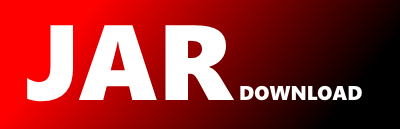
com.alogient.cameleon.sdk.community.blog.vo.BlogVo Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.blog.vo;
import java.io.Serializable;
import java.util.Date;
public class BlogVo implements Serializable {
/**
* The blog id
*/
private Integer id;
/**
* Number of articles
*/
private int numberOfArticles;
/**
* Owner id
*/
private String ownerId;
/**
* Owner first name
*/
private String ownerFirstName;
/**
* Owner last name
*/
private String ownerLastName;
/**
* Blog text
*/
private String text;
/**
* Blog title
*/
private String title;
/**
* Creation date
*/
private Date creationDate;
/**
* Last modified date
*/
private Date lastModified;
/**
* @return the blog id
*/
public Integer getId() {
return id;
}
/**
* @param blogId the blog id
*/
public void setId(Integer blogId) {
this.id = blogId;
}
/**
* @return the number of articles
*/
public int getNumberOfArticles() {
return numberOfArticles;
}
/**
* @param numberOfArticles the number of articles to set
*/
public void setNumberOfArticles(int numberOfArticles) {
this.numberOfArticles = numberOfArticles;
}
/**
* @return the owner id
*/
public String getOwnerId() {
return ownerId;
}
/**
* @param ownerId the ownerId to set
*/
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
/**
* @return the ownerFirstName
*/
public String getOwnerFirstName() {
return ownerFirstName;
}
/**
* @param ownerFirstName the ownerFirstName to set
*/
public void setOwnerFirstName(String ownerFirstName) {
this.ownerFirstName = ownerFirstName;
}
/**
* @return the ownerLastName
*/
public String getOwnerLastName() {
return ownerLastName;
}
/**
* @param ownerLastName the ownerLastName to set
*/
public void setOwnerLastName(String ownerLastName) {
this.ownerLastName = ownerLastName;
}
/**
* @return the text
*/
public String getText() {
return text;
}
/**
* @param text the text to set
*/
public void setText(String text) {
this.text = text;
}
/**
* @return the title
*/
public String getTitle() {
return title;
}
/**
* @param title the title to set
*/
public void setTitle(String title) {
this.title = title;
}
/**
* @return the creationDate
*/
public Date getCreationDate() {
return creationDate;
}
/**
* @param creationDate the creationDate to set
*/
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
/**
* @return the lastModified
*/
public Date getLastModified() {
return lastModified;
}
/**
* @param lastModified the lastModified to set
*/
public void setLastModified(Date lastModified) {
this.lastModified = lastModified;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy