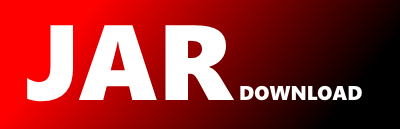
com.alogient.cameleon.sdk.community.blog.dao.model.Blog Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.blog.dao.model;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.OneToMany;
import javax.persistence.OrderBy;
import javax.persistence.Table;
import java.io.Serializable;
import java.util.Date;
import java.util.Set;
@Entity
@Table(name = "Blog")
public class Blog implements Serializable {
/**
* The blog's primary key
*/
private Integer id;
/**
* The blog creation date
*/
private Date creationDate;
/**
* Owner Id
*/
private String ownerId;
/**
* Owner firstname
*/
private String ownerFirstName;
/**
* Owner lastname
*/
private String ownerLastName;
/**
* The blog culture associated with this blog
*/
private Set blogCultures;
/**
* The article associated with this blog
*/
private Set blogArticles;
/**
* @return the blogId
*/
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "ID", nullable = false)
public Integer getId() {
return id;
}
/**
* @param id the blogId to set
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return the creationDate
*/
@Column(name = "CreationDate", nullable = false)
@Basic(optional=false)
public Date getCreationDate() {
return creationDate;
}
/**
* @param creationDate set the creationDate
*/
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
/**
* @return the ownerId
*/
@Column(name = "OwnerId", nullable = false)
@Basic(optional=false)
public String getOwnerId() {
return ownerId;
}
/**
* @param ownerId set the ownerId
*/
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
/**
* @return the ownerFirstName
*/
@Column(name = "OwnerFirstName")
public String getOwnerFirstName() {
return ownerFirstName;
}
/**
* @param ownerFirstName set the ownerFirstName
*/
public void setOwnerFirstName(String ownerFirstName) {
this.ownerFirstName = ownerFirstName;
}
/**
* @return the ownerLastName
*/
@Column(name = "OwnerLastName")
public String getOwnerLastName() {
return ownerLastName;
}
/**
* @param ownerLastName set the ownerLastName
*/
public void setOwnerLastName(String ownerLastName) {
this.ownerLastName = ownerLastName;
}
/**
* @return the blogCulture
*/
@OneToMany(fetch = FetchType.LAZY, mappedBy = "blog", cascade = CascadeType.ALL)
public Set getBlogCultures() {
return blogCultures;
}
/**
* @param blogCultures the blogCulture to set
*/
public void setBlogCultures(Set blogCultures) {
this.blogCultures = blogCultures;
}
/**
* @return the blogArticle
*/
@OneToMany(fetch = FetchType.LAZY, mappedBy = "blog", cascade = CascadeType.ALL)
@OrderBy("creationDate")
public Set getBlogArticles() {
return blogArticles;
}
/**
* @param blogArticles the blogArticle to set
*/
public void setBlogArticles(Set blogArticles) {
this.blogArticles = blogArticles;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy