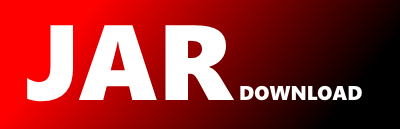
com.almworks.jira.structure.api.sync.SyncEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api.sync;
import com.almworks.integers.LongList;
import com.almworks.jira.structure.api.event.JiraChangeEvent;
import com.almworks.jira.structure.api.row.RowManager;
import com.almworks.jira.structure.api.structure.history.HistoryEntry;
import com.atlassian.annotations.PublicApi;
import org.jetbrains.annotations.Nullable;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
/**
* {@code SyncEvent} with its only subclasses, {@link SyncEvent.Structure} and {@link SyncEvent.Jira}, is
* used to represent to incremental synchronization a single change in JIRA or in Structure.
*
* Note that although both subclasses override the default {@code toString()} method to provide details about the event,
* {@link SyncEvent.Structure} includes into the output row IDs without resolving them into item IDs;
* row IDs might be of little use in application logs. You might want to create a more detailed message including row IDs
* by using {@link RowManager}.
*
* @author Igor Sereda
*/
@PublicApi
public abstract class SyncEvent implements Comparable {
static final ThreadLocal TIMESTAMP_FORMAT = new ThreadLocal() {
@Override
protected DateFormat initialValue() {
SimpleDateFormat fmt = new SimpleDateFormat("HH:mm:ss.SSS'Z'");
fmt.setTimeZone(TimeZone.getTimeZone("GMT"));
return fmt;
}
};
private final long myTimestamp;
private final Long mySyncInstanceId;
// todo Add user? It is currently only used in the logs, and apparently, we've never used this information.
// todo | The downside to adding the user is the need to add a column to the DB table.
private SyncEvent(long timestamp, @Nullable Long syncInstanceId) {
myTimestamp = timestamp;
mySyncInstanceId = syncInstanceId;
}
public long getTimestamp() {
return myTimestamp;
}
@Nullable
public Long getSyncInstanceId() {
return mySyncInstanceId;
}
@Override
public int compareTo(SyncEvent o) {
return Long.compare(myTimestamp, o.myTimestamp);
}
protected StringBuilder toString(StringBuilder builder) {
if (mySyncInstanceId != null) builder.append(':').append(mySyncInstanceId);
builder.append(TIMESTAMP_FORMAT.get().format(new Date(myTimestamp)));
return builder;
}
@PublicApi
public static final class Structure extends SyncEvent {
private final HistoryEntry myHistoryEntry;
public Structure(HistoryEntry historyEntry) {
super(historyEntry.getTimestamp(), historyEntry.getSynchronizer());
myHistoryEntry = historyEntry;
}
public HistoryEntry getHistoryEntry() {
return myHistoryEntry;
}
public String toString() {
return toString(new StringBuilder("s(")).append(',').append(myHistoryEntry).append(')').toString();
}
}
@PublicApi
public static final class Jira extends SyncEvent {
private final JiraChangeEvent myEvent;
public Jira(long timestamp, Long syncInstanceId, JiraChangeEvent event) {
super(timestamp, syncInstanceId);
if (event == null) throw new NullPointerException();
myEvent = event;
}
public JiraChangeEvent getEvent() {
return myEvent;
}
public LongList getAffectedIssuesSorted() {
return myEvent.getAffectedIssuesSorted();
}
public String toString() {
return toString(new StringBuilder("j(")).append(',').append(myEvent).append(')').toString();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy