypeList;
/**
* An array that consists of the metadata configurations of the edge type.
*/
@NameInMap("RelationTypeList")
public java.util.List relationTypeList;
/**
* An array that consists of all vertexes of the tracing diagram.
*/
@NameInMap("VertexList")
public java.util.List vertexList;
public static DescribeTraceInfoDetailResponseBodyTraceInfoDetail build(java.util.Map map) throws Exception {
DescribeTraceInfoDetailResponseBodyTraceInfoDetail self = new DescribeTraceInfoDetailResponseBodyTraceInfoDetail();
return TeaModel.build(map, self);
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetail setEdgeList(java.util.List edgeList) {
this.edgeList = edgeList;
return this;
}
public java.util.List getEdgeList() {
return this.edgeList;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetail setEntityTypeList(java.util.List entityTypeList) {
this.entityTypeList = entityTypeList;
return this;
}
public java.util.List getEntityTypeList() {
return this.entityTypeList;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetail setRelationTypeList(java.util.List relationTypeList) {
this.relationTypeList = relationTypeList;
return this;
}
public java.util.List getRelationTypeList() {
return this.relationTypeList;
}
public DescribeTraceInfoDetailResponseBodyTraceInfoDetail setVertexList(java.util.List vertexList) {
this.vertexList = vertexList;
return this;
}
public java.util.List getVertexList() {
return this.vertexList;
}
}
}