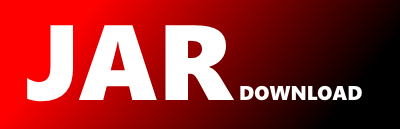
com.almende.eve.state.FileState Maven / Gradle / Ivy
package com.almende.eve.state;
import java.io.Serializable;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
/**
* @class FileState
*
* A persistent state for an Eve Agent, which stores the data on disk.
* Data is stored in the path provided by the configuration file.
*
* The state provides general information for the agent (about itself,
* the environment, and the system configuration), and the agent can store
* persistent data in the state.
* The state extends a standard Java Map.
*
* Usage:
* AgentFactory factory = new AgentFactory(config);
* State state = new State("agentId");
* state.put("key", "value");
* System.out.println(state.get("key")); // "value"
*
* @author jos
*/
public abstract class FileState extends AbstractState {
protected FileState() {}
public FileState(String agentId) {
super(agentId);
}
/**
* init is executed once before the agent method is invoked
*/
@Override
abstract public void init();
/**
* destroy is executed once after the agent method is invoked
* if the properties are changed, they will be saved
*/
@Override
abstract public void destroy();
@Override
abstract public void clear();
@Override
abstract public Set keySet();
@Override
abstract public boolean containsKey(Object key);
@Override
abstract public boolean containsValue(Object value);
@Override
abstract public Set> entrySet();
@Override
abstract public Serializable get(Object key);
@Override
abstract public boolean isEmpty();
@Override
abstract public Serializable put(String key, Serializable value);
@Override
abstract public void putAll(Map extends String, ? extends Serializable> map);
@Override
abstract public Serializable remove(Object key);
@Override
abstract public int size();
@Override
abstract public Collection values();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy