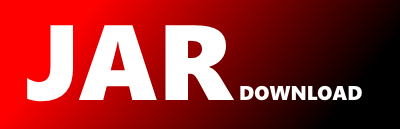
com.almworks.jira.structure.api.pull.DataVersion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api.pull;
import com.almworks.jira.structure.api.forest.ForestSource;
import com.atlassian.annotations.PublicApi;
import org.jetbrains.annotations.Nullable;
import javax.annotation.concurrent.Immutable;
/**
* {@code DataVersion} is used to identify whether some content has changed and to
* support incremental updates. It contains two numbers:
*
*
* - signature, which is a randomly chosen value that defines the "namespace"
* for the second number, and
* - version, which is a number that is incremented every time an update to
* the data happens.
*
*
* The code that observes {@link VersionedDataSource} would typically store last received
* {@code DataVersion} and use it next time in {@link VersionedDataSource#getUpdate(DataVersion)}.
*
* The code that implements {@code VersionedDataSource}, would typically increment {@code version}
* when an incremental update happens and reinitialize {@code signature} when non-incremental update
* happens.
*
* Two instances of {@code DataVersion} can be compared — we can tell which one happened
* before which, but only if their signatures match.
*
* @see VersionedDataSource
* @see ForestSource
*/
@PublicApi
@Immutable
public class DataVersion {
public static final DataVersion ZERO = new DataVersion(0, 0);
private final int mySignature;
private final int myVersion;
/**
* Constructs {@code DataVersion}.
*/
public DataVersion(int signature, int version) {
mySignature = signature;
myVersion = version;
}
/**
* Returns {@code true} if this version can be compared to the specified version (that is possible only if
* their signatures match)
*/
public boolean isComparable(@Nullable DataVersion anotherVersion) {
return anotherVersion != null && mySignature == anotherVersion.getSignature();
}
/**
* Returns {@code true} if this version is "before" the given version, that is, it has the same signature
* and lower version.
*/
public boolean isBefore(@Nullable DataVersion anotherVersion) {
if (!isComparable(anotherVersion)) {
assert false : this + " vs. " + anotherVersion;
return false;
}
assert anotherVersion != null : this;
return myVersion < anotherVersion.getVersion();
}
/**
* Creates a new {@code DataVersion} with version incremented by 1.
*/
public DataVersion increment() {
return increment(1);
}
/**
* Creates a new {@code DataVersion} with version incremented by the specified amount.
*/
public DataVersion increment(int count) {
if (count < 0) {
throw new IllegalArgumentException("version cannot decrement");
}
if (count == 0) return this;
return new DataVersion(mySignature, myVersion + count);
}
/**
* Signature defines the identity of the version sequence. Only versions within the same signature can be compared.
*
* @return the signature of the version sequence
*/
public int getSignature() {
return mySignature;
}
/**
* Returns the version within one sequence, identified by signature
*/
public int getVersion() {
return myVersion;
}
public String toString() {
return String.format("%08x:%d", mySignature, myVersion);
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DataVersion that = (DataVersion) o;
if (mySignature != that.mySignature) return false;
if (myVersion != that.myVersion) return false;
return true;
}
public int hashCode() {
int result = mySignature;
result = 31 * result + myVersion;
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy