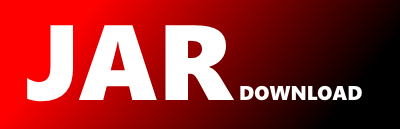
redis.clients.jedis.ShardedJedisPipeline Maven / Gradle / Ivy
The newest version!
package redis.clients.jedis;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Queue;
public class ShardedJedisPipeline extends PipelineBase {
private BinaryShardedJedis jedis;
private List results = new ArrayList();
private Queue clients = new LinkedList();
private static class FutureResult {
private Client client;
public FutureResult(Client client) {
this.client = client;
}
public Object get() {
return client.getOne();
}
}
public void setShardedJedis(BinaryShardedJedis jedis) {
this.jedis = jedis;
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy