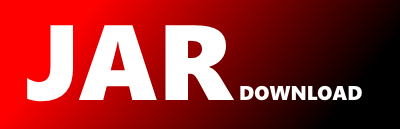
com.almende.eve.agent.AgentCache Maven / Gradle / Ivy
package com.almende.eve.agent;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import com.almende.eve.config.Config;
public class AgentCache {
private static int maxSize = 100;
private static Map cache = new ConcurrentHashMap(maxSize);
protected static List scores = new ArrayList(maxSize);
public static void configCache(Config config){
synchronized(cache){
Integer maxSize = config.get("AgentCache","maxSize");
if (maxSize != null) AgentCache.maxSize=maxSize;
//System.err.println("Init AgentCache on maxSize:"+this.maxSize);
AgentCache.cache = new ConcurrentHashMap(AgentCache.maxSize+1);
AgentCache.scores = new ArrayList(AgentCache.maxSize);
}
}
public static Agent get(String agentId){
MetaInfo result = cache.get(agentId);
if (result != null){
result.use();
//System.err.println("Got:"+result.agent.getId());
return result.agent;
}
return null;
}
public static void put(String agentId, Agent agent){
synchronized(cache){
MetaInfo entry = new MetaInfo(agent);
cache.put(agentId, entry);
int overshoot = cache.size()-maxSize;
if (overshoot > 0){
evict(overshoot);
}
scores.add(entry);
//System.err.println("Added:"+agent.getId());
}
}
static protected void evict(int amount){
synchronized(cache){
Collections.sort(scores);
ArrayList toEvict = new ArrayList(amount);
for (int i=0; i) scores.subList(amount, scores.size());
for (MetaInfo entry: toEvict){
cache.remove(entry.agent.getId());
}
//System.err.println("Evicted:"+amount+" records");
}
}
static public void delete(String agentId) {
cache.remove(agentId);
}
}
class MetaInfo implements Comparable {
Agent agent;
int count=0;
long created;
public MetaInfo(Agent agent){
created = System.currentTimeMillis();
this.agent = agent;
}
public void use(){
count++;
}
public long getAge(){
return (System.currentTimeMillis()-created);
}
public double score(){
return count/getAge();
}
@Override
public int compareTo(MetaInfo o) {
return Double.compare(score(), o.score());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy