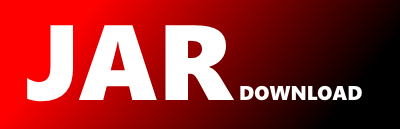
com.alogient.cameleon.sdk.community.blog.vo.CommentVo Maven / Gradle / Ivy
package com.alogient.cameleon.sdk.community.blog.vo;
import java.io.Serializable;
import java.util.Date;
public class CommentVo implements Serializable {
/**
* Id of the comment
*/
private Integer id;
/**
* Id of the article
*/
private Integer articleId;
/**
* The article creation date
*/
private Date creationDate;
/**
* The article last modification date
*/
private Date lastModified;
/**
* The article's text
*/
private String text;
/**
* The author id. Id in the membership module
*/
private String authorId;
/**
* The author first name
*/
private String authorFirstName;
/**
* The author last name
*/
private String authorLastName;
/**
* @return the blog id
*/
public Integer getId() {
return id;
}
/**
* @param blogId the blog id
*/
public void setId(Integer blogId) {
this.id = blogId;
}
/**
* @return the article id
*/
public Integer getArticleId() {
return articleId;
}
/**
* @param articleId the articleId to set
*/
public void setArticleId(Integer articleId) {
this.articleId = articleId;
}
/**
* @return the text
*/
public String getText() {
return text;
}
/**
* @param text the text to set
*/
public void setText(String text) {
this.text = text;
}
/**
* @return the creationDate
*/
public Date getCreationDate() {
return creationDate;
}
/**
* @param creationDate the creationDate to set
*/
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
/**
* @return the lastModified
*/
public Date getLastModified() {
return lastModified;
}
/**
* @param lastModified the lastModified to set
*/
public void setLastModified(Date lastModified) {
this.lastModified = lastModified;
}
/**
* @return the authorId
*/
public String getAuthorId() {
return authorId;
}
/**
* @param authorId the authorId to set
*/
public void setAuthorId(String authorId) {
this.authorId = authorId;
}
/**
* @return the authorFirstName
*/
public String getAuthorFirstName() {
return authorFirstName;
}
/**
* @param authorFirstName the authorFirstName to set
*/
public void setAuthorFirstName(String authorFirstName) {
this.authorFirstName = authorFirstName;
}
/**
* @return the authorLastName
*/
public String getAuthorLastName() {
return authorLastName;
}
/**
* @param authorLastName the authorLastName to set
*/
public void setAuthorLastName(String authorLastName) {
this.authorLastName = authorLastName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy