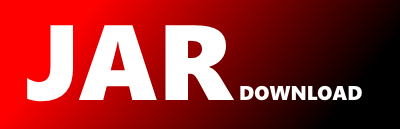
com.alogient.cameleon.sdk.community.blog.dao.BlogDao Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.blog.dao;
import com.alogient.cameleon.sdk.community.blog.dao.model.Blog;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogArticle;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogArticleComment;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogArticleCulture;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogTag;
import java.util.Collection;
/**
* This DAO is used to manage blog
*/
public interface BlogDao {
/**
* Used to tell to retrieve all results.
*/
public static final int ALL_RESULTS = -1;
/**
* Used to retreive a blog from the DB using it's PK
* @param blogId the blog PK
* @return the blog (if it exists)
*/
Blog getBlog(Integer blogId);
/**
* Used to retrieve the number of articles of a blog
* @param blogId id of the blog
* @return the number of articles
*/
int getNumberOfArticles(Integer blogId);
/**
* Used to retrieve a tag by id
* @param tagId id of the tag to retrieve
* @return the tag
*/
BlogTag getTagById(Integer tagId);
/**
* Used to retrieve the list of tags
* @return the list of tags
*/
Collection getTags();
/**
* Used to retrieve an article.
* @param articleId id of the article to retrieve
* @return an article
*/
BlogArticleCulture getArticleCulture(Integer articleId);
/**
* Used to retreive articles. The maxResult parameters will be used to specify
* how many articles you want to retrieve.
*
* @param blogId id of the blog
* @param firstResult tell to start at the firstResult id
* @param maxResult maximum articles to retrieve
* @param toDate return maxResult articles until the toDate is reached
* @return articles
*/
Collection getArticles(Integer blogId, Integer firstResult, Integer maxResult, Long toDate);
/**
* Save a blog article culture
* @param blogArticleCulture the blogarticleculture
*/
void saveBlogArticleCulture(BlogArticleCulture blogArticleCulture);
/**
* Saves or overwites an article
* @param blogArticle the article to save
*/
void saveArticle(BlogArticle blogArticle);
/**
* Delete an article
* @param articleId id of the article to delete
*/
void deleteArticle(Integer articleId);
/**
* Saves or overwites a comment
* @param blogArticleComment the comment to save
*/
void saveComment(BlogArticleComment blogArticleComment);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy