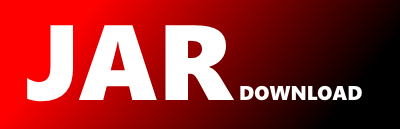
com.almende.eve.agent.callback.AsyncCallbackQueue Maven / Gradle / Ivy
/*
* Copyright: Almende B.V. (2014), Rotterdam, The Netherlands
* License: The Apache Software License, Version 2.0
*/
package com.almende.eve.agent.callback;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import com.almende.eve.config.Config;
/**
* Queue to hold a list with callbacks in progress.
* The Queue handles timeouts on the callbacks.
*
* @param
* the generic type
*/
public class AsyncCallbackQueue {
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy