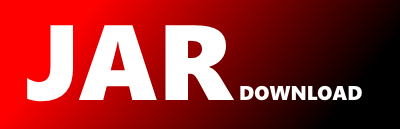
com.almworks.jira.structure.api.view.StructureView Maven / Gradle / Ivy
Show all versions of structure-api Show documentation
package com.almworks.jira.structure.api.view;
import com.almworks.jira.structure.api.auth.StructureAuth;
import com.almworks.jira.structure.api.error.StructureException;
import com.almworks.jira.structure.api.permissions.PermissionLevel;
import com.almworks.jira.structure.api.permissions.PermissionRule;
import com.almworks.jira.structure.api.settings.StructureConfiguration;
import com.almworks.jira.structure.api.structure.Structure;
import com.atlassian.annotations.PublicApi;
import com.atlassian.jira.user.ApplicationUser;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.Collection;
import java.util.List;
/**
* {@code StructureView} represents a view - a named collection of parameters (such as grid columns) that are used
* to configure how Structure widget displays a structure.
*
* The main property of a {@code StructureView} is view specification, which defines the visual configuration.
* Other properties - id, name, description, permissions and owner - help manage the views.
*
* You typically get an instance of {@code StructureView} by calling {@code get...} methods of {@link StructureViewManager}.
* Like {@link Structure}, an instance of {@code StructureView} is mutable — you can change its properties, but to
* have changes take effect you need to save them using {@link #saveChanges}.
*
*
* To create a new view, call {@link StructureViewManager#createView()} to get
* an empty {@code StructureView} instance, call {@code set...} methods to set the properties (at least, view name and view specification must be set)
* and then call {@link #saveChanges}.
* When {@code saveChanges} has completed, the new View has been created and you can use {@link #getId()} to get the
* new view's ID.
*
* Like structures, views also have an owner and a set of permission rules. The similar access level calculation
* algorithm applies for the views, except that {@link PermissionRule.ApplyStructure} rules are ignored.
*
* Access level applied to view has the following meaning:
*
*
* - {@link PermissionLevel#NONE} - the view is not accessible for the user.
* - {@link PermissionLevel#VIEW} - the user can apply this view when viewing any structure. The user can adjust the
* visual configuration in the browser by, say, adding columns, but they won't be able to save the changes as the new view specification.
* - {@link PermissionLevel#EDIT} - the user can use the view and also change its specification (save the adjustments they made in browser).
* The user won't be able to change other properties of the view, like name or permissions.
* - {@link PermissionLevel#ADMIN} - the user can make any change of the view or delete it.
*
*
* Instances of classes implementing this interface have reference equality: their {@code equals()}
* and {@code hashCode()} behave in the same way as {@code Object}'s.
*
* The implementations of this interface are not thread-safe. Every thread must obtain their
* own copy of the {@code StructureView} instance. Moreover, every time you need an
* instance of {@code StructureView}, you should get it from the {@code StructureViewManager}
* because the properties might have changed or the view might have been deleted.
*
* @see StructureViewManager
* @since 7.2.0 (Structure 2.0)
* @author Igor Sereda
*/
@PublicApi
public interface StructureView {
// GETTERS
/**
* Returns the ID of the view, or {@code 0} if the view has no ID (when it's new and hasn't been saved yet).
*
* @return the ID of the view, or 0 if it doesn't yet have an ID
*/
long getId();
/**
* Returns the name of the view or an empty string if the view name has not been set yet
*/
@NotNull
String getName();
/**
* Returns the description of the view or an empty string if description was not set
*/
@NotNull
String getDescription();
/**
* Returns the view specification, which defines the visual configuration of the widget
* @see ViewSpecification
*/
@NotNull
ViewSpecification getSpecification();
/**
* Returns the owner of the view, or {@code null} if the view has no owner. In the latter case, it is manageable
* by JIRA administrators, or whoever has {@link PermissionLevel#ADMIN} access to it.
*/
@Nullable
ApplicationUser getOwner();
/**
* Used to get permissions rules that are used to calculate user's access level. As with the permission rules
* for {@link Structure}, the last matching rule defines the access level.
*
* @return permission rules used to calculate the user's access level to this view
*/
@NotNull
List getPermissions();
// CONVENIENCE GETTERS
/**
* Calculates access level to this view for the specified user. If the user is not allowed to use Structure plugin,
* the result will always be {@link PermissionLevel#NONE}.
*
* This method does not take into account pending changes to the permissions list made through {@link #setPermissions}
* method call, until they are committed to the database with {@link #saveChanges}.
*
* This method does not take into account {@link StructureAuth authentication context}.
*
* @param user the user, null means anonymous
* @return the permissions level
* @see StructureViewManager#getViewPermission
*/
@NotNull
PermissionLevel getEffectivePermission(@Nullable ApplicationUser user);
/**
*
Checks if the view is "shared", that is, the permission rules give at least {@code VIEW} access to someone.
* If the view is not shared, only the owner and JIRA administrators have access to it.
*
* Note that a redundant rule, such as assigning the owner a permission level (although the owner always has
* {@code ADMIN} access) will make this view "shared", although technically it still will be "private".
*
* This method takes into account the pending changes to permissions made through {@link #setPermissions}.
*
* @return true if the view is shared
*/
boolean isShared();
/**
* Checks if the view is "public", that is, everyone has at least {@code VIEW} access to it.
*
*
This method takes into account the pending changes to permissions made through {@link #setPermissions}.
*
* @return true if the view is public
*/
boolean isPublic();
// MUTATORS
/**
*
Sets the name of the view. Although it is possible to set name to null, it is an invalid value
* and {@code saveChanges} will throw an error when called, unless a non-null name is set.
*
* To store the changed information in the database, use {@link #saveChanges}.
*
* @param name view name; {@code null} is interpreted as "don't change"
* @return this view
*/
@NotNull
StructureView setName(@Nullable String name);
/**
* Sets the description of the view.
*
* To store the changed information in the database, use {@link #saveChanges}.
*
* @param description view description; {@code null} is interpreted as "don't change"
* @return this view
*/
@NotNull
StructureView setDescription(@Nullable String description);
/**
* Sets the owner of the view.
*
* To store the changed information in the database, use {@link #saveChanges}.
*
* @param owner new owner. {@code null} is interpreted as "don't change".
* @return this view
*/
@NotNull
StructureView setOwner(@Nullable ApplicationUser owner);
/**
* Sets the permission rules for this view. Like with {@link Structure}, permission rules are evaluated in the specified order
* and the last matching rule defines the access level to the view for the given user.
*
* View owner and JIRA administrators always have {@link PermissionLevel#ADMIN} access level.
*
* Currently only {@link PermissionRule.SetLevel} rules are allowed for views.
*
* To store the changed information in the database, use {@link #saveChanges}. At this point, the specified rules are validated, and
* {@link StructureException} is thrown if any of them is found invalid.
*
* @param permissions a collection of permissions. {@code null} is interpreted as "don't change"
* @return this view
*/
@NotNull
StructureView setPermissions(@Nullable Collection extends PermissionRule> permissions);
/**
* Sets the specification of the view, which defines the visual configuration of the gadget.
*
* This method is intended for cases where you already have a ready specification that needs to be
* saved in a view. If you are building a specification, consider using {@link #setSpecification(ViewSpecification.Builder)}}.
*
* To store the changed information in the database, use {@link #saveChanges}.
*
* @param specification view specification. {@code null} is interpreted as "don't change"
* @return this view
*/
@NotNull
StructureView setSpecification(@Nullable ViewSpecification specification);
/**
* Sets the specification of the view, which defines the visual configuration of the gadget.
*
* This method is intended for cases where you build a new specification or adjust an existing specification via
* {@link ViewSpecification.Builder}. If you already have a ready instance of {@link ViewSpecification}, you can
* set it with {@link #setSpecification(ViewSpecification)}.
*
*
To store the changed information in the database, use {@link #saveChanges}.
*
* @param specification view specification. {@code null} is interpreted as "don't change"
* @return this view
*/
@NotNull
StructureView setSpecification(@Nullable ViewSpecification.Builder specification);
// CONVENIENCE MUTATORS
/**
* Updates the properties that have been set (have non-null value) in the passed builder. See {@link StructureViewBean}
* for details on the builder.
*
* To store the changed information in the database, use {@link #saveChanges}.
*
* @param builder the builder with view properties
* @return this view
*/
@NotNull
StructureView update(@Nullable StructureViewBean.Builder builder);
/**
* Convenience method that modifies the view's permission rules, making it viewable by anyone.
*
* If permission list contains rules that give higher than {@link PermissionLevel#VIEW}
permission to someone,
* those rules are retained. Other rules are removed.
*
* To store the changed information in the database, use {@link #saveChanges}.
*
* @return this view.
*/
@NotNull
StructureView makePublic();
/**
* Call this method to save the changes made with {@code set...} methods and update the database.
*
* Before this method is called, all changes are only stored in this instance of {@code StructureView} and have no other
* effect. After this method has successfully executed, the changes are stored in the database and applied.
*
* The updater - {@link StructureAuth#getUser() the current user} - must have
* {@link PermissionLevel#ADMIN} access level to the view and be allowed to use Structure plugin in
* {@link StructureConfiguration}.
* Anyone, except anonymous, can create new views.
* To share a view with someone else, the user needs to have "Create Shared Objects" global permission.
*
* All security checks can be {@link StructureAuth#isSecurityOverridden() disabled} for the current context.
*
* For detailed description of the permission rules validation, see {@link Structure#saveChanges}.
*
* @return this view
* @throws StructureException if there's a permissions problem, if required fields (name, specification) are not set, or if there
* are other problems
* @see Structure#saveChanges
*/
@NotNull
StructureView saveChanges() throws StructureException;
}