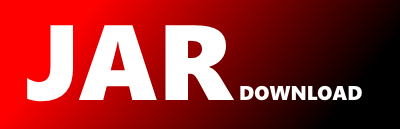
com.aliyun.sas20181203.models.ListCheckItemWarningMachineRequest Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class ListCheckItemWarningMachineRequest extends TeaModel {
/**
* The ID of the check item.
* This parameter is required.
*
* example:
* 8
*/
@NameInMap("CheckId")
public Long checkId;
/**
* The name of the field that is used to query containers.
*
* example:
* clusterId
*/
@NameInMap("ContainerFieldName")
public String containerFieldName;
/**
* The value of the field that is used to query containers.
*
* example:
* ce89cdd0ea732472a8703821b19e****
*/
@NameInMap("ContainerFieldValue")
public String containerFieldValue;
/**
* The number of the page to return. Pages start from page 1. Default value: 1.
*
* example:
* 1
*/
@NameInMap("CurrentPage")
public Integer currentPage;
/**
* The ID of the asset group.
*
* You can call the DescribeAllGroups operation to query the ID of the asset group.
*
*
* example:
* 1161****
*/
@NameInMap("GroupId")
public Long groupId;
/**
* The language of the content within the request and response. Default value: zh. Valid values:
*
* - zh: Chinese
* - en: English
*
*
* example:
* zh
*/
@NameInMap("Lang")
public String lang;
/**
* The number of entries to return on each page. Default value: 20. If you leave this parameter empty, 20 entries are returned on each page.
*
* We recommend that you do not leave this parameter empty.
*
*
* example:
* 20
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The keyword that is used to query servers in fuzzy match mode.
*
* example:
* 225
*/
@NameInMap("Remark")
public String remark;
/**
* The type of the check item.
*
* example:
* cis
*/
@NameInMap("RiskType")
public String riskType;
/**
* The data source. Default value: default. Valid values:
*
* - default: The check items of baselines for hosts.
* - agentless: The check items of baselines for agentless detection.
*
*
* example:
* agentless
*/
@NameInMap("Source")
public String source;
/**
* The status of the check item. Valid values:
*
* 1: failed
*
* 2: verifying
*
* 3: passed
*
* 6: ignored
*
* 7: fixing
*
*
*
* example:
* 3
*/
@NameInMap("Status")
public Integer status;
/**
* The UUID array of the servers.
*/
@NameInMap("UuidList")
public java.util.List uuidList;
public static ListCheckItemWarningMachineRequest build(java.util.Map map) throws Exception {
ListCheckItemWarningMachineRequest self = new ListCheckItemWarningMachineRequest();
return TeaModel.build(map, self);
}
public ListCheckItemWarningMachineRequest setCheckId(Long checkId) {
this.checkId = checkId;
return this;
}
public Long getCheckId() {
return this.checkId;
}
public ListCheckItemWarningMachineRequest setContainerFieldName(String containerFieldName) {
this.containerFieldName = containerFieldName;
return this;
}
public String getContainerFieldName() {
return this.containerFieldName;
}
public ListCheckItemWarningMachineRequest setContainerFieldValue(String containerFieldValue) {
this.containerFieldValue = containerFieldValue;
return this;
}
public String getContainerFieldValue() {
return this.containerFieldValue;
}
public ListCheckItemWarningMachineRequest setCurrentPage(Integer currentPage) {
this.currentPage = currentPage;
return this;
}
public Integer getCurrentPage() {
return this.currentPage;
}
public ListCheckItemWarningMachineRequest setGroupId(Long groupId) {
this.groupId = groupId;
return this;
}
public Long getGroupId() {
return this.groupId;
}
public ListCheckItemWarningMachineRequest setLang(String lang) {
this.lang = lang;
return this;
}
public String getLang() {
return this.lang;
}
public ListCheckItemWarningMachineRequest setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public ListCheckItemWarningMachineRequest setRemark(String remark) {
this.remark = remark;
return this;
}
public String getRemark() {
return this.remark;
}
public ListCheckItemWarningMachineRequest setRiskType(String riskType) {
this.riskType = riskType;
return this;
}
public String getRiskType() {
return this.riskType;
}
public ListCheckItemWarningMachineRequest setSource(String source) {
this.source = source;
return this;
}
public String getSource() {
return this.source;
}
public ListCheckItemWarningMachineRequest setStatus(Integer status) {
this.status = status;
return this;
}
public Integer getStatus() {
return this.status;
}
public ListCheckItemWarningMachineRequest setUuidList(java.util.List uuidList) {
this.uuidList = uuidList;
return this;
}
public java.util.List getUuidList() {
return this.uuidList;
}
}