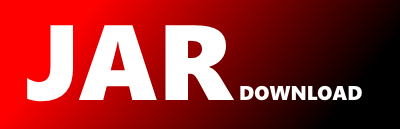
com.almworks.jira.structure.api.util.JiraUsers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api.util;
import com.atlassian.crowd.embedded.api.User;
import com.atlassian.jira.user.ApplicationUser;
import com.atlassian.jira.user.ApplicationUsers;
import org.jetbrains.annotations.Contract;
import org.jetbrains.annotations.Nullable;
/**
* Workaround for https://jira.atlassian.com/browse/JRA-62034
*/
public class JiraUsers {
private static final ClassLoader CLASS_LOADER = ApplicationUsers.class.getClassLoader();
@Contract("null -> null")
public static ApplicationUser byKey(@Nullable String userKey) {
if (userKey == null) {
return null;
}
Thread thread = Thread.currentThread();
ClassLoader ccl = thread.getContextClassLoader();
thread.setContextClassLoader(CLASS_LOADER);
try {
return ApplicationUsers.byKey(userKey);
} finally {
thread.setContextClassLoader(ccl);
}
}
@Contract("null -> null")
public static ApplicationUser from(@Nullable User user) {
if (user == null) {
return null;
}
Thread thread = Thread.currentThread();
ClassLoader ccl = thread.getContextClassLoader();
thread.setContextClassLoader(CLASS_LOADER);
try {
return ApplicationUsers.from(user);
} finally {
thread.setContextClassLoader(ccl);
}
}
@Contract("null -> null")
public static String getKeyFor(@Nullable User user) {
if (user == null) {
return null;
}
Thread thread = Thread.currentThread();
ClassLoader ccl = thread.getContextClassLoader();
thread.setContextClassLoader(CLASS_LOADER);
try {
return ApplicationUsers.getKeyFor(user);
} finally {
thread.setContextClassLoader(ccl);
}
}
@Contract("null -> null")
public static String getKeyFor(@Nullable ApplicationUser user) {
return ApplicationUsers.getKeyFor(user); // doesn't call ComponentAccessor
}
@Contract("null -> null")
public static User toDirectoryUser(@Nullable ApplicationUser user) {
return ApplicationUsers.toDirectoryUser(user); // doesn't call ComponentAccessor
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy