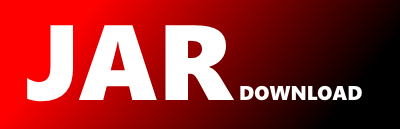
com.almworks.jira.structure.api2g.attribute.AttributeSpecUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api2g.attribute;
import com.almworks.jira.structure.api2g.attribute.loader.AttributeLoader;
import com.almworks.jira.structure.util.StructureUtil;
import com.google.common.collect.ImmutableMap;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.text.ParseException;
import java.util.HashMap;
import java.util.Map;
public class AttributeSpecUtil {
public static final String DEFAULT_ATTRIBUTESPEC_PARAMETER_NAME = "attribute";
public static final String DEFAULT_FORMAT_PARAMETER_NAME = "format";
public static final String DEFAULT_ID_PARAMETER_NAME = "id";
public static final String DEFAULT_PARAMS_PARAMETER_NAME = "params";
private static Map buildSimpleAttributeParameterMap(String value) {
if (value == null) {
return null;
}
if (value.startsWith("{") && value.endsWith("}")) {
throw new IllegalArgumentException("simple parameter must not be a text in curly braces, like " + value);
}
Map map = new HashMap<>(1);
map.put(DEFAULT_ATTRIBUTESPEC_PARAMETER_NAME, ImmutableMap.builder().put("id", value).build());
return map;
}
@Nullable
public static AttributeLoader cast(AttributeLoader> loader, AttributeSpec spec) {
if (loader == null) return null;
if (!loader.getAttributeSpec().equals(spec)) return null;
//noinspection unchecked
return (AttributeLoader) loader;
}
@NotNull
public static AttributeSpec> parseAttributeSpecWithStandardFormat(String specString) throws ParseException {
// todo configure static ObjectMapper and use it
int p = getFormatIndex(specString);
ValueFormat> format = ValueFormat.getStandardFormat(specString.substring(p + 1));
if (format == null) {
throw new ParseException("cannot parse attribute specification [" + specString + "]: unknown value format", p + 1);
}
return parseIdAndParams(specString, p, format);
}
@NotNull
public static AttributeSpec parseAttributeSpec(String specString, ValueFormat expectedFormat) throws ParseException {
int p = getFormatIndex(specString);
if (!expectedFormat.getFormatId().equals(specString.substring(p + 1))) {
throw new ParseException("error parsing attribute specification [" + specString + "]: expected format " + expectedFormat + ", received format " + specString.substring(p + 1), p + 1);
}
return parseIdAndParams(specString, p, expectedFormat);
}
private static int getFormatIndex(String specString) throws ParseException {
int p = specString.lastIndexOf(':');
if (p < 0) {
throw new ParseException("error parsing attribute specification - colon not found: [" + specString + "]", 0);
}
return p;
}
private static AttributeSpec parseIdAndParams(String specString, int formatIndex, ValueFormat format) throws ParseException {
int k = specString.indexOf(':');
assert k >= 0 : k;
String id = specString.substring(0, k);
Map params = null;
if (k != formatIndex) {
String paramString = specString.substring(k + 1, formatIndex);
if (paramString.startsWith("{") && paramString.endsWith("}")) {
params = StructureUtil.fromJson(paramString, Map.class);
} else {
params = buildSimpleAttributeParameterMap(paramString);
}
}
try {
return new AttributeSpec(id, format, params);
} catch (IllegalArgumentException e) {
throw new ParseException("cannot parse attribute spec [" + specString + "]: " + e.getMessage(), 0);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy