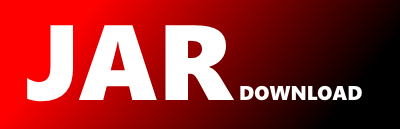
com.almworks.jira.structure.api.settings.StructurePage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structure-api Show documentation
Show all versions of structure-api Show documentation
Public API for the Structure Plugin for JIRA
package com.almworks.jira.structure.api.settings;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import org.jetbrains.annotations.Nullable;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import javax.xml.bind.annotation.XmlType;
import java.util.Collection;
import java.util.EnumSet;
/**
* StructurePage
enum lists all non-admin JIRA pages (page types) that display
* Structure Widget or that are adjusted by Structure Plugin. It is used to define per-page
* user interface settings.
*
* @see UISettings
* @author Igor Sereda
*/
@XmlType(name = "structure-page")
@XmlEnum
public enum StructurePage {
/**
* Structure Board page - the one that opens when you click Structure in the top menu bar.
*/
@XmlEnumValue("structure")
STRUCTURE_BOARD("structure"),
/**
* Either Structure Board page with issue details panel or Project page with issue details panel.
*
* @deprecated No longer supported since Structure doesn't use a separate view for a details panel layout.
* Use {@link #STRUCTURE_BOARD} or {@link #PROJECT_TAB} instead.
*/
@Deprecated
@XmlEnumValue("structure-with-details")
STRUCTURE_BOARD_WITH_DETAILS("structure-with-details"),
/**
* The page with a single issue.
*/
@XmlEnumValue("issue")
ISSUE_VIEW("issue"),
/**
* "Structure" tab on the project page.
*/
@XmlEnumValue("project")
PROJECT_TAB("project"),
/**
* "Structure" tab on the component page.
*/
@XmlEnumValue("component")
COMPONENT_TAB("component"),
/**
* "Structure" tab on the version page.
*/
@XmlEnumValue("version")
VERSION_TAB("version"),
/**
* Issue navigator - for now, Structure is present only as a Views menu element.
*/
@XmlEnumValue("navigator")
ISSUE_NAVIGATOR("navigator"),
/**
* Structure Gadget - either on Dashboard or in Confluence.
*/
@XmlEnumValue("gadget")
GADGET("gadget"),
/**
* "Structure" Tab in the issue details view on GreenHopper rapid board
*/
@XmlEnumValue("greenhopper")
GREENHOPPER_TAB("greenhopper");
private final String myId;
private StructurePage(String id) {
myId = id;
}
@JsonValue
public String getId() {
return myId;
}
@JsonCreator
@Nullable
public static StructurePage forId(@Nullable String id) {
if (id == null) return null;
for (StructurePage page : StructurePage.values()) {
if (id.equals(page.getId())) return page;
}
return null;
}
@Nullable
public static EnumSet enumSet(@Nullable Collection pages) {
if (pages == null || pages.isEmpty()) return null;
EnumSet set = EnumSet.noneOf(StructurePage.class);
set.addAll(pages);
return set;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy