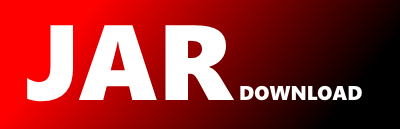
com.almworks.jira.structure.api.UserCompat Maven / Gradle / Ivy
Show all versions of structure-api Show documentation
package com.almworks.jira.structure.api;
import com.atlassian.crowd.embedded.api.User;
import com.atlassian.jira.user.ApplicationUser;
import com.atlassian.jira.user.ApplicationUsers;
import org.jetbrains.annotations.Nullable;
import java.lang.reflect.Method;
/**
* This class is created in order to be able to have single source code for JIRA 7 and JIRA 6 versions of the product.
*
* Most JIRA 6.3 APIs that take {@code User} as a parameter also have overloaded methods that take {@code ApplicationUser}.
* In JIRA 7.0, {@code User} is dropped, but we can switch to using {@code ApplicationUser} and have that code work with
* JIRA 6.3 and JIRA 7.0.
*
* However, some methods with {@code User} parameter do not have an overloaded duplicate in JIRA 6.3, and in JIRA 7.0
* they just changed it to {@code ApplicationUser}. This makes it impossible to have a single binary for JIRA 6 and 7,
* unless we use reflection every time.
*
* However, we can have a single source code, thanks to this class. During compilation, the appropriate method
* signature is chosen based on what API is used.
*
* @author che
*/
public class UserCompat implements ApplicationUser, User {
// not null in JIRA 7
@Nullable
private static final Method getIdMethod = getGetIdMethod();
private final ApplicationUser myApplicationUser;
private final User myUser;
private UserCompat(ApplicationUser user) {
myApplicationUser = user;
myUser = user.getDirectoryUser();
}
private static Method getGetIdMethod() {
try {
return ApplicationUser.class.getMethod("getId");
} catch (NoSuchMethodException e) {
return null;
}
}
@Override
public String getKey() {
return myApplicationUser.getKey();
}
@Override
public String getUsername() {
return myApplicationUser.getUsername();
}
@Override
public String getName() {
return myApplicationUser.getName();
}
@Override
public long getDirectoryId() {
return myApplicationUser.getDirectoryId();
}
@Override
public boolean isActive() {
return myApplicationUser.isActive();
}
@Override
public String getEmailAddress() {
return myApplicationUser.getEmailAddress();
}
@Override
public String getDisplayName() {
return myApplicationUser.getDisplayName();
}
@Override
public int compareTo(User user) {
return myUser.compareTo(user);
}
@Override
public User getDirectoryUser() {
return myUser;
}
//@Override only in JIRA 7
public Long getId() {
if (getIdMethod == null) return null;
try {
return (Long) getIdMethod.invoke(myApplicationUser);
} catch (Exception e) {
return null;
}
}
@Override
public int hashCode() {
return myApplicationUser.hashCode();
}
@Override
public boolean equals(Object obj) {
if (obj == null) return false;
if (obj == this) return true;
if (obj instanceof ApplicationUser) {
return equalUser(this, (ApplicationUser) obj);
} else if (obj instanceof User) {
ApplicationUser user = ApplicationUsers.from((User) obj);
return equalUser(this, user);
} else {
return false;
}
}
private boolean equalUser(ApplicationUser u1, ApplicationUser u2) {
String k1 = u1.getKey();
String k2 = u2.getKey();
return k1 == null ? k2 == null : k1.equals(k2);
}
public static UserCompat from(User user) {
if (user == null) return null;
return new UserCompat(ApplicationUsers.from(user));
}
public static UserCompat from(ApplicationUser applicationUser) {
if (applicationUser == null) return null;
return new UserCompat(applicationUser);
}
public static UserCompat fromObject(Object userObject) {
if (userObject instanceof User) return from((User) userObject);
if (userObject instanceof ApplicationUser) return from((ApplicationUser) userObject);
return null;
}
}