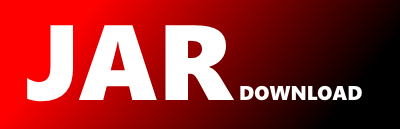
com.alogient.cameleon.sdk.community.blog.dao.model.BlogArticle Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.blog.dao.model;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import java.io.Serializable;
import java.util.Date;
import java.util.Set;
@Entity
@Table(name = "Article")
public class BlogArticle implements Serializable {
/**
* The blog article culture's primary key
*/
private Integer id;
/**
* The associated blog
*/
private Blog blog;
/**
* The blog article creation date
*/
private Date creationDate;
/**
* The article culture associated with this article
*/
private Set blogArticleCulture;
/**
* @return the blogId
*/
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "ID", nullable = false)
public Integer getId() {
return id;
}
/**
* @param id the blogCultureId to set
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return the blog
*/
@ManyToOne(fetch = FetchType.LAZY, optional = false, cascade = CascadeType.ALL)
@JoinColumn(name = "BlogId", referencedColumnName = "ID")
public Blog getBlog() {
return blog;
}
/**
* @param blog the blog to set
*/
public void setBlog(Blog blog) {
this.blog = blog;
}
/**
* @return the creationDate
*/
@Column(name = "CreationDate", nullable = false)
@Basic(optional=false)
public Date getCreationDate() {
return creationDate;
}
/**
* @param creationDate set the creationDate
*/
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
/**
* @return the blogArticleComment
*/
@OneToMany(fetch = FetchType.LAZY, mappedBy = "blogArticle", cascade = CascadeType.ALL)
public Set getBlogArticleCulture() {
return blogArticleCulture;
}
/**
* @param blogArticleCulture the blogArticleCulture to set
*/
public void setBlogArticleCulture(Set blogArticleCulture) {
this.blogArticleCulture = blogArticleCulture;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy