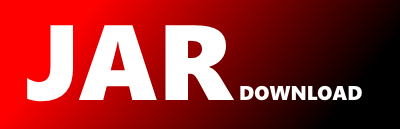
com.aliyun.sddp20190103.models.DescribeOssObjectsResponseBody Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sddp20190103.models;
import com.aliyun.tea.*;
public class DescribeOssObjectsResponseBody extends TeaModel {
/**
* The page number of the returned page.
*
* example:
* 1
*/
@NameInMap("CurrentPage")
public Integer currentPage;
/**
* A list of OSS objects.
*/
@NameInMap("Items")
public java.util.List items;
@NameInMap("Marker")
public String marker;
@NameInMap("NextMarker")
public String nextMarker;
/**
* The number of entries returned per page.
*
* example:
* 12
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The ID of the request.
*
* example:
* 769FB3C1-F4C9-42DF-9B72-7077A8989C13
*/
@NameInMap("RequestId")
public String requestId;
/**
* The total number of entries returned.
*
* example:
* 1
*/
@NameInMap("TotalCount")
public Integer totalCount;
@NameInMap("Truncated")
public Boolean truncated;
public static DescribeOssObjectsResponseBody build(java.util.Map map) throws Exception {
DescribeOssObjectsResponseBody self = new DescribeOssObjectsResponseBody();
return TeaModel.build(map, self);
}
public DescribeOssObjectsResponseBody setCurrentPage(Integer currentPage) {
this.currentPage = currentPage;
return this;
}
public Integer getCurrentPage() {
return this.currentPage;
}
public DescribeOssObjectsResponseBody setItems(java.util.List items) {
this.items = items;
return this;
}
public java.util.List getItems() {
return this.items;
}
public DescribeOssObjectsResponseBody setMarker(String marker) {
this.marker = marker;
return this;
}
public String getMarker() {
return this.marker;
}
public DescribeOssObjectsResponseBody setNextMarker(String nextMarker) {
this.nextMarker = nextMarker;
return this;
}
public String getNextMarker() {
return this.nextMarker;
}
public DescribeOssObjectsResponseBody setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public DescribeOssObjectsResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeOssObjectsResponseBody setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
public DescribeOssObjectsResponseBody setTruncated(Boolean truncated) {
this.truncated = truncated;
return this;
}
public Boolean getTruncated() {
return this.truncated;
}
public static class DescribeOssObjectsResponseBodyItemsRuleList extends TeaModel {
/**
* The number of times that the rule is hit.
*
* example:
* 100
*/
@NameInMap("Count")
public Long count;
/**
* The search keyword. Fuzzy match is supported.
*
* example:
* ID card
*/
@NameInMap("Name")
public String name;
/**
* The ID of the sensitivity level of the OSS object. Valid values:
*
* - 1: N/A, which indicates that no sensitive data is detected.
* - 2: S1, which indicates the low sensitivity level.
* - 3: S2, which indicates the medium sensitivity level.
* - 4: S3, which indicates the high sensitivity level.
* - 5: S4, which indicates the highest sensitivity level.
*
*
* example:
* 2
*/
@NameInMap("RiskLevelId")
public Long riskLevelId;
public static DescribeOssObjectsResponseBodyItemsRuleList build(java.util.Map map) throws Exception {
DescribeOssObjectsResponseBodyItemsRuleList self = new DescribeOssObjectsResponseBodyItemsRuleList();
return TeaModel.build(map, self);
}
public DescribeOssObjectsResponseBodyItemsRuleList setCount(Long count) {
this.count = count;
return this;
}
public Long getCount() {
return this.count;
}
public DescribeOssObjectsResponseBodyItemsRuleList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public DescribeOssObjectsResponseBodyItemsRuleList setRiskLevelId(Long riskLevelId) {
this.riskLevelId = riskLevelId;
return this;
}
public Long getRiskLevelId() {
return this.riskLevelId;
}
}
public static class DescribeOssObjectsResponseBodyItems extends TeaModel {
/**
* The name of the bucket.
*
* example:
* oss-duplicate-***
*/
@NameInMap("BucketName")
public String bucketName;
/**
* The type of the OSS object. Valid values include 900001, 800015, or 800005, which indicates the MP4 file, PDF file, or OSS configuration file, respectively.
*
* example:
* 900001
*/
@NameInMap("Category")
public Long category;
/**
* The name of the file type.
*
* example:
* MP4 file
*/
@NameInMap("CategoryName")
public String categoryName;
/**
* The code of the file type.
*
* example:
* 1
*/
@NameInMap("FileCategoryCode")
public Long fileCategoryCode;
/**
* The name of the file type.
*
* example:
* text file
*/
@NameInMap("FileCategoryName")
public String fileCategoryName;
/**
* The file ID of the OSS object.
*
* example:
* file-22***
*/
@NameInMap("FileId")
public String fileId;
/**
* The ID of the OSS object.
*
* example:
* 17383
*/
@NameInMap("Id")
public String id;
/**
* The ID of the instance to which the OSS object belongs.
*
* example:
* 1232122
*/
@NameInMap("InstanceId")
public Long instanceId;
/**
* The time when the file was last modified.
*
* example:
* 1536751124000
*/
@NameInMap("LastModifiedTime")
public Long lastModifiedTime;
/**
* The name of the OSS object.
*
* example:
* obj_id
*/
@NameInMap("Name")
public String name;
/**
* The region ID of the OSS object.
*
* example:
* cn-***
*/
@NameInMap("RegionId")
public String regionId;
/**
* The ID of the sensitivity level of the OSS object. Valid values:
*
* - 1: N/A, which indicates that no sensitive data is detected.
* - 2: S1, which indicates the low sensitivity level.
* - 3: S2, which indicates the medium sensitivity level.
* - 4: S3, which indicates the high sensitivity level.
* - 5: S4, which indicates the highest sensitivity level.
*
*
* example:
* 2
*/
@NameInMap("RiskLevelId")
public Long riskLevelId;
/**
* The name of the sensitivity level for the OSS object.
*
* example:
* Medium sensitivity level
*/
@NameInMap("RiskLevelName")
public String riskLevelName;
/**
* The number of rules that are hit.
*
* example:
* 100
*/
@NameInMap("RuleCount")
public Integer ruleCount;
/**
* A list of rules.
*/
@NameInMap("RuleList")
public java.util.List ruleList;
/**
* The number of fields that are hit.
*
* example:
* 50
*/
@NameInMap("SensitiveCount")
public Integer sensitiveCount;
/**
* The size of the file. Unit: bytes.
*
* example:
* 20
*/
@NameInMap("Size")
public Long size;
public static DescribeOssObjectsResponseBodyItems build(java.util.Map map) throws Exception {
DescribeOssObjectsResponseBodyItems self = new DescribeOssObjectsResponseBodyItems();
return TeaModel.build(map, self);
}
public DescribeOssObjectsResponseBodyItems setBucketName(String bucketName) {
this.bucketName = bucketName;
return this;
}
public String getBucketName() {
return this.bucketName;
}
public DescribeOssObjectsResponseBodyItems setCategory(Long category) {
this.category = category;
return this;
}
public Long getCategory() {
return this.category;
}
public DescribeOssObjectsResponseBodyItems setCategoryName(String categoryName) {
this.categoryName = categoryName;
return this;
}
public String getCategoryName() {
return this.categoryName;
}
public DescribeOssObjectsResponseBodyItems setFileCategoryCode(Long fileCategoryCode) {
this.fileCategoryCode = fileCategoryCode;
return this;
}
public Long getFileCategoryCode() {
return this.fileCategoryCode;
}
public DescribeOssObjectsResponseBodyItems setFileCategoryName(String fileCategoryName) {
this.fileCategoryName = fileCategoryName;
return this;
}
public String getFileCategoryName() {
return this.fileCategoryName;
}
public DescribeOssObjectsResponseBodyItems setFileId(String fileId) {
this.fileId = fileId;
return this;
}
public String getFileId() {
return this.fileId;
}
public DescribeOssObjectsResponseBodyItems setId(String id) {
this.id = id;
return this;
}
public String getId() {
return this.id;
}
public DescribeOssObjectsResponseBodyItems setInstanceId(Long instanceId) {
this.instanceId = instanceId;
return this;
}
public Long getInstanceId() {
return this.instanceId;
}
public DescribeOssObjectsResponseBodyItems setLastModifiedTime(Long lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
return this;
}
public Long getLastModifiedTime() {
return this.lastModifiedTime;
}
public DescribeOssObjectsResponseBodyItems setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public DescribeOssObjectsResponseBodyItems setRegionId(String regionId) {
this.regionId = regionId;
return this;
}
public String getRegionId() {
return this.regionId;
}
public DescribeOssObjectsResponseBodyItems setRiskLevelId(Long riskLevelId) {
this.riskLevelId = riskLevelId;
return this;
}
public Long getRiskLevelId() {
return this.riskLevelId;
}
public DescribeOssObjectsResponseBodyItems setRiskLevelName(String riskLevelName) {
this.riskLevelName = riskLevelName;
return this;
}
public String getRiskLevelName() {
return this.riskLevelName;
}
public DescribeOssObjectsResponseBodyItems setRuleCount(Integer ruleCount) {
this.ruleCount = ruleCount;
return this;
}
public Integer getRuleCount() {
return this.ruleCount;
}
public DescribeOssObjectsResponseBodyItems setRuleList(java.util.List ruleList) {
this.ruleList = ruleList;
return this;
}
public java.util.List getRuleList() {
return this.ruleList;
}
public DescribeOssObjectsResponseBodyItems setSensitiveCount(Integer sensitiveCount) {
this.sensitiveCount = sensitiveCount;
return this;
}
public Integer getSensitiveCount() {
return this.sensitiveCount;
}
public DescribeOssObjectsResponseBodyItems setSize(Long size) {
this.size = size;
return this;
}
public Long getSize() {
return this.size;
}
}
}