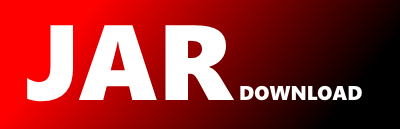
com.almworks.jira.structure.api.sync.SyncAuditLog Maven / Gradle / Ivy
Show all versions of structure-api Show documentation
package com.almworks.jira.structure.api.sync;
import com.almworks.jira.structure.api.util.MapObject;
import com.atlassian.annotations.PublicApi;
import org.apache.commons.lang.StringUtils;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.Collections;
import java.util.List;
/**
* This interface is used by synchronizers to log audit information regarding their actions, configuration changes, etc.
* Synchronizers can optionally implement support for undoing action records from the audit log.
*
* This component replaces Structure 2.x component SynchronizerUndoRecorder
*
* @author Igor Sereda
* @author Igor Baltiyskiy
*/
@PublicApi
public interface SyncAuditLog {
/**
*
* Adds records to the audit log for a logical group of actions taken by one synchronizer in the course of one run.
* Can also be used to record meta-actions that affect multiple synchronizer instances.
*
*
* The format of each action string is up to the synchronizer. If the synchronizer supports undo for the action,
* there should be enough information for it to perform the undo.
*
*
* All records are represented as {@link MapObject}s. They are stored in JSON format as generated by {@link MapObject#toString}.
*
* @param description optional: description of the change
* @param actions a list of actions in the arbitrary format. If an action is {@code null} or an empty String, it is skipped.
* If the list is {@code null} or empty, no action records are created - only description record if description is provided
*/
void recordActions(@NotNull SyncInstance syncInstance, @Nullable MapObject description, @Nullable List actions);
/**
*
* Like {@link #recordActions(SyncInstance, MapObject, List)}, but doesn't require a SyncInstance
.
*
*
* @param syncInstanceId ID of the sync instance or 0 if the actions refer to multiple synchronizers
* @param syncModuleKey module key of the synchronizer that performed the actions or an empty string if the actions refer
* to multiple synchronizer instances
* @param structureId ID of the structure, with which the synchronizer instance (or instances) is associated. 0 if
* the actions refer to synchronizer instances associated with multiple structures
* @see #recordActions(SyncInstance, MapObject, List)
* */
void recordActions(long syncInstanceId, @NotNull String syncModuleKey, long structureId,
@Nullable MapObject description, @Nullable List actions);
/**
* Checks if the synchronizer audit log that contains the specified actions is going to be actually recorded.
* This method can be used to check if there's a need to call {@link #recordActions(SyncInstance, MapObject, List)}
* in case preparation of description is a resource-heavy operation.
*
* @param actions a list of actions to be later passed to {@code recordActions}
* @return true if {@code recordActions} will do any meaningful work
*/
boolean isActionGroupRecorded(@Nullable List actions);
/**
*
* Retrieve recorded actions. You can filter the actions to get actions for a particular sync instance,
* for a period of time, or both.
*
*
* The actions are grouped together so that each group corresponds to a single {@link #recordActions} call.
* Action groups come in the order of decreasing timestamp
*
*
* Note: the number of actions in the returned action groups is subject to the hard limit. If there are too many
* actions, the actions in the last action group might be truncated.
*
* @param syncInstanceId 0 or sync instance ID to filter by sync instance
* @param structureId 0 or structure ID to filter by sync instances installed on this structure
* @param timestampFrom minimum timestamp (ms from the Epoch), inclusive; {@link Long#MIN_VALUE} yields all actions from the beginning
* @param timestampTo maximum timestamp (ms from the Epoch), exclusive; {@link Long#MAX_VALUE} yields all actions until the end */
List getActions(long syncInstanceId, long structureId, long timestampFrom, long timestampTo);
class ActionGroup {
private final long mySyncInstanceId;
private final String mySyncModuleKey;
private final long myStructureId;
private final long myTimestamp;
private final MapObject myDescription;
private final List myActions;
public ActionGroup(long syncInstanceId, String syncModuleKey, long structureId, long timestamp,
MapObject description,
List actions)
{
mySyncInstanceId = syncInstanceId;
myStructureId = structureId;
mySyncModuleKey = StringUtils.isEmpty(syncModuleKey) ? null : syncModuleKey;
myTimestamp = timestamp;
myDescription = description;
myActions = Collections.unmodifiableList(actions);
}
public long getSyncInstanceId() {
return mySyncInstanceId;
}
@NotNull
public String getSyncModuleKey() {
return mySyncModuleKey;
}
public long getStructureId() {
return myStructureId;
}
public long getTimestamp() {
return myTimestamp;
}
@Nullable
public MapObject getDescription() {
return myDescription;
}
@NotNull
public List getActions() {
return myActions;
}
}
}