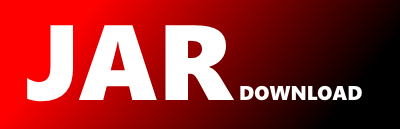
com.almworks.jira.structure.api.util.JsonUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api.util;
import com.atlassian.annotations.Internal;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.map.annotate.JsonSerialize;
import org.codehaus.jackson.type.TypeReference;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.*;
import static org.codehaus.jackson.map.DeserializationConfig.Feature.FAIL_ON_UNKNOWN_PROPERTIES;
@Internal
public final class JsonUtil {
private static final org.slf4j.Logger logger = LoggerFactory.getLogger(JsonUtil.class);
public static final TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy