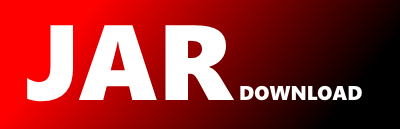
com.alogient.cameleon.sdk.community.interspire.request.impl.BaseRequest Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.community.interspire.request.impl;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.io.StringReader;
import java.io.StringWriter;
import java.net.HttpURLConnection;
import java.net.Proxy;
import java.net.URL;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import org.apache.commons.io.IOUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.alogient.cameleon.sdk.community.interspire.jaxb.CustomFields;
import com.alogient.cameleon.sdk.community.interspire.jaxb.Details;
import com.alogient.cameleon.sdk.community.interspire.jaxb.Item;
import com.alogient.cameleon.sdk.community.interspire.jaxb.XmlRequest;
import com.alogient.cameleon.sdk.community.interspire.request.ISend;
import com.alogient.cameleon.sdk.community.interspire.response.IResponse;
/**
* This is the base class for all requests. This class implements the ISend interface
*
* @author jmirc
*/
public class BaseRequest implements ISend {
private static final Logger LOGGER = LoggerFactory.getLogger(BaseRequest.class);
public static boolean isReady = false;
private static Marshaller marshaller;
private static Unmarshaller umarshaller;
private final String interspireURL;
/**
* Contructor. JABX needs a default contructor.
*
* @param interspireURL the Interspire URL
* @param responseClass the Class used by the unmarshaller object to deserialize the XML
*/
public BaseRequest(String interspireURL, Class responseClass) {
this.interspireURL = interspireURL;
try {
JAXBContext jc = JAXBContext.newInstance(XmlRequest.class, CustomFields.class, Details.class, Item.class, responseClass);
marshaller = jc.createMarshaller();
umarshaller = jc.createUnmarshaller();
isReady = true;
} catch (JAXBException e) {
LOGGER.error("Unable to initialize Jabx", e);
isReady = false;
}
}
/**
* Convert an object into an XML
*
* @param request the object to convert
* @return the XML
* @throws Exception if an exception occurs
*/
@Override
public String toXML(XmlRequest request) throws Exception {
if (!isReady) {
throw new Exception("JAXB has not been initializable");
}
StringWriter writer = new StringWriter();
try {
marshaller.marshal(request, writer);
return writer.getBuffer().toString();
} catch (Exception e) {
e.printStackTrace();
throw e;
}
}
/**
* Convert a xml response to a Response object
*
* @param xml the xml to convert
* @return the Response object
* @throws Exception if an exception occurs
*/
@Override
public IResponse fromXML(String xml) throws Exception {
if (!isReady) {
throw new Exception("JAXB has not been initializable");
}
StringReader in = new StringReader(xml);
return (IResponse) umarshaller.unmarshal(in);
}
/**
* Send the order to the PsiGate server
*
* @param request the request to send
* @return a response
* @throws Exception if any exception occurs
*/
@Override
public IResponse send(XmlRequest request) throws Exception {
return send(request, null);
}
/**
* Send the order to the PsiGate server
*
* @param request the request to send
* @param proxy used when a proxy must be used
* @return a response
* @throws Exception if any exception occurs
*/
@Override
public IResponse send(XmlRequest request, Proxy proxy) throws Exception {
if (!isReady) {
throw new Exception("JAXB has not been initializable");
}
URL url = new URL(interspireURL);
HttpURLConnection connection;
if (proxy != null) {
connection = (HttpURLConnection) url.openConnection(proxy);
} else {
connection = (HttpURLConnection) url.openConnection();
}
connection.setConnectTimeout(30000);
connection.setReadTimeout(30000);
connection.setDoInput(true);
connection.setDoOutput(true);
PrintWriter out = new PrintWriter(connection.getOutputStream());
String requestQuery = toXML(request);
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Request " + requestQuery);
}
out.println(requestQuery);
out.flush();
out.close();
// read the response
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String resp = IOUtils.toString(in);
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Response " + resp);
}
return fromXML(resp);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy