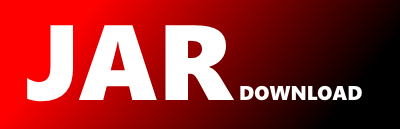
com.alogient.cameleon.sdk.community.blog.service.BlogService Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.blog.service;
import com.alogient.cameleon.sdk.common.util.exception.SaveObjectException;
import com.alogient.cameleon.sdk.community.blog.util.exception.ArticleNotFoundException;
import com.alogient.cameleon.sdk.community.blog.util.exception.BlogNotFoundException;
import com.alogient.cameleon.sdk.community.blog.vo.ArticleVo;
import com.alogient.cameleon.sdk.community.blog.vo.BlogVo;
import com.alogient.cameleon.sdk.community.blog.vo.CommentVo;
import com.alogient.cameleon.sdk.community.blog.vo.TagVo;
import com.alogient.cameleon.sdk.content.vo.CultureVo;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
/**
*
*/
@Transactional(propagation = Propagation.REQUIRES_NEW, readOnly = false)
public interface BlogService {
/**
* Get the blog from blog id
* @param blogId the id of the blog
* @param cultureVo the culture vo
* @return a blog
* @throws com.alogient.cameleon.sdk.community.blog.util.exception.BlogNotFoundException If the blog could not be found or converted
*/
BlogVo getBlog(Integer blogId, CultureVo cultureVo)
throws BlogNotFoundException;
/**
* Used to retrieve the number of articles of a blog
* @param blogId id of the blog
* @return the number of articles
*/
int getNumberOfArticles(Integer blogId);
/**
* Used to retrieve the list of tags
* @return the list of tags
*/
List getTags();
/**
* Get the article by id
* @param articleId id of the article
* @throws ArticleNotFoundException if the article could not be found or converted
* @return the article
*/
ArticleVo getArticle(Integer articleId)
throws ArticleNotFoundException;
/**
* Get the articles associated to a blog
*
* @param blogId the id of the blog
* @param cultureVo the culture vo
* @throws ArticleNotFoundException if the article could not be found or converted
* @return articles
*/
List getArticles(Integer blogId, CultureVo cultureVo)
throws ArticleNotFoundException;
/**
* Get the X latest articles associated to a blog
*
* @param blogId the id of the blog
* @param cultureVo the culture vo
* @param maxResult maximum articles to retrieve
* @param toDate return maxResult articles until the toDate is reached.
* @throws com.alogient.cameleon.sdk.community.blog.util.exception.ArticleNotFoundException if the article could not
* be found or converted
* @return articles
*/
List getArticles(Integer blogId, CultureVo cultureVo, Integer maxResult, Long toDate)
throws ArticleNotFoundException;
/**
* Get the articles associated to a blog
*
* @param blogId the id of the blog
* @param cultureVo the culture vo
* @param firstResult tell to start at the firstResult id
* @param maxResult maximum articles to retrieve
* @param toDate return maxResult articles until the toDate is reached
* @return articles
* @throws com.alogient.cameleon.sdk.community.blog.util.exception.ArticleNotFoundException if the article could not
* be found or converted
*/
List getArticles(Integer blogId, CultureVo cultureVo, Integer firstResult, Integer maxResult,
Long toDate)
throws ArticleNotFoundException;
/**
* Update an article
* @param articleVo the article to save
* @throws SaveObjectException thrown if the article could not be saved
*/
public void updateArticle(ArticleVo articleVo) throws SaveObjectException;
/**
* Submit an article
* @param blogId the id of the blog
* @param cultureVo the culture vo
* @param articleVo the article to save
* @throws SaveObjectException thrown if the article could not be saved
*/
public void submitArticle(Integer blogId, CultureVo cultureVo, ArticleVo articleVo) throws SaveObjectException;
/**
* Delete an article
* @param articleId id of the article
*/
public void deleteArticle(Integer articleId);
/**
* Submit a comment
* @param cultureVo the culture vo
* @param commentVo the comment to save
* @throws SaveObjectException thrown if the comment could not be saved
*/
public void submitComment(CultureVo cultureVo, CommentVo commentVo) throws SaveObjectException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy