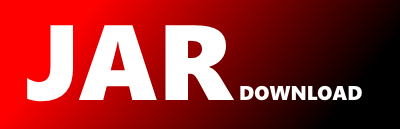
com.aliyun.sas20181203.models.QueryIncidentTracingDetailResponseBody Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class QueryIncidentTracingDetailResponseBody extends TeaModel {
/**
* The request ID.
*
* example:
* D2956025-4E5C-529D-92B4-B2591DDED067
*/
@NameInMap("RequestId")
public String requestId;
/**
* Indicates whether the request was successful. Valid values:
*
* - true
* - false
*
*
* example:
* True
*/
@NameInMap("Success")
public Boolean success;
/**
* The information about the provenance graph.
*/
@NameInMap("TracingDetail")
public QueryIncidentTracingDetailResponseBodyTracingDetail tracingDetail;
public static QueryIncidentTracingDetailResponseBody build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBody self = new QueryIncidentTracingDetailResponseBody();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public QueryIncidentTracingDetailResponseBody setSuccess(Boolean success) {
this.success = success;
return this;
}
public Boolean getSuccess() {
return this.success;
}
public QueryIncidentTracingDetailResponseBody setTracingDetail(QueryIncidentTracingDetailResponseBodyTracingDetail tracingDetail) {
this.tracingDetail = tracingDetail;
return this;
}
public QueryIncidentTracingDetailResponseBodyTracingDetail getTracingDetail() {
return this.tracingDetail;
}
public static class QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList extends TeaModel {
/**
* The ID of the Alibaba Cloud account to which the current edge belongs.
*
* example:
* 1277498600854739
*/
@NameInMap("Aliuid")
public String aliuid;
/**
* The ID of the end node for the current edge.
*
* example:
* 223a185f05e5fc3c637
*/
@NameInMap("EndId")
public String endId;
/**
* The type of the end node for the current edge. Valid values include the following values:
*
* - process
* - file
* - alert
* - ip
* - domain
*
*
* example:
* process_test_process
*/
@NameInMap("EndType")
public String endType;
/**
* The name of the current edge.
*
* example:
* mongod
*/
@NameInMap("Name")
public String name;
/**
* The origin vertex ID of the current edge.
*
* example:
* distribution
*/
@NameInMap("Origin")
public String origin;
/**
* The text that contains the properties of the current edge.
*
* example:
* {\"bandWidth\":\"8192\",\"internetIp\":\"8.211.13.50\",\"changeReason\":\"EIP_BIND\",\"bindInstanceId\":\"i-gw887xhzjvyjfv7vdfs3\",\"bindType\":\"EIP_ECS\"}
*/
@NameInMap("Properties")
public String properties;
/**
* The property of the current edge.
*
* example:
* {\"coverage\":\"global\"}
*/
@NameInMap("Property")
public java.util.Map property;
/**
* The ID of the rule based on which the current edge is generated.
*
* example:
* 136
*/
@NameInMap("RuleId")
public String ruleId;
/**
* The display type of the current edge.
*
* example:
* 0
*/
@NameInMap("ShowType")
public String showType;
/**
* The ID of the start node for the current edge.
*
* example:
* 23003
*/
@NameInMap("StartId")
public String startId;
/**
* The type of the start node for the current edge. Valid values include the following values:
*
* - process
* - file
* - alert:
* - ip
* - domain
*
*
* example:
* process
*/
@NameInMap("StartType")
public String startType;
/**
* The time when the current edge was created.
*
* example:
* 1652941117
*/
@NameInMap("Time")
public String time;
/**
* The UNIX timestamp when the current edge was created. Unit: milliseconds.
*
* example:
* 1636092632
*/
@NameInMap("Timestamp")
public Long timestamp;
/**
* The type of the current edge. Valid values include the following values:
*
* - process_exec_file: The relationship indicates an executable file that is run by a process.
* - process_connect_ip: The relationship indicates an IP address that is connected by a process.
* - domain_trgger_alert: The relationship indicates an alert that is triggered for a domain name.
*
*
* example:
* elf
*/
@NameInMap("Type")
public String type;
/**
* The type of the current edge.
*
* example:
* cis
*/
@NameInMap("TypeName")
public String typeName;
/**
* The time when the current edge was updated.
*
* example:
* 2022-01-13 12:49:33
*/
@NameInMap("UpdateTime")
public String updateTime;
/**
* The UUID of the current edge. The SIEM system generates UUIDs for nodes and edges in the provenance graph to help you locate the nodes or edges.
*
* example:
* 678e29f4-d78f-4a7c-a2bc-38434a138538
*/
@NameInMap("Uuid")
public String uuid;
public static QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList self = new QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setAliuid(String aliuid) {
this.aliuid = aliuid;
return this;
}
public String getAliuid() {
return this.aliuid;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setEndId(String endId) {
this.endId = endId;
return this;
}
public String getEndId() {
return this.endId;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setEndType(String endType) {
this.endType = endType;
return this;
}
public String getEndType() {
return this.endType;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setOrigin(String origin) {
this.origin = origin;
return this;
}
public String getOrigin() {
return this.origin;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setProperties(String properties) {
this.properties = properties;
return this;
}
public String getProperties() {
return this.properties;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setProperty(java.util.Map property) {
this.property = property;
return this;
}
public java.util.Map getProperty() {
return this.property;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setRuleId(String ruleId) {
this.ruleId = ruleId;
return this;
}
public String getRuleId() {
return this.ruleId;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setShowType(String showType) {
this.showType = showType;
return this;
}
public String getShowType() {
return this.showType;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setStartId(String startId) {
this.startId = startId;
return this;
}
public String getStartId() {
return this.startId;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setStartType(String startType) {
this.startType = startType;
return this;
}
public String getStartType() {
return this.startType;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setTime(String time) {
this.time = time;
return this;
}
public String getTime() {
return this.time;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setTimestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
public Long getTimestamp() {
return this.timestamp;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setTypeName(String typeName) {
this.typeName = typeName;
return this;
}
public String getTypeName() {
return this.typeName;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
public String getUpdateTime() {
return this.updateTime;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEdgeList setUuid(String uuid) {
this.uuid = uuid;
return this;
}
public String getUuid() {
return this.uuid;
}
}
public static class QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList extends TeaModel {
/**
* The version ID of the current entity.
*
* example:
* 1768
*/
@NameInMap("CurrentVersionId")
public String currentVersionId;
/**
* The display color of the current entity.
*
* example:
* #FFF
*/
@NameInMap("DisplayColor")
public String displayColor;
/**
* The display icon of the current entity.
*
* example:
*
*
*
*/
@NameInMap("DisplayIcon")
public String displayIcon;
/**
* The display sequence of the current entity.
*
* example:
* 2
*/
@NameInMap("DisplayOrder")
public Integer displayOrder;
/**
* The display template of the current entity.
*
* example:
* []
*/
@NameInMap("DisplayTemplate")
public String displayTemplate;
/**
* The time when the current entity was created.
*
* example:
* 2022-10-09T10:53Z
*/
@NameInMap("GmtCreate")
public String gmtCreate;
/**
* The time when the current entity was updated.
*
* example:
* 1585816811000
*/
@NameInMap("GmtModified")
public String gmtModified;
/**
* The ID of the current entity.
*
* example:
* 1425
*/
@NameInMap("Id")
public String id;
/**
* Indicates whether the entity is a virtual node. Valid values:
*
* - 1: yes
* - 0: no
*
*
* example:
* 1
*/
@NameInMap("IsVirtualNode")
public Integer isVirtualNode;
/**
* The type of the current entity. Valid values include the following values:
*
* - process
* - file
* - alert
* - ip
* - domain
*
*
* example:
* auto-test-policy-name
*/
@NameInMap("Name")
public String name;
/**
* The namespace of the current entity.
*
* example:
* 78
*/
@NameInMap("Namespace")
public String namespace;
/**
* The synchronization ID of the current entity.
*
* example:
* e2fdf402-b4ed-4e1a-9e95-44d6069600b0
*/
@NameInMap("SyncId")
public Integer syncId;
/**
* The tag that indicates whether tracing was successful. Valid values:
*
* - 1: successful
* - 0: failed
*
*
* example:
* 1
*/
@NameInMap("TraceSuccessFlag")
public Integer traceSuccessFlag;
public static QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList self = new QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setCurrentVersionId(String currentVersionId) {
this.currentVersionId = currentVersionId;
return this;
}
public String getCurrentVersionId() {
return this.currentVersionId;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setDisplayColor(String displayColor) {
this.displayColor = displayColor;
return this;
}
public String getDisplayColor() {
return this.displayColor;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setDisplayIcon(String displayIcon) {
this.displayIcon = displayIcon;
return this;
}
public String getDisplayIcon() {
return this.displayIcon;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setDisplayOrder(Integer displayOrder) {
this.displayOrder = displayOrder;
return this;
}
public Integer getDisplayOrder() {
return this.displayOrder;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setDisplayTemplate(String displayTemplate) {
this.displayTemplate = displayTemplate;
return this;
}
public String getDisplayTemplate() {
return this.displayTemplate;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setGmtCreate(String gmtCreate) {
this.gmtCreate = gmtCreate;
return this;
}
public String getGmtCreate() {
return this.gmtCreate;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setGmtModified(String gmtModified) {
this.gmtModified = gmtModified;
return this;
}
public String getGmtModified() {
return this.gmtModified;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setId(String id) {
this.id = id;
return this;
}
public String getId() {
return this.id;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setIsVirtualNode(Integer isVirtualNode) {
this.isVirtualNode = isVirtualNode;
return this;
}
public Integer getIsVirtualNode() {
return this.isVirtualNode;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setNamespace(String namespace) {
this.namespace = namespace;
return this;
}
public String getNamespace() {
return this.namespace;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setSyncId(Integer syncId) {
this.syncId = syncId;
return this;
}
public Integer getSyncId() {
return this.syncId;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailEntityTypeList setTraceSuccessFlag(Integer traceSuccessFlag) {
this.traceSuccessFlag = traceSuccessFlag;
return this;
}
public Integer getTraceSuccessFlag() {
return this.traceSuccessFlag;
}
}
public static class QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList extends TeaModel {
/**
* The version ID of the current relationship.
*
* example:
* 1487
*/
@NameInMap("CurrentVersionId")
public String currentVersionId;
/**
* The direction of the current relationship. Valid values:
*
* - 1: forward
* - 0: reverse
*
*
* example:
* 1
*/
@NameInMap("Directed")
public Integer directed;
/**
* The display color of the current relationship.
*
* example:
* #FFF
*/
@NameInMap("DisplayColor")
public String displayColor;
/**
* The display icon of the current relationship.
*
* example:
* https://img.alicdn.com/imgextra/i2/O1CN01jpZwD31G56XYPEJv2_!!600000000****-55-tps-25-28.svg
*/
@NameInMap("DisplayIcon")
public String displayIcon;
/**
* The display template of the current relationship.
*
* example:
* []
*/
@NameInMap("DisplayTemplate")
public String displayTemplate;
/**
* The time when the current relationship was created.
*
* example:
* 2022-09-23T10:50Z
*/
@NameInMap("GmtCreate")
public String gmtCreate;
/**
* The time when the current relationship was updated.
*
* example:
* 2022-07-12T07:58:49Z
*/
@NameInMap("GmtModified")
public String gmtModified;
/**
* The ID of the current relationship.
*
* example:
* 1514
*/
@NameInMap("Id")
public String id;
/**
* The type of the current relationship. Valid values include the following values:
*
* - process_exec_file: The relationship indicates an executable file that is run by a process.
* - process_connect_ip: The relationship indicates an IP address that is connected by a process.
* - domain_trgger_alert: The relationship indicates an alert that is triggered for a domain name.
*
*
* example:
* wusa
*/
@NameInMap("Name")
public String name;
/**
* The namespace of the current relationship.
*
* example:
* default
*/
@NameInMap("Namespace")
public String namespace;
/**
* The display type of the current relationship.
*
* example:
* 0
*/
@NameInMap("ShowType")
public String showType;
/**
* The synchronization ID of the current relationship.
*
* example:
* sync-0000aws50gyy2ocisbmx
*/
@NameInMap("SyncId")
public Integer syncId;
public static QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList self = new QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setCurrentVersionId(String currentVersionId) {
this.currentVersionId = currentVersionId;
return this;
}
public String getCurrentVersionId() {
return this.currentVersionId;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setDirected(Integer directed) {
this.directed = directed;
return this;
}
public Integer getDirected() {
return this.directed;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setDisplayColor(String displayColor) {
this.displayColor = displayColor;
return this;
}
public String getDisplayColor() {
return this.displayColor;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setDisplayIcon(String displayIcon) {
this.displayIcon = displayIcon;
return this;
}
public String getDisplayIcon() {
return this.displayIcon;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setDisplayTemplate(String displayTemplate) {
this.displayTemplate = displayTemplate;
return this;
}
public String getDisplayTemplate() {
return this.displayTemplate;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setGmtCreate(String gmtCreate) {
this.gmtCreate = gmtCreate;
return this;
}
public String getGmtCreate() {
return this.gmtCreate;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setGmtModified(String gmtModified) {
this.gmtModified = gmtModified;
return this;
}
public String getGmtModified() {
return this.gmtModified;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setId(String id) {
this.id = id;
return this;
}
public String getId() {
return this.id;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setNamespace(String namespace) {
this.namespace = namespace;
return this;
}
public String getNamespace() {
return this.namespace;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setShowType(String showType) {
this.showType = showType;
return this;
}
public String getShowType() {
return this.showType;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailRelationTypeList setSyncId(Integer syncId) {
this.syncId = syncId;
return this;
}
public Integer getSyncId() {
return this.syncId;
}
}
public static class QueryIncidentTracingDetailResponseBodyTracingDetailVertexListDisplayInfo extends TeaModel {
/**
* The name of the property that needs to be displayed for the current node.
*
* example:
* scan:ACSV-2020-111301
*/
@NameInMap("Name")
public String name;
/**
* The value of the property that needs to be displayed for the current node.
*
* example:
* 10.16.1
*/
@NameInMap("Value")
public String value;
public static QueryIncidentTracingDetailResponseBodyTracingDetailVertexListDisplayInfo build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBodyTracingDetailVertexListDisplayInfo self = new QueryIncidentTracingDetailResponseBodyTracingDetailVertexListDisplayInfo();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexListDisplayInfo setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexListDisplayInfo setValue(String value) {
this.value = value;
return this;
}
public String getValue() {
return this.value;
}
}
public static class QueryIncidentTracingDetailResponseBodyTracingDetailVertexListNeighborList extends TeaModel {
/**
* The number of nodes.
*
* example:
* 0
*/
@NameInMap("Count")
public Integer count;
/**
* Indicates whether more nodes are adjacent to the current node. Valid values:
*
* - true
* - false
*
*
* example:
* True
*/
@NameInMap("HasMore")
public Boolean hasMore;
/**
* The type of the node. Valid values include the following values:
*
* - process
* - file
* - alert
* - ip
* - domain
*
*
* example:
* 2
*/
@NameInMap("Type")
public String type;
public static QueryIncidentTracingDetailResponseBodyTracingDetailVertexListNeighborList build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBodyTracingDetailVertexListNeighborList self = new QueryIncidentTracingDetailResponseBodyTracingDetailVertexListNeighborList();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexListNeighborList setCount(Integer count) {
this.count = count;
return this;
}
public Integer getCount() {
return this.count;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexListNeighborList setHasMore(Boolean hasMore) {
this.hasMore = hasMore;
return this;
}
public Boolean getHasMore() {
return this.hasMore;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexListNeighborList setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
}
public static class QueryIncidentTracingDetailResponseBodyTracingDetailVertexList extends TeaModel {
/**
* The ID of the Alibaba Cloud account to which the current node belongs.
*
* example:
* 1487146717137516
*/
@NameInMap("Aliuid")
public String aliuid;
/**
* The display information of the current node.
*/
@NameInMap("DisplayInfo")
public java.util.List displayInfo;
/**
* The ID of the current node.
*
* example:
* 383044
*/
@NameInMap("Id")
public String id;
/**
* The rendering language of the current node.
*
* example:
* zh
*/
@NameInMap("Lang")
public String lang;
/**
* The name of the current node.
*
* example:
* auto-test-attestor
*/
@NameInMap("Name")
public String name;
/**
* The nodes that are adjacent to the current node.
*/
@NameInMap("NeighborList")
public java.util.List neighborList;
/**
* The text that contains the properties of the current node.
*
* example:
* [{\"PropertyValues\": [{\"PropertyValueId\": 239, \"PropertyValue\": \"121\"}, {\"PropertyValueId\": 240, \"PropertyValue\": \"6666\"}], \"PropertyKey\": \"22222222\", \"PropertyId\": 203}]
*/
@NameInMap("Properties")
public String properties;
/**
* The property of the current node.
*
* example:
* {\"coverage\":\"global\"}
*/
@NameInMap("Property")
public java.util.Map property;
/**
* The ID of the rule based on which the current node is generated.
*
* example:
* 301425
*/
@NameInMap("RuleId")
public String ruleId;
/**
* The time when the current node was created.
*
* example:
* 2021-11-26
*/
@NameInMap("Time")
public String time;
/**
* The UNIX timestamp when the current node was created. Unit: milliseconds.
*
* example:
* 1663048980
*/
@NameInMap("Timestamp")
public Long timestamp;
/**
* The type of the current node. Valid values include the following values:
*
* - process
* - file
* - alert
* - ip
* - domain
*
*
* example:
* alidetect
*/
@NameInMap("Type")
public String type;
/**
* The time when the current node was updated.
*
* example:
* 2022-01-13 12:49:33
*/
@NameInMap("UpdateTime")
public String updateTime;
/**
* The UUID of the current node. The security information and event management (SIEM) system generates UUIDs for nodes and edges in the provenance graph to help you locate the nodes or edges.
*
* example:
* 32e36d8a-2b5d-4f71-98a8-12775685a3b4
*/
@NameInMap("Uuid")
public String uuid;
public static QueryIncidentTracingDetailResponseBodyTracingDetailVertexList build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBodyTracingDetailVertexList self = new QueryIncidentTracingDetailResponseBodyTracingDetailVertexList();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setAliuid(String aliuid) {
this.aliuid = aliuid;
return this;
}
public String getAliuid() {
return this.aliuid;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setDisplayInfo(java.util.List displayInfo) {
this.displayInfo = displayInfo;
return this;
}
public java.util.List getDisplayInfo() {
return this.displayInfo;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setId(String id) {
this.id = id;
return this;
}
public String getId() {
return this.id;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setLang(String lang) {
this.lang = lang;
return this;
}
public String getLang() {
return this.lang;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setNeighborList(java.util.List neighborList) {
this.neighborList = neighborList;
return this;
}
public java.util.List getNeighborList() {
return this.neighborList;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setProperties(String properties) {
this.properties = properties;
return this;
}
public String getProperties() {
return this.properties;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setProperty(java.util.Map property) {
this.property = property;
return this;
}
public java.util.Map getProperty() {
return this.property;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setRuleId(String ruleId) {
this.ruleId = ruleId;
return this;
}
public String getRuleId() {
return this.ruleId;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setTime(String time) {
this.time = time;
return this;
}
public String getTime() {
return this.time;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setTimestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
public Long getTimestamp() {
return this.timestamp;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
public String getUpdateTime() {
return this.updateTime;
}
public QueryIncidentTracingDetailResponseBodyTracingDetailVertexList setUuid(String uuid) {
this.uuid = uuid;
return this;
}
public String getUuid() {
return this.uuid;
}
}
public static class QueryIncidentTracingDetailResponseBodyTracingDetail extends TeaModel {
/**
* The edges.
*/
@NameInMap("EdgeList")
public java.util.List edgeList;
/**
* The entities.
*/
@NameInMap("EntityTypeList")
public java.util.List entityTypeList;
/**
* The rendering language of the returned result. Valid values:
*
* - zh: Chinese
* - en: English
*
*
* example:
* zh
*/
@NameInMap("Lang")
public String lang;
/**
* The relationships.
*/
@NameInMap("RelationTypeList")
public java.util.List relationTypeList;
/**
* The nodes.
*/
@NameInMap("VertexList")
public java.util.List vertexList;
public static QueryIncidentTracingDetailResponseBodyTracingDetail build(java.util.Map map) throws Exception {
QueryIncidentTracingDetailResponseBodyTracingDetail self = new QueryIncidentTracingDetailResponseBodyTracingDetail();
return TeaModel.build(map, self);
}
public QueryIncidentTracingDetailResponseBodyTracingDetail setEdgeList(java.util.List edgeList) {
this.edgeList = edgeList;
return this;
}
public java.util.List getEdgeList() {
return this.edgeList;
}
public QueryIncidentTracingDetailResponseBodyTracingDetail setEntityTypeList(java.util.List entityTypeList) {
this.entityTypeList = entityTypeList;
return this;
}
public java.util.List getEntityTypeList() {
return this.entityTypeList;
}
public QueryIncidentTracingDetailResponseBodyTracingDetail setLang(String lang) {
this.lang = lang;
return this;
}
public String getLang() {
return this.lang;
}
public QueryIncidentTracingDetailResponseBodyTracingDetail setRelationTypeList(java.util.List relationTypeList) {
this.relationTypeList = relationTypeList;
return this;
}
public java.util.List getRelationTypeList() {
return this.relationTypeList;
}
public QueryIncidentTracingDetailResponseBodyTracingDetail setVertexList(java.util.List vertexList) {
this.vertexList = vertexList;
return this;
}
public java.util.List getVertexList() {
return this.vertexList;
}
}
}