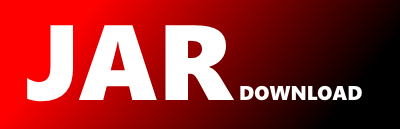
com.alogient.cameleon.sdk.content.dao.PageDao Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.dao;
import java.util.List;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import com.alogient.cameleon.sdk.content.dao.model.Page;
@Transactional(propagation = Propagation.REQUIRED, readOnly = true)
public interface PageDao {
/**
* Verify if a page exists
*
* @param cultureId The culture we want to test the existance for
* @param pageId The node we want to test the existance for
* @param isLive The environnement we want to test for
* @return True if the page exist, false otherwise
*/
boolean exist(Integer cultureId, Integer pageId, Boolean isLive);
/**
* Get page
*
* @param pageId The node we want the page from
* @return Page entity
*/
Page getPage(Integer pageId);
/**
* Get page
*
* @param cultureId The culture we want the page in
* @param navId The node we want the page from
* @return Page entity
*/
Page getPage(Integer cultureId, Integer navId);
/**
* List of visible supages of a page
*
* @param pageId The node we want the sub pages from
* @return Subpages collection
*/
List getSubPages(Integer pageId);
/**
* List of visible supages of a page
*
* @param cultureId The culture we want the sub pages in
* @param navId The node we want the sub pages from
* @return Subpages collection
*/
List getSubPages(Integer cultureId, Integer navId);
/**
* List of pages of a certain template
*
* @param templateName The template we want the pages for
* @param cultureId The culture we want the pages for
* @return Page collection
*/
List getTemplatePages(String templateName, Integer cultureId);
/**
* Creates a new page (nav)
*
* @param parentNavId The parent under which to create the page
* @param templateName The template the page corresponds to
* @param cultureCode The culture (default) to create the page in
* @param pageName The page name
* @param author The page author (creator)
* @return The created navId
*/
Integer createPage(Integer parentNavId, String templateName, String cultureCode, String pageName, String author);
/**
* Updates a page
*
* @param page the updated page
* @param author the author of the modification
*/
void pageUpdate(Page page, String author);
/**
* Approves a page
*
* @param navId the navId of the page to approve
* @param cultureCode the culture to approve it in
* @param author the approval author
*/
void pageApprove(Integer navId, String cultureCode, String author);
/**
* Changes the visibility of a page
*
* @param navId the navId to alter
* @param cultureCode the culture to alter
* @param isLive is this a change to live or preview status
* @param isVisible is this a change to visible or hidden
* @param author the change author
*/
void pageVisibilityUpdate(Integer navId, String cultureCode, boolean isLive, boolean isVisible, String author);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy