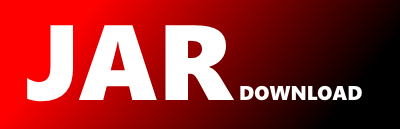
com.almworks.jira.structure.api2g.DataVersion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api2g;
public class DataVersion {
public static final DataVersion ZERO = new DataVersion(0, 0);
private final int mySignature;
private final int myVersion;
public DataVersion(int signature, int version) {
mySignature = signature;
myVersion = version;
}
public boolean isComparable(DataVersion anotherVersion) {
return mySignature == anotherVersion.getSignature();
}
public boolean isBefore(DataVersion anotherVersion) {
if (!isComparable(anotherVersion)) {
assert false : this + " vs. " + anotherVersion;
return false;
}
return myVersion < anotherVersion.getVersion();
}
public DataVersion increment() {
return increment(1);
}
public DataVersion increment(int count) {
if (count < 0) {
throw new IllegalArgumentException("version cannot decrement");
}
return new DataVersion(mySignature, myVersion + count);
}
/**
* Signature defines the identity of the version sequence. Only versions within the same signature can be compared.
*
* @return the signature of the version sequence
*/
public int getSignature() {
return mySignature;
}
/**
* @return the version
*/
public int getVersion() {
return myVersion;
}
public String toString() {
return String.format("%08x:%d", mySignature, myVersion);
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DataVersion that = (DataVersion) o;
if (mySignature != that.mySignature) return false;
if (myVersion != that.myVersion) return false;
return true;
}
public int hashCode() {
int result = mySignature;
result = 31 * result + myVersion;
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy