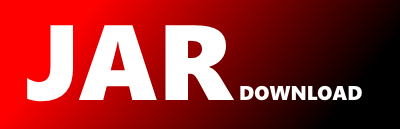
com.alogient.cameleon.sdk.content.dao.model.Element Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.dao.model;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import java.io.Serializable;
/**
* This entity represents the content of a part in a template for a certain page. This content can be either a text or a link to a binary
* content.
*
* @author alord
*/
@Entity
@Table(name = "Element")
public class Element implements Serializable {
/**
* Serial version unique identifier
*/
private static final long serialVersionUID = -1519475623664170668L;
/**
* FIXME Create field
*
* This entity's primary key
*/
private Integer elementId;
/**
* The page this element belongs to
*/
private Page page;
/**
* The part this element corresponds to in the template
*/
private Part part;
/**
* The "live" value (if any) of this element
*/
private String value;
/**
* The "live" link to binary content (if any) of this element
*/
private String file;
/**
* The "preview" value (if any) of this element
*/
private String previewValue;
/**
* The "preview" link to binary content (if any) of this element
*/
private String previewFile;
/**
* @return the elementId
*/
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "ElementID", nullable = false)
public Integer getElementId() {
return elementId;
}
/**
* @param elementId
* the elementId to set
*/
public void setElementId(Integer elementId) {
this.elementId = elementId;
}
/**
* @return the page
*/
@ManyToOne(fetch = FetchType.LAZY, cascade = CascadeType.ALL, optional = false)
@JoinColumn(name = "PageID")
public Page getPage() {
return page;
}
/**
* @param page
* the page to set
*/
public void setPage(Page page) {
this.page = page;
}
/**
* @return the part
*/
@ManyToOne(fetch = FetchType.LAZY, optional = false, cascade = CascadeType.ALL)
@JoinColumn(name = "PartID")
public Part getPart() {
return part;
}
/**
* @param part
* the part to set
*/
public void setPart(Part part) {
this.part = part;
}
/**
* @return the value
*/
@Column(name = "ElementValue", nullable = true)
@Basic(optional=true)
public String getValue() {
return value;
}
/**
* @param value
* the value to set
*/
public void setValue(String value) {
this.value = value;
}
/**
* @return the file
*/
@Column(name = "ElementFile", nullable = true)
@Basic(optional=true)
public String getFile() {
return file;
}
/**
* @param file
* the file to set
*/
public void setFile(String file) {
this.file = file;
}
/**
* @return the previewValue
*/
@Column(name = "ElementValuePreview", nullable = true)
@Basic(optional=true)
public String getPreviewValue() {
return previewValue;
}
/**
* @param previewValue
* the previewValue to set
*/
public void setPreviewValue(String previewValue) {
this.previewValue = previewValue;
}
/**
* @return the previewFile
*/
@Column(name = "ElementFilePreview", nullable = true)
@Basic(optional=true)
public String getPreviewFile() {
return previewFile;
}
/**
* @param previewFile
* the previewFile to set
*/
public void setPreviewFile(String previewFile) {
this.previewFile = previewFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy