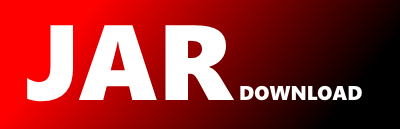
com.alogient.cameleon.sdk.community.blog.dao.model.BlogCulture Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.blog.dao.model;
import com.alogient.cameleon.sdk.common.dao.model.Culture;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import java.io.Serializable;
import java.util.Date;
@Entity
@Table(name = "BlogCulture")
public class BlogCulture implements Serializable {
/**
* The blog culture's primary key
*/
private Integer blogCultureId;
/**
* The associated blog
*/
private Blog blog;
/**
* The associated culture
*/
private Culture culture;
/**
* The blog last modification date
*/
private Date lastModified;
/**
* The blog's title
*/
private String title;
/**
* The blog's text
*/
private String text;
/**
* @return the blogId
*/
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "ID", nullable = false)
public Integer getBlogCultureId() {
return blogCultureId;
}
/**
* @param blogCultureId the blogCultureId to set
*/
public void setBlogCultureId(Integer blogCultureId) {
this.blogCultureId = blogCultureId;
}
/**
* @return the blog
*/
@ManyToOne(fetch = FetchType.LAZY, optional = false, cascade = CascadeType.ALL)
@JoinColumn(name = "BlogId", referencedColumnName = "ID")
public Blog getBlog() {
return blog;
}
/**
* @param blog the blog to set
*/
public void setBlog(Blog blog) {
this.blog = blog;
}
/**
* @return the culture
*/
@ManyToOne(fetch = FetchType.LAZY, optional = false, cascade = CascadeType.ALL)
@JoinColumn(name = "CultureCode", referencedColumnName = "CultureCode")
public Culture getCulture() {
return culture;
}
/**
* @param culture the culture to set
*/
public void setCulture(Culture culture) {
this.culture = culture;
}
/**
* @return the lastModified
*/
@Column(name = "LastModified", nullable = false)
@Basic(optional = false)
public Date getLastModified() {
return lastModified;
}
/**
* @param lastModified the lastModified to set
*/
public void setLastModified(Date lastModified) {
this.lastModified = lastModified;
}
/**
* @return the lastModified
*/
@Column(name = "title", nullable = false)
@Basic(optional = false)
public String getTitle() {
return title;
}
/**
* @param title the title to set
*/
public void setTitle(String title) {
this.title = title;
}
/**
* @return the lastModified
*/
@Column(name = "text", nullable = false)
@Basic(optional = false)
public String getText() {
return text;
}
/**
* @param text the text to set
*/
public void setText(String text) {
this.text = text;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy