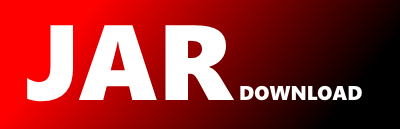
redis.clients.util.Slowlog Maven / Gradle / Ivy
package redis.clients.util;
import java.util.ArrayList;
import java.util.List;
public class Slowlog {
private final long id;
private final long timeStamp;
private final long executionTime;
private final List args;
@SuppressWarnings("unchecked")
public static List from(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy