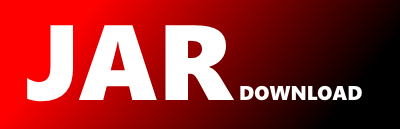
com.almworks.integers.mojo.GenerateMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of generator-maven-plugin
Show all versions of generator-maven-plugin
Maven plugin to generate java code from templates
The newest version!
package com.almworks.integers.mojo;
import com.almworks.integers.generator.IntegerCollectionsCodegen;
import org.apache.maven.plugin.*;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.*;
import org.apache.maven.project.MavenProject;
import java.io.*;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.*;
import java.util.regex.*;
@Mojo( name = "generate-java")
public class GenerateMojo extends AbstractMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
/**
* Root templates directory
*/
@Parameter(name="templates", defaultValue = "${project.basedir}/src/main/templates")
private File templates;
/**
* If set to true and {@link #templates} directory is empty the build fails.
*/
@Parameter(name = "failIfNoTemplates", defaultValue = "true")
private boolean failIfNoTemplates;
/**
* Template file extension
*/
@Parameter(name="file-ext", defaultValue = "tpl")
private String fileExt;
/**
* Output directory for generated java sources
*/
@Parameter(name = "output", defaultValue = "${project.build.directory}/gen-java")
private File output;
/**
* If true - do not generate java source, just check.
* If false - generate java sources.
*/
@Parameter(name = "check-only", defaultValue = "false")
private boolean checkOnly;
private static final Pattern FILE_EXT = Pattern.compile("^.*\\.([^.]+)$");
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
boolean runMojo = checkParameters();
cleanTarget();
if (!runMojo) return;
List templatePaths = new ArrayList<>();
Path templatesRoot = templates.toPath();
try {
getLog().info(String.format("Collect template files from [%s]", templatesRoot));
Files.walkFileTree(templatesRoot, new SimpleFileVisitor() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
Matcher m = FILE_EXT.matcher(file.getFileName().toString());
if (m.matches() && fileExt.equals(m.group(1))) templatePaths.add(file);
return FileVisitResult.CONTINUE;
}
});
} catch (Throwable e) {
throw new MojoExecutionException("Failed to collect templates", e);
}
if (templatePaths.isEmpty()) throw new MojoFailureException(String.format("No templates with extension [%s] found in [%s]", fileExt, templates));
getLog().debug(String.format("%s templates collected", templatePaths.size()));
StringBuilder templatePathsBuilder = new StringBuilder();
for (Path path : templatePaths) {
if (templatePathsBuilder.length() > 0) templatePathsBuilder.append(File.pathSeparator);
String relative = templatesRoot.relativize(path).toString();
templatePathsBuilder.append(relative);
getLog().info(" " + relative);
}
IntegerCollectionsCodegen.main(new String[]{templates.getPath(), templatePathsBuilder.toString(), output.getPath(), String.valueOf(checkOnly)});
project.addCompileSourceRoot(output.getPath());
}
/**
* Checks mojo parameters and creates directories
* @return true if parameters are valid and directories are created
* false if execution should be cancelled without failure
* @throws MojoFailureException if parameters are not valid and mojo must fail
*/
private boolean checkParameters() throws MojoFailureException {
if (templates == null) throw new MojoFailureException("Missing 'templates' parameter");
if (!Files.isDirectory(templates.toPath()))
if (failIfNoTemplates)
throw new MojoFailureException(String.format("Templates directory does not exist [%s]", templates));
else return false;
fileExt = fileExt != null ? fileExt.trim() : "";
if (fileExt.isEmpty()) throw new MojoFailureException("Missing 'fileExt' parameter");
if (output == null) throw new MojoFailureException("Missing 'output' parameter");
if (!Files.exists(output.toPath()))
if (!output.mkdirs())throw new MojoFailureException(String.format("Failed to create directory [%s]", output));
if (!Files.isDirectory(output.toPath())) throw new MojoFailureException(String.format("Output is not a directory [%s]", output));
return true;
}
private void cleanTarget() throws MojoExecutionException {
Path root = output.toPath();
if (!Files.exists(root)) return;
try {
Files.walkFileTree(root, new SimpleFileVisitor() {
@Override
public FileVisitResult postVisitDirectory(Path dir, IOException exc) throws IOException {
if (!root.equals(dir)) Files.delete(dir);
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
Files.delete(file);
return FileVisitResult.CONTINUE;
}
});
} catch (IOException e) {
throw new MojoExecutionException("Failed to clean output directory", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy