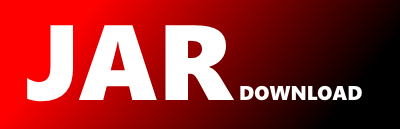
com.aliyun.sas20181203.models.ListFileProtectRuleResponseBody Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class ListFileProtectRuleResponseBody extends TeaModel {
/**
* The details of returned data.
*/
@NameInMap("FileProtectList")
public java.util.List fileProtectList;
/**
* The pagination information.
*/
@NameInMap("PageInfo")
public ListFileProtectRuleResponseBodyPageInfo pageInfo;
/**
* The request ID.
*
* example:
* FBBEB173-1F43-505F-A876-C03ECDF6CE4C
*/
@NameInMap("RequestId")
public String requestId;
public static ListFileProtectRuleResponseBody build(java.util.Map map) throws Exception {
ListFileProtectRuleResponseBody self = new ListFileProtectRuleResponseBody();
return TeaModel.build(map, self);
}
public ListFileProtectRuleResponseBody setFileProtectList(java.util.List fileProtectList) {
this.fileProtectList = fileProtectList;
return this;
}
public java.util.List getFileProtectList() {
return this.fileProtectList;
}
public ListFileProtectRuleResponseBody setPageInfo(ListFileProtectRuleResponseBodyPageInfo pageInfo) {
this.pageInfo = pageInfo;
return this;
}
public ListFileProtectRuleResponseBodyPageInfo getPageInfo() {
return this.pageInfo;
}
public ListFileProtectRuleResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public static class ListFileProtectRuleResponseBodyFileProtectList extends TeaModel {
/**
* The handling method of the rule. Valid values:
*
* - pass: allow
* - alert
*
*
* example:
* pass
*/
@NameInMap("Action")
public String action;
/**
* The severity of alerts. Valid values:
*
* - 0: does not generate alerts
* - 1: sends notifications
* - 2: suspicious
* - 3: high-risk
*
*
* example:
* 0
*/
@NameInMap("AlertLevel")
public String alertLevel;
/**
* The total number of affected assets.
*
* example:
* 12
*/
@NameInMap("EffectInstanceCount")
public Long effectInstanceCount;
/**
* The operations performed on the files.
*/
@NameInMap("FileOps")
public java.util.List fileOps;
/**
* The paths to the monitored files. Wildcard characters are supported.
*/
@NameInMap("FilePaths")
public java.util.List filePaths;
/**
* The time when the rule was created.
*
* example:
* 1682304179000
*/
@NameInMap("GmtCreate")
public Long gmtCreate;
/**
* The time when the rule was last modified.
*
* example:
* 1682304179000
*/
@NameInMap("GmtModified")
public Long gmtModified;
/**
* The ID of the rule.
*
* example:
* 1412511
*/
@NameInMap("Id")
public Long id;
/**
* The type of the operating system. Valid values:
*
* - windows: Windows
* - linux: Linux
*
*
* example:
* linux
*/
@NameInMap("Platform")
public String platform;
/**
* The paths to the monitored processes. Wildcard characters are supported.
*/
@NameInMap("ProcPaths")
public java.util.List procPaths;
/**
* The name of the rule.
*
* example:
* test11
*/
@NameInMap("RuleName")
public String ruleName;
/**
* The status of the rule. Valid values:
*
* - 0: disabled
* - 1: enabled
*
*
* example:
* 1
*/
@NameInMap("Status")
public Integer status;
/**
* The switch ID of the rule.
*
* example:
* FILE_PROTECT_RULE_SWITCH_TYPE_1693474122927
*/
@NameInMap("SwitchId")
public String switchId;
public static ListFileProtectRuleResponseBodyFileProtectList build(java.util.Map map) throws Exception {
ListFileProtectRuleResponseBodyFileProtectList self = new ListFileProtectRuleResponseBodyFileProtectList();
return TeaModel.build(map, self);
}
public ListFileProtectRuleResponseBodyFileProtectList setAction(String action) {
this.action = action;
return this;
}
public String getAction() {
return this.action;
}
public ListFileProtectRuleResponseBodyFileProtectList setAlertLevel(String alertLevel) {
this.alertLevel = alertLevel;
return this;
}
public String getAlertLevel() {
return this.alertLevel;
}
public ListFileProtectRuleResponseBodyFileProtectList setEffectInstanceCount(Long effectInstanceCount) {
this.effectInstanceCount = effectInstanceCount;
return this;
}
public Long getEffectInstanceCount() {
return this.effectInstanceCount;
}
public ListFileProtectRuleResponseBodyFileProtectList setFileOps(java.util.List fileOps) {
this.fileOps = fileOps;
return this;
}
public java.util.List getFileOps() {
return this.fileOps;
}
public ListFileProtectRuleResponseBodyFileProtectList setFilePaths(java.util.List filePaths) {
this.filePaths = filePaths;
return this;
}
public java.util.List getFilePaths() {
return this.filePaths;
}
public ListFileProtectRuleResponseBodyFileProtectList setGmtCreate(Long gmtCreate) {
this.gmtCreate = gmtCreate;
return this;
}
public Long getGmtCreate() {
return this.gmtCreate;
}
public ListFileProtectRuleResponseBodyFileProtectList setGmtModified(Long gmtModified) {
this.gmtModified = gmtModified;
return this;
}
public Long getGmtModified() {
return this.gmtModified;
}
public ListFileProtectRuleResponseBodyFileProtectList setId(Long id) {
this.id = id;
return this;
}
public Long getId() {
return this.id;
}
public ListFileProtectRuleResponseBodyFileProtectList setPlatform(String platform) {
this.platform = platform;
return this;
}
public String getPlatform() {
return this.platform;
}
public ListFileProtectRuleResponseBodyFileProtectList setProcPaths(java.util.List procPaths) {
this.procPaths = procPaths;
return this;
}
public java.util.List getProcPaths() {
return this.procPaths;
}
public ListFileProtectRuleResponseBodyFileProtectList setRuleName(String ruleName) {
this.ruleName = ruleName;
return this;
}
public String getRuleName() {
return this.ruleName;
}
public ListFileProtectRuleResponseBodyFileProtectList setStatus(Integer status) {
this.status = status;
return this;
}
public Integer getStatus() {
return this.status;
}
public ListFileProtectRuleResponseBodyFileProtectList setSwitchId(String switchId) {
this.switchId = switchId;
return this;
}
public String getSwitchId() {
return this.switchId;
}
}
public static class ListFileProtectRuleResponseBodyPageInfo extends TeaModel {
/**
* The page number.
*
* example:
* 1
*/
@NameInMap("CurrentPage")
public Integer currentPage;
/**
* The number of entries per page.
*
* example:
* 20
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The total number of entries returned.
*
* example:
* 253
*/
@NameInMap("TotalCount")
public Integer totalCount;
public static ListFileProtectRuleResponseBodyPageInfo build(java.util.Map map) throws Exception {
ListFileProtectRuleResponseBodyPageInfo self = new ListFileProtectRuleResponseBodyPageInfo();
return TeaModel.build(map, self);
}
public ListFileProtectRuleResponseBodyPageInfo setCurrentPage(Integer currentPage) {
this.currentPage = currentPage;
return this;
}
public Integer getCurrentPage() {
return this.currentPage;
}
public ListFileProtectRuleResponseBodyPageInfo setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public ListFileProtectRuleResponseBodyPageInfo setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
}
}