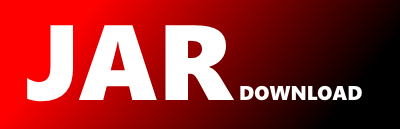
com.almworks.jira.structure.api.row.TransientRow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api.row;
import com.almworks.jira.structure.api.item.ItemIdentity;
import com.atlassian.annotations.Internal;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.function.Function;
@Internal
public class TransientRow implements StructureRow {
private final StructureRow myDelegate;
private final long myCreatorId;
private final long myOriginalId;
public TransientRow(@NotNull StructureRow delegate, long creatorId, long originalId) {
myDelegate = delegate;
myCreatorId = creatorId;
myOriginalId = originalId;
}
@Override
public long getRowId() {
return myDelegate.getRowId();
}
@Override
@NotNull
public ItemIdentity getItemId() {
return myDelegate.getItemId();
}
@Override
public long getSemantics() {
return myDelegate.getSemantics();
}
@Override
@Nullable
public I getItem(@NotNull Class itemClass) {
return myDelegate.getItem(itemClass);
}
public long getCreatorId() {
return myCreatorId;
}
public long getOriginalId() {
return myOriginalId;
}
@Override
public String toString() {
return "TransientRow[" + getRowId() + ":" + getItemId() + ":" + getSemantics() + ":" + myCreatorId + ":" + myOriginalId + "]";
}
public static long getCreatorId(StructureRow row) {
return row instanceof TransientRow ? ((TransientRow) row).getCreatorId() : 0;
}
public static long getOriginalId(StructureRow row) {
return row instanceof TransientRow ? ((TransientRow) row).getOriginalId() : 0;
}
public static StructureRow getDelegate(StructureRow row) {
while (row instanceof TransientRow) {
row = ((TransientRow) row).myDelegate;
}
return row;
}
/**
* Replaces delegate in the transient row chain. If replacer function returns the same row or null, no replacement takes place.
*/
public static StructureRow replaceDelegate(StructureRow row, Function replacer) {
if (row instanceof TransientRow) {
TransientRow tr = (TransientRow) row;
StructureRow delegate = getDelegate(row);
StructureRow replaced = replacer.apply(delegate);
return delegate == replaced || replaced == null ? row : new TransientRow(replaced, tr.getCreatorId(), tr.getOriginalId());
} else {
StructureRow replaced = replacer.apply(row);
return replaced == null ? row : replaced;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy