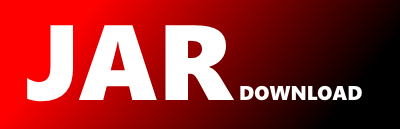
com.almworks.jira.structure.api.StructureError Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api;
import com.almworks.jira.structure.api2g.item.ItemIdentity;
/**
* {@code StructureError} is an enumeration of all possible error types
* in the Structure layer. Each error is supplemented by numeric code, which
* may be used to define localizable error messages.
*
* {@code StructureError} may also be used to start a construction of {@code StructureException}
* - see {@link StructureException}.
*
* @see StructureException
*/
public enum StructureError {
// === Generic Errors ===
GENERIC_ERROR(1000),
JIRA_DATA_ACCESS_EXCEPTION(1001),
SEARCH_ERROR(1002),
LICENSE_PROBLEM(1003),
LOCK_FAILED(1004),
CONCURRENT_UPDATE_FAILED(1005),
// === Bad Request ===
INVALID_JQL(2001),
INVALID_FILTER_ID(2002),
INACCESSIBLE_FILTER(2003),
INVALID_SJQL(2004),
// todo this only makes sense for Structure Pages. Have extensible StructureError?
INVALID_CQL(2010),
// Permission rules-related
PERMISSION_RULE_APPLICATION_FORMS_CYCLE(2100),
GROUP_NOT_EXISTS_OR_INACCESSIBLE(2101),
PROJECT_NOT_EXISTS_OR_INACCESSIBLE(2102),
PROJECT_ROLE_NOT_EXISTS_OR_INACCESSIBLE(2103),
USER_NOT_EXISTS_OR_INACCESIBLE(2104),
APPLY_RULE_NOT_APPLICABLE(2105),
// views
INVALID_VIEW_PROPERTIES(2201),
// perspectives
INVALID_PERSPECTIVE_PROPERTIES(2301),
// === Permissions Related ===
ISSUE_NOT_EXISTS_OR_NOT_ACCESSIBLE(4001),
FOREST_CHANGE_PROHIBITED_BY_PARENT_PERMISSIONS(4002),
PROJECT_NOT_ENABLED_FOR_STRUCTURE(4003),
ISSUE_NOT_EDITABLE(4004),
STRUCTURE_NOT_EXISTS_OR_NOT_ACCESSIBLE(4005),
NOT_ALLOWED_TO_CREATE_STRUCTURE(4006),
STRUCTURE_ADMIN_DENIED(4007),
STRUCTURE_EDIT_DENIED(4008),
JIRA_ADMIN_DENIED(4009),
STRUCTURE_PLUGIN_ACCESS_DENIED(4010),
VERSION_NOT_EXISTS_OR_NOT_ACCESSIBLE(4011),
ARCHIVED_STRUCTURE_EDIT_DENIED(4012),
STRUCTURE_OWNER_EDIT_DENIED(4013),
VIEW_NOT_EXISTS_OR_NOT_ACCESSIBLE(4101),
VIEW_ADMIN_DENIED(4102),
VIEW_EDIT_DENIED(4103),
VIEW_CREATION_DENIED(4104),
PERSPECTIVE_CREATION_DENIED(4201),
ACTING_USER_NOT_FOUND(4301),
// === Integrity Related ===
ROW_MISSING_FROM_STRUCTURE(5001),
INVALID_MOVE(5002),
INVALID_OPERATION(5003),
UNAVAILABLE_ITEM_TYPE(5004),
// StructureException refactoring pending.
// Proposed general error categories:
INVALID_PARAMETER(400),
PERMISSION_DENIED(403),
ITEM_NOT_FOUND(404),
INTERNAL_ERROR(500)
;
private final int myCode;
StructureError(int code) {
myCode = code;
}
/**
* @return error code
*/
public int getCode() {
return myCode;
}
/**
* @return true if this error is related to permissions
*/
public boolean isPermissionError() {
return myCode / 1000 == 4 || this == PERMISSION_DENIED;
}
public boolean isNotFoundError() {
return this == STRUCTURE_NOT_EXISTS_OR_NOT_ACCESSIBLE || this == VIEW_NOT_EXISTS_OR_NOT_ACCESSIBLE || this == ITEM_NOT_FOUND;
}
public StructureException.Builder builder() {
return new StructureException.Builder(this);
}
public StructureException withLocalizedMessage(String messageKey, Object... messageParameters) {
return builder().withLocalizedMessage(messageKey, messageParameters);
}
public StructureException withMessage(String message) {
return builder().withMessage(message);
}
public StructureException withoutMessage() {
return builder().withoutMessage();
}
public StructureException.Builder forStructure(Long structure) {
return builder().forStructure(structure);
}
public StructureException.Builder forView(Long view) {
return builder().forView(view);
}
// todo review usage and introduce forRow(long), OR review StructureError/StructureException
public StructureException.Builder forIssue(Long issue) {
return builder().forIssue(issue);
}
public StructureException.Builder forItem(ItemIdentity itemId) {
return builder().forItem(itemId);
}
public StructureException.Builder causedBy(Throwable cause) {
return builder().causedBy(cause);
}
public String toString() {
return super.toString() + "[" + myCode + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy