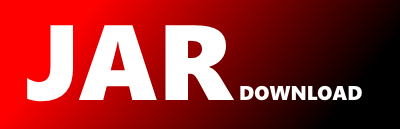
com.almende.eve.agent.log.EventLogger Maven / Gradle / Ivy
/*
* Copyright: Almende B.V. (2014), Rotterdam, The Netherlands
* License: The Apache Software License, Version 2.0
*/
package com.almende.eve.agent.log;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.almende.eve.agent.AgentHostDefImpl;
import com.almende.eve.rpc.jsonrpc.JSONRPCException;
/**
* The Class EventLogger.
*/
public class EventLogger {
private static final Logger LOG = Logger.getLogger(EventLogger.class
.getCanonicalName());
private AgentHostDefImpl agentHost = null;
/**
* Instantiates a new event logger.
*/
protected EventLogger() {
}
/**
* Instantiates a new event logger.
*
* @param agentHost the agent host
*/
public EventLogger(final AgentHostDefImpl agentHost) {
this.agentHost = agentHost;
}
/**
* Log.
*
* @param agentId the agent id
* @param event the event
* @param params the params
*/
public void log(final String agentId, final String event, final Object params) {
try {
final String logAgentId = getLogAgentId(agentId);
final LogAgent agent = (LogAgent) agentHost.getAgent(logAgentId);
if (agent != null) {
// log only if the log agent exists
agent.log(new Log(agentId, event, params));
}
} catch (final Exception e) {
LOG.log(Level.WARNING, "", e);
}
}
/**
* Gets the logs.
*
* @param agentId the agent id
* @param since the since
* @return the logs
* @throws JSONRPCException the jSONRPC exception
* @throws ClassNotFoundException the class not found exception
* @throws InstantiationException the instantiation exception
* @throws IllegalAccessException the illegal access exception
* @throws InvocationTargetException the invocation target exception
* @throws NoSuchMethodException the no such method exception
* @throws IOException Signals that an I/O exception has occurred.
*/
public List getLogs(final String agentId, final Long since)
throws JSONRPCException, ClassNotFoundException,
InstantiationException, IllegalAccessException,
InvocationTargetException, NoSuchMethodException, IOException {
final String logAgentId = getLogAgentId(agentId);
LogAgent agent = (LogAgent) agentHost.getAgent(logAgentId);
if (agent == null) {
// create the log agent if it does not yet exist
agent = agentHost
.createAgent(LogAgent.class, logAgentId);
agent.config(agentHost.getAgent(agentId).getFirstUrl());
}
return agent.getLogs(since);
}
/**
* Gets the log agent id.
*
* @param agentId the agent id
* @return the log agent id
*/
private String getLogAgentId(final String agentId) {
// TODO: use a naming here which cannot conflict with other agents.
// introduce a separate namespace or something like that?
return "_logagent_" + agentId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy