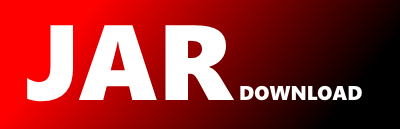
com.almworks.jira.structure.api.attribute.AttributeValuesReceiver Maven / Gradle / Ivy
Show all versions of structure-api Show documentation
package com.almworks.jira.structure.api.attribute;
import com.almworks.integers.LongSet;
import com.almworks.jira.structure.api.forest.ForestService;
import com.atlassian.annotations.PublicSpi;
import org.jetbrains.annotations.NotNull;
/**
* Implementing {@code AttributeValuesReceiver} and using {@link StructureAttributeService#loadAttributeValues} methods
* allows for the calling code to get some values while other values are still being loaded, get metadata and cancel the loading process.
*
* Most methods have default implementations so the only method that is required is {@link #receiveValues}. Override other methods
* if you need the corresponding information.
*
* For most methods, there's no particular order in which they are called. Each method may be called multiple times.
*
* The implementations of these method must be reasonably quick. Copying the data for the future consumption (maybe from another thread) is
* the typical design. You can take locks but make sure they are usually quick to acquire.
*
* You must not call {@link StructureAttributeService} or {@link ForestService} or any other system component that can potentially
* block or involve a lot of computation or cause reentrancy into the attribute service.
*
* @see StructureAttributeService#loadAttributeValues
*/
@PublicSpi
public interface AttributeValuesReceiver {
/**
* Offers the receiver to retrieve the values for the specified attribute. It is usually called when a chunk of the values
* for some attribute has been calculated.
*
* The receiver should request values from {@link ValueColumn} only for the rows that were requested
* in the {@link StructureAttributeService#loadAttributeValues} call. If you request a value for a row that was not originally requested,
* you can get either a {@code null}, a current value, or an outdated value.
*
* The implementation must not save the {@code values} instance anywhere. All values must be read inside this method.
*
* This method may be called multiple times for the same attribute spec. The later calls may contain the same or different values.
*
* Although {@link ValueColumn#getValue} may return null, it should not return null for the rows that were requested.
*
* @param attributeSpec attribute for which values have been loaded
* @param values values accessor
* @see ValueColumn
*/
void receiveValues(@NotNull AttributeSpec attributeSpec, @NotNull ValueColumn values);
/**
* Used to check if the attribute loading should be cancelled. This method is called periodically during the loading process.
*
* The implementation of this method should be really, really fast and not involve any locking. {@code isCancelled()} is called many times
* and any delay would be greatly multiplied.
*
* @return true if the loading should be cancelled
*/
default boolean isCancelled() {
return false;
}
/**
* Notifies the receiver that the loading process has loaded all requested rows for the given attribute spec. This may be
* used to count the progress or see which attributes are still being loaded.
*
* Note that {@link #receiveValues} for the same attribute may still be called after this method is called, if more values are loaded
* for this attribute and some rows that were not requested (this may be caused by other multi-row attributes depending on this one).
*
* @param attributeSpec attribute for which the requested values have been loaded
* @see #receiveValues
*/
default void valuesReady(@NotNull AttributeSpec> attributeSpec) {}
/**
* Provides the receiver with the metadata about the loading process. This method is called once right before the loading is completed.
*
* The metadata contains some versioning information and diagnostics.
*
* The versions - {@link ValuesMeta#getItemsVersion()} and {@link ValuesMeta#getForestVersion()} - are guaranteed to happen before
* or be the same as the actual versions of items stream and the forest at the moments when each reported value was loaded. You can use these
* versions to safely check if there might have been any updates in the items or the forest since the loading.
*
* @param meta metadata
*/
default void receiveMeta(@NotNull ValuesMeta meta) {}
/**
* Notifies the receiver that some of the requested rows were not available for loading by the requested user.
*
* A row may be unavailable because it doesn't exist or because the user does not the permission to view the associated item.
*
* This method may be called several times during a single loading call, and the subsequent calls may include the row IDs that were reported
* in the previous calls. An implementation should accumulate the row IDs somewhere.
*
* @param rows inaccessible row ids
*/
default void receiveInaccessibleRows(@NotNull LongSet rows) {}
}