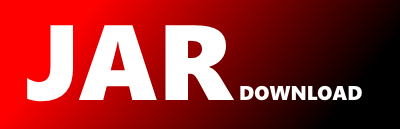
com.aliyun.sas20181203.models.StopHoneypotResponseBody Maven / Gradle / Ivy
Show all versions of sas20181203 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sas20181203.models;
import com.aliyun.tea.*;
public class StopHoneypotResponseBody extends TeaModel {
/**
* The response code. The status code 200 indicates that the request was successful. Other status codes indicate that the request failed. You can identify the cause of the failure based on the status code.
*
* example:
* 200
*/
@NameInMap("Code")
public String code;
/**
* The data returned.
*/
@NameInMap("Data")
public StopHoneypotResponseBodyData data;
/**
* The HTTP status code.
*
* example:
* 200
*/
@NameInMap("HttpStatusCode")
public Integer httpStatusCode;
/**
* The returned message.
*
* example:
* successful
*/
@NameInMap("Message")
public String message;
/**
* The request ID.
*
* example:
* D65AADFC-1D20-5A6A-8F6A-9FA53C*****
*/
@NameInMap("RequestId")
public String requestId;
/**
* Indicates whether the request was successful. Valid values:
*
* - true
* - false
*
*
* example:
* true
*/
@NameInMap("Success")
public Boolean success;
public static StopHoneypotResponseBody build(java.util.Map map) throws Exception {
StopHoneypotResponseBody self = new StopHoneypotResponseBody();
return TeaModel.build(map, self);
}
public StopHoneypotResponseBody setCode(String code) {
this.code = code;
return this;
}
public String getCode() {
return this.code;
}
public StopHoneypotResponseBody setData(StopHoneypotResponseBodyData data) {
this.data = data;
return this;
}
public StopHoneypotResponseBodyData getData() {
return this.data;
}
public StopHoneypotResponseBody setHttpStatusCode(Integer httpStatusCode) {
this.httpStatusCode = httpStatusCode;
return this;
}
public Integer getHttpStatusCode() {
return this.httpStatusCode;
}
public StopHoneypotResponseBody setMessage(String message) {
this.message = message;
return this;
}
public String getMessage() {
return this.message;
}
public StopHoneypotResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public StopHoneypotResponseBody setSuccess(Boolean success) {
this.success = success;
return this;
}
public Boolean getSuccess() {
return this.success;
}
public static class StopHoneypotResponseBodyData extends TeaModel {
/**
* The name of the management node to which the honeypot belongs.
*
* example:
* managerNoden****
*/
@NameInMap("ControlNodeName")
public String controlNodeName;
/**
* The ID of the honeypot.
*
* example:
* 9bf8cd373112263d4bc102fc5dba9d9f812ee05d4d35c487d330d52e937f****
*/
@NameInMap("HoneypotId")
public String honeypotId;
/**
* The display name of the image.
*
* example:
* RuoYi
*/
@NameInMap("HoneypotImageDisplayName")
public String honeypotImageDisplayName;
/**
* The name of the image that is used for the honeypot.
*
* example:
* metabase
*/
@NameInMap("HoneypotImageName")
public String honeypotImageName;
/**
* The name of the honeypot.
*
* example:
* hyl-phpmya****
*/
@NameInMap("HoneypotName")
public String honeypotName;
/**
* The ID of the management node.
*
* example:
* a882e590-b87b-45a6-87b9-d0a3e5a0****
*/
@NameInMap("NodeId")
public String nodeId;
/**
* The ID of the honeypot custom parameter.
*
* example:
* 868a7579-00b5-4a74-999d-8bd3f411****
*/
@NameInMap("PresetId")
public String presetId;
/**
* The statuses of the honeypots.
*/
@NameInMap("State")
public java.util.List state;
public static StopHoneypotResponseBodyData build(java.util.Map map) throws Exception {
StopHoneypotResponseBodyData self = new StopHoneypotResponseBodyData();
return TeaModel.build(map, self);
}
public StopHoneypotResponseBodyData setControlNodeName(String controlNodeName) {
this.controlNodeName = controlNodeName;
return this;
}
public String getControlNodeName() {
return this.controlNodeName;
}
public StopHoneypotResponseBodyData setHoneypotId(String honeypotId) {
this.honeypotId = honeypotId;
return this;
}
public String getHoneypotId() {
return this.honeypotId;
}
public StopHoneypotResponseBodyData setHoneypotImageDisplayName(String honeypotImageDisplayName) {
this.honeypotImageDisplayName = honeypotImageDisplayName;
return this;
}
public String getHoneypotImageDisplayName() {
return this.honeypotImageDisplayName;
}
public StopHoneypotResponseBodyData setHoneypotImageName(String honeypotImageName) {
this.honeypotImageName = honeypotImageName;
return this;
}
public String getHoneypotImageName() {
return this.honeypotImageName;
}
public StopHoneypotResponseBodyData setHoneypotName(String honeypotName) {
this.honeypotName = honeypotName;
return this;
}
public String getHoneypotName() {
return this.honeypotName;
}
public StopHoneypotResponseBodyData setNodeId(String nodeId) {
this.nodeId = nodeId;
return this;
}
public String getNodeId() {
return this.nodeId;
}
public StopHoneypotResponseBodyData setPresetId(String presetId) {
this.presetId = presetId;
return this;
}
public String getPresetId() {
return this.presetId;
}
public StopHoneypotResponseBodyData setState(java.util.List state) {
this.state = state;
return this;
}
public java.util.List getState() {
return this.state;
}
}
}