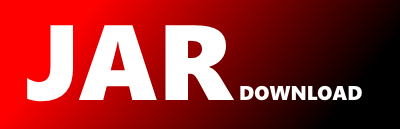
com.alogient.cameleon.sdk.community.blog.service.impl.BlogServiceImpl Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.blog.service.impl;
import com.alogient.cameleon.sdk.common.dao.model.Culture;
import com.alogient.cameleon.sdk.common.util.exception.SaveObjectException;
import com.alogient.cameleon.sdk.community.blog.dao.BlogDao;
import com.alogient.cameleon.sdk.community.blog.dao.model.Blog;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogArticle;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogArticleComment;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogArticleCulture;
import com.alogient.cameleon.sdk.community.blog.dao.model.BlogTag;
import com.alogient.cameleon.sdk.community.blog.service.BlogService;
import com.alogient.cameleon.sdk.community.blog.util.BlogModelToVoConverter;
import com.alogient.cameleon.sdk.community.blog.util.exception.ArticleNotFoundException;
import com.alogient.cameleon.sdk.community.blog.util.exception.BlogNotFoundException;
import com.alogient.cameleon.sdk.community.blog.vo.ArticleVo;
import com.alogient.cameleon.sdk.community.blog.vo.BlogVo;
import com.alogient.cameleon.sdk.community.blog.vo.CommentVo;
import com.alogient.cameleon.sdk.community.blog.vo.TagVo;
import com.alogient.cameleon.sdk.content.dao.CultureDao;
import com.alogient.cameleon.sdk.content.vo.CultureVo;
import java.sql.Date;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class BlogServiceImpl implements BlogService {
/**
* Blog data access object
*/
private BlogDao blogDao;
/**
* Culture data access object
*/
private CultureDao cultureDao;
/**
* @param blogDao the blogDao to set
*/
public void setBlogDao(BlogDao blogDao) {
this.blogDao = blogDao;
}
/**
* @param cultureDao the CultureDao to set
*/
public void setCultureDao(CultureDao cultureDao) {
this.cultureDao = cultureDao;
}
@Override
public BlogVo getBlog(Integer blogId, CultureVo cultureVo) throws BlogNotFoundException {
// Check for invalid parameters
if (blogId == null) {
throw new BlogNotFoundException(
"Not such blog in the database. Blog.ID: " + blogId);
} else if (cultureVo == null) {
throw new BlogNotFoundException(
"Not such blog in the database. Culture: " + cultureVo);
}
// Get the data blog the database
Blog blog = blogDao.getBlog(blogId);
if (blog == null) {
throw new BlogNotFoundException(
"Not such blog in the database. Blog.ID: " + blogId);
}
Culture culture = cultureDao.getCulture(cultureVo.getCultureId());
if (culture == null) {
throw new BlogNotFoundException(
"Not such blog in the database. Culture.CultureID: "
+ cultureVo.getCultureId());
}
// Get number of articles
int numberOfArticles = blogDao.getNumberOfArticles(blogId);
// Convert the blog into a BlogVo
return BlogModelToVoConverter.convertToBlogVo(blog, numberOfArticles, culture);
}
@Override
public int getNumberOfArticles(Integer blogId) {
return blogDao.getNumberOfArticles(blogId);
}
@Override
public List getTags() {
return BlogModelToVoConverter.convertToTagVo(blogDao.getTags());
}
@Override
public ArticleVo getArticle(Integer articleId) throws ArticleNotFoundException {
// Check for invalid parameters
if (articleId == null) {
throw new ArticleNotFoundException(
"Not such article in the database. article.id: " + articleId);
}
BlogArticleCulture blogArticleCulture = blogDao.getArticleCulture(articleId);
// Convert the list of article into a ArticleVo
return BlogModelToVoConverter.convertToArticleVo(blogArticleCulture);
}
@Override
public List getArticles(Integer blogId, CultureVo cultureVo)
throws ArticleNotFoundException {
return getArticles(blogId, cultureVo, 0, BlogDao.ALL_RESULTS, null);
}
@Override
public List getArticles(Integer blogId, CultureVo cultureVo, Integer maxResult, Long toDate) throws ArticleNotFoundException {
return getArticles(blogId, cultureVo, 0, maxResult, toDate);
}
@Override
public List getArticles(Integer blogId, CultureVo cultureVo, Integer firstResult, Integer maxResult,
Long toDate) throws ArticleNotFoundException {
// Check for invalid parameters
if (blogId == null) {
throw new ArticleNotFoundException(
"Not such blog in the database. Blog.ID: " + blogId);
} else if (cultureVo == null) {
throw new ArticleNotFoundException(
"Not such article in the database. Culture: " + cultureVo);
}
Collection articles = blogDao.getArticles(blogId, firstResult, maxResult, toDate);
Culture culture = cultureDao.getCulture(cultureVo.getCultureId());
if (culture == null) {
throw new ArticleNotFoundException(
"Not such articles in the database. Culture.CultureID: "
+ cultureVo.getCultureId());
}
// Convert the list of article into a ArticleVo
return BlogModelToVoConverter.convertToArticleVo(articles, culture);
}
@Override
public void updateArticle(ArticleVo articleVo) throws SaveObjectException {
if (articleVo == null) {
throw new NullPointerException("articleVo could not be null");
}
BlogArticleCulture blogArticleCulture = blogDao.getArticleCulture(articleVo.getId());
// update it
blogArticleCulture.setTitle(articleVo.getTitle());
blogArticleCulture.setText(articleVo.getText());
// Add the tags
Set blogTags = new HashSet();
List tags = articleVo.getTags();
for (TagVo tag : tags) {
BlogTag blogTag = blogDao.getTagById(tag.getId());
blogTags.add(blogTag);
}
blogArticleCulture.setBlogTags(blogTags);
blogDao.saveBlogArticleCulture(blogArticleCulture);
}
@Override
public void deleteArticle(Integer articleId) {
// Check for invalid parameters
if (articleId == null) {
throw new NullPointerException("Blog.ID could not be null");
}
blogDao.deleteArticle(articleId);
}
@Override
public void submitArticle(Integer blogId, CultureVo cultureVo, ArticleVo articleVo)
throws SaveObjectException {
// Check for invalid parameters
if (blogId == null) {
throw new NullPointerException("Blog.ID could not be null");
} else if (cultureVo == null) {
throw new NullPointerException("CultureVo could not be null");
} else if (articleVo == null) {
throw new NullPointerException("articleVo could not be null");
}
// Get the dependen objects from the data base
Culture culture = cultureDao.getCulture(cultureVo.getCultureId());
if (culture == null) {
throw new SaveObjectException("Unable to save an article. The culture is empty");
}
Date currentDate = new Date(System.currentTimeMillis());
HashSet blogArticleCultures = new HashSet();
BlogArticleCulture blogArticleCulture = new BlogArticleCulture();
BlogArticle blogArticle = new BlogArticle();
blogArticle.setCreationDate(currentDate);
blogArticle.setBlog(blogDao.getBlog(blogId));
blogArticleCultures.add(blogArticleCulture);
blogArticle.setBlogArticleCulture(blogArticleCultures);
blogArticleCulture.setTitle(articleVo.getTitle());
blogArticleCulture.setText(articleVo.getText());
blogArticleCulture.setAuthorId(articleVo.getAuthorId());
blogArticleCulture.setAuthorFirstName(articleVo.getAuthorFirstName());
blogArticleCulture.setAuthorLastName(articleVo.getAuthorLastName());
blogArticleCulture.setCulture(culture);
blogArticleCulture.setLastModified(currentDate);
blogArticleCulture.setBlogArticle(blogArticle);
// Add the tags
Set blogTags = new HashSet();
List tags = articleVo.getTags();
for (TagVo tag : tags) {
BlogTag blogTag = blogDao.getTagById(tag.getId());
blogTags.add(blogTag);
}
blogArticleCulture.setBlogTags(blogTags);
blogDao.saveArticle(blogArticle);
}
@Override
public void submitComment(CultureVo cultureVo, CommentVo commentVo)
throws SaveObjectException {
// Check for invalid parameters
if (cultureVo == null) {
throw new NullPointerException("CultureVo could not be null");
} else if (commentVo == null) {
throw new NullPointerException("CommentVo could not be null");
}
Date currentDate = new Date(System.currentTimeMillis());
BlogArticleCulture blogArticle = blogDao.getArticleCulture(commentVo.getArticleId());
BlogArticleComment comment = new BlogArticleComment();
comment.setBlogArticleCulture(blogArticle);
comment.setCreationDate(currentDate);
comment.setLastModified(currentDate);
comment.setText(commentVo.getText());
comment.setAuthorId(commentVo.getAuthorId());
comment.setAuthorFirstName(commentVo.getAuthorFirstName());
comment.setAuthorLastName(commentVo.getAuthorLastName());
blogDao.saveComment(comment);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy