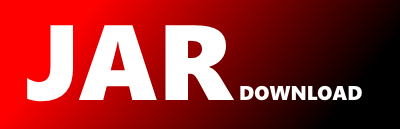
com.alogient.cameleon.sdk.content.dao.model.Page Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.dao.model;
import java.io.Serializable;
import java.util.Date;
import java.util.Set;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.NamedNativeQueries;
import javax.persistence.NamedNativeQuery;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import javax.persistence.Transient;
import com.alogient.cameleon.sdk.common.dao.model.Culture;
/**
* This entity represents a page or a document depending on how you use it. This is the object from where you get the
* data to display.
*
* @author alord
*/
@Entity
@Table(name = "Page")
@NamedNativeQueries({
@NamedNativeQuery(name = "getPageFromNav", query = "SELECT " + "p.* " + "FROM " + "Page AS p JOIN Culture AS c ON p.CultureCode = c.CultureCode " + "WHERE "
+ "p.NavID = :navId AND c.CultureId = :cultureId", resultClass = Page.class),
@NamedNativeQuery(name = "getSubPage", query = "SELECT " + "p.* " + "FROM " + "Page as parent " + "JOIN Nav AS n " + "ON parent.NavID = n.ParentID " + "JOIN Page AS p "
+ "ON n.NavID = p.NavID " + "AND parent.CultureCode = p.CultureCode " + "WHERE " + "parent.PageID = :parentPageId ORDER BY n.NavOrder", resultClass = Page.class),
@NamedNativeQuery(name = "getNavSubPage", query = "SELECT " + "p.* " + "FROM " + "Nav AS n " + "JOIN Page AS p " + "ON n.NavID = p.NavID " + "JOIN Culture AS c "
+ "ON p.CultureCode = c.CultureCode " + "WHERE " + "n.ParentID = :parentNavId AND c.CultureID = :cultureId ORDER BY n.NavOrder", resultClass = Page.class),
@NamedNativeQuery(name = "getTemplatePages", query = "SELECT " + "p.* " + "FROM " + "Page AS p " + "JOIN Nav AS n ON p.NavID = n.NavID "
+ "JOIN Template AS t ON n.TemplateID = t.TemplateID " + "JOIN Culture AS c ON p.CultureCode = c.CultureCode " + "WHERE "
+ "t.TemplateName = :templateName AND c.CultureId = :cultureId ORDER BY n.NavOrder", resultClass = Page.class) })
public class Page implements Serializable {
/**
* Serial version unique identifier
*/
private static final long serialVersionUID = -1852543561009710540L;
/**
* The primary for this item
*/
private Integer pageId;
/**
* The readable name for this item in "live" (it doesn't have to be unique)
*/
private String pageName;
/**
* The readable name for this item in "preview" (it doesn't have to be unique)
*/
private String pageNamePreview;
/**
* The associated navigation node
*/
private Nav nav;
/**
* The associated culture
*/
private Culture culture;
/**
* The status of the page
*/
private String pageStatus;
/**
* Is this page in the default menu / site map
*/
private Boolean pageNavEnd;
/**
* When was this page created
*/
private Date creationDate;
/**
* When was this page last update
*/
private Date lastModified;
/**
* The author of the last manipulation
*/
private String author;
/**
* Is this page visible in "live"
*/
private Boolean pageVisibility;
/**
* Is this page visible in "preview"
*/
private Boolean pageVisibilityPreview;
/**
* When was this page last approved
*/
private Date approbationDate;
/**
* The elements composing this page
*/
private Set elements;
/**
* @return the pageId
*/
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "PageID", nullable = false)
public Integer getPageId() {
return pageId;
}
/**
* @param pageId the pageId to set
*/
public void setPageId(Integer pageId) {
this.pageId = pageId;
}
/**
* @return the pageName
*/
@Column(name = "PageName", nullable = true, length = 256)
@Basic(optional = true)
public String getPageName() {
return pageName;
}
/**
* @param pageName the pageName to set
*/
public void setPageName(String pageName) {
this.pageName = pageName;
}
/**
* @return the pageNamePreview
*/
@Column(name = "PageNamePreview", nullable = true, length = 256)
@Basic(optional = true)
public String getPageNamePreview() {
return pageNamePreview;
}
/**
* @param pageNamePreview the pageNamePreview to set
*/
public void setPageNamePreview(String pageNamePreview) {
this.pageNamePreview = pageNamePreview;
}
/**
* @return the nav
*/
@ManyToOne(fetch = FetchType.LAZY, optional = false, cascade = CascadeType.ALL)
@JoinColumn(name = "NavID")
public Nav getNav() {
return nav;
}
/**
* @param nav the nav to set
*/
public void setNav(Nav nav) {
this.nav = nav;
}
/**
* @return the culture
*/
@ManyToOne(fetch = FetchType.LAZY, optional = false, cascade = CascadeType.ALL)
@JoinColumn(name = "CultureCode", referencedColumnName = "CultureCode")
public Culture getCulture() {
return culture;
}
/**
* @param culture the culture to set
*/
public void setCulture(Culture culture) {
this.culture = culture;
}
/**
* @return the pageStatus
*/
@Column(name = "PageStatus", nullable = false, length = 64)
@Basic(optional = false)
public String getPageStatus() {
return pageStatus;
}
/**
* @param pageStatus the pageStatus to set
*/
public void setPageStatus(String pageStatus) {
this.pageStatus = pageStatus;
}
/**
* @return the pageNavEnd
*/
@Column(name = "PageNavEnd", nullable = false)
@Basic(optional = false)
public Boolean getPageNavEnd() {
return pageNavEnd;
}
/**
* @param pageNavEnd the pageNavEnd to set
*/
public void setPageNavEnd(Boolean pageNavEnd) {
this.pageNavEnd = pageNavEnd;
}
/**
* @return the creationDate
*/
@Column(name = "CreationDate", nullable = false)
@Basic(optional = false)
public Date getCreationDate() {
return creationDate;
}
/**
* @param creationDate the creationDate to set
*/
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
/**
* @return the lastModified
*/
@Column(name = "LastModified", nullable = false)
@Basic(optional = false)
public Date getLastModified() {
return lastModified;
}
/**
* @param lastModified the lastModified to set
*/
public void setLastModified(Date lastModified) {
this.lastModified = lastModified;
}
/**
* @return the author
*/
@Column(name = "Author", nullable = false)
@Basic(optional = false)
public String getAuthor() {
return author;
}
/**
* @param author the author to set
*/
public void setAuthor(String author) {
this.author = author;
}
/**
* @return the pageVisibility
*/
@Column(name = "PageVisibility", nullable = false)
@Basic(optional = false)
public Boolean getPageVisibility() {
return pageVisibility;
}
/**
* @param pageVisibility the pageVisibility to set
*/
public void setPageVisibility(Boolean pageVisibility) {
this.pageVisibility = pageVisibility;
}
/**
* @return the pageVisibilityPreview
*/
@Column(name = "PageVisibilityPreview", nullable = false)
@Basic(optional = false)
public Boolean getPageVisibilityPreview() {
return pageVisibilityPreview;
}
/**
* @param pageVisibilityPreview the pageVisibilityPreview to set
*/
public void setPageVisibilityPreview(Boolean pageVisibilityPreview) {
this.pageVisibilityPreview = pageVisibilityPreview;
}
/**
* @return the approbationDate
*/
@Column(name = "ApprobationDate", nullable = true)
@Basic(optional = true)
public Date getApprobationDate() {
return approbationDate;
}
/**
* @param approbationDate the approbationDate to set
*/
public void setApprobationDate(Date approbationDate) {
this.approbationDate = approbationDate;
}
/**
* @return the elements
*/
@OneToMany(fetch = FetchType.LAZY, mappedBy = "page", cascade = CascadeType.ALL)
public Set getElements() {
return elements;
}
/**
* @param elements the elements to set
*/
public void setElements(Set elements) {
this.elements = elements;
}
/**
* Is this page visible
*
* @param isLive should the method return the value for live or preview
* @return true if the page is visible, false if it isn't
*/
@Transient
public boolean isVisible(boolean isLive) {
if (!"Approved".equals(pageStatus) && !"Pending".equals(pageStatus)) {
return false;
}
return isLive ? pageVisibility : pageVisibilityPreview;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy